View previous topic - View next topic |
Author |
Message |
LeoDraco Demon Hunter
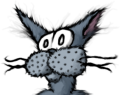
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Fri Oct 15, 2004 11:18 pm Post subject: |
[quote] |
|
LordGalbalan wrote: | It's an ALGORITHM for cryin' out loud! Its use depends on your definition of its components. It's an interaction between data and systems, at its most basic. |
Good algorithm design is intrinsically tied to data structure design; one can argue (and rather well) that implementations of algorithms that alter the internal representation of a data structure should be specifically tied to that data structure via some sort of encapsulation method. Extensibility, if required, can be provided without necessitating an understanding of the internal representation of the structure. Part of the problem with your implementations is that they are tied to a particular data structure internal representation that a client has to know about, rather than to an interface.
Quote: | Is it a waste of energy to rewrite an entire function just to state its variables in terms of a specific instance of it, instead of just passing objects or variables to it that are named in the context of their own use? Well yeah, it definitely is. |
I point you to this and ask you to apply the above quote to it. In the code found in that link, repetition of an entire function is found multitudinous times. Refactoring could simplify most of that.
I think that it would be more advantageous to construct the interfaces to operations common to vector spaces, and write the functionality that you are purporting to showcase utilizing that interface, rather than through the current methodolgy you are using. For example, we could say that a vector in its vector space is assignable, addable, and multiplicable. So, we could define the following:
Code: |
function assign( destination, source );
function add( source_a, source_b );
function multiply( source_a, source_b );
|
Where the latter two functions return the vector sum or product of the two source arguments. We could then write your function as:
Code: |
assign( destination, add( source, auxillary ) );
|
(Note, I really don't care for your terminology; I am simply using it for explicative purposes.)
Now, personally, I would use a language which provided operator overloading, and encode the implementations of these in overloaded methods of the objects. This would allow for the clean and sexy:
Code: |
destination := source + auxillary
|
What's more, you desire abstract extendibility, for code reuse, yes? We can define an abstract base class, called VectorSpace, in which are defined all operations applicable to a given vector space. (We could, theoretically, actually implement the operations in this class, if a common representation of the data could be found; however, as vectors are somewhat different from matrices are somewhat different from polynomials are somewhat different from functions, this isn't necessarily a good idea.) Then, for each particular vector space representation we desire, we could provide a subclass of VectorSpace, each of which would provide the implementation needed for the particular operation. Best part of all this? A client needs only know that he is operating on a given VectorSpace subclass, and not on the internal representation of that subclass.
If you still wanted to encode the "ScrollPlane" functionality with such a heirarchy, you could simply do the following:
Code: |
function ScrollPlane( destination: VectorSpace, source: VectorSpace, auxillary: VectorSpace )
{
destination := source + auxillary
}
|
You have your abstraction, which is perfectly extendible for all types of vector spaces, and encapsulation isn't broken. (Course, there is a slight problem with the above: you could throw any type of VectorSpace in there, which would be bad. Some run-time type-checking could be provided to ensure that everything is kosher.) _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sun Oct 24, 2004 9:28 pm Post subject: |
[quote] |
|
Well, it would appear that if I include the structure of the passed objects in my code, and provide an accompanying implementation of it, then my code will be understood. (at least, that's what I gather from LeoDraco)
I'm working to port these Javascript structures to C. Maybe that will secure a few less whines....
|
|
Back to top |
|
 |
Mandrake elementry school minded asshole

Joined: 28 May 2002 Posts: 1341 Location: GNARR!
|
Posted: Sun Oct 24, 2004 9:29 pm Post subject: |
[quote] |
|
Not if it still looks like ass. _________________ "Well, last time I flicked on a lighter, I'm pretty sure I didn't create a black hole."-
Xmark
http://pauljessup.com
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sun Oct 24, 2004 10:30 pm Post subject: |
[quote] |
|
Code: |
typedef struct PlanarAxis {
int Y;
int X;
};
typedef struct PLANE {
PlanarAxis Origin;
PlaxarAxis Total;
};
typedef struct SOURCE {
PLANE Plane;
};
typedef struct AUXILIARY {
PLANE Plane;
};
typedef struct DESTINATION {
PLANE Plane;
};
typedef struct FOCUS {
PLANE Sight;
PLANE Constant;
SOURCE Source;
PlanarAxis Index;
};
typedef struct MAP {
AUXILIARY Auxillary;
};
typedef struct VIEWPORT {
DESTINATION Destination;
};
ScrollPlane (Viewport, Map, Sprite);
void ScrollPlane (VIEWPORT *Viewport, MAP *Map, FOCUS *Focus) {
/* |*| The ScrollPlane Function
//
//
// |!| The ScrollPlane function translates a plane
// if its focus is centered.
*/
if (((Map->Origin->Y > Focus->Sight->Y)
&&
(Map->Origin->Y
< (Map->Total->Y - Focus->Sight->Y)))
&&
((Map->Origin->X > Focus->Sight->X)
&&
(Map->Origin->X
< (Map->Total->X - Focus->Sight->X)))) {
ScrollViewport (Viewport, Map, Focus);
}
else {
Focus->Index->Y = Focus->Index->Y + Focus->Constant->Y;
Focus->Index->X = Focus->Index->X + Focus->Constant->X;
Focus->Index = 1;
}
}
|
Better?
Last edited by tcaudilllg on Tue Oct 26, 2004 6:06 am; edited 6 times in total
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Sun Oct 24, 2004 10:53 pm Post subject: |
[quote] |
|
Actually in this case I'd say your JavaScript version was better. :-P
I still don't understand a single line of your code. And then there's also "Sprite->Constant->Y", where I don't see your sprite struct containing a "Constant" member.
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Mon Oct 25, 2004 12:40 am Post subject: |
[quote] |
|
LordGalbalan wrote: | Code: |
typedef struct PLANE {
int Y;
int X;
int Plane;
}; |
|
I have no idea what that is supposed to mean. In mathematics, a plane is a 2D space. That you need a datatype to represent a plane indicates to me that you want a plane as a 2D subset of a 3D space. The equation of a plane within a 3D space is ax + by + c = 0. In other words, I would expect a plane to have data members a, b, and c. I would expect these to be of some floating point type. I would expect these to be documented. I would not expect data members called X and Y. And why the hell would a datatype called PLANE have a member called Plane? Is that a recursive definition or something?
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Mon Oct 25, 2004 12:57 am Post subject: |
[quote] |
|
Removed the "Plane" integer because it was irrelevant to the function. Also added "Constant" to sprite as type PLANE.
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Mon Oct 25, 2004 2:12 am Post subject: |
[quote] |
|
I still have no idea what a PLANE object is supposed to represent. Unless (judging from its members) it's a point in a plane, in which case the names POINT, POINT2D, ORDERED_PAIR, COORDINATE, and XY would all have been more appropriate.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Mon Oct 25, 2004 2:30 am Post subject: |
[quote] |
|
It doesn't matter if you "know" what it means or not. You have a sense of its purpose by way of your own judgement, and that I gave you that sense of purpose was the entire intent.
This is the inequality that you need to scroll a viewport, and you understand that, too. What may not be clear is the focus....
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Mon Oct 25, 2004 3:21 am Post subject: |
[quote] |
|
Look, I am critiquing your code for your own benefit, since you obviously have trouble writing code that other people can understand. I know how to scroll a viewport, but your code reads like gibberish. If you choose to dismiss my critique, you are only hurting yourself and making it less likely that other people will help you.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Mon Oct 25, 2004 11:22 pm Post subject: |
[quote] |
|
I'm not dismissing what you say, only evaluating it in terms of my real goal. You have correctly discerned the use of this program. So, I have reached the "critical threshold" of your understanding.
EDIT: changed the Focus struct and reorganized the ScrollViewport function to reflect the changes. Should be consistent now.
Last edited by tcaudilllg on Tue Oct 26, 2004 6:08 am; edited 1 time in total
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Tue Oct 26, 2004 1:29 am Post subject: |
[quote] |
|
LordGalbalan wrote: | You have correctly discerned the use of this program. |
Only because you told me. Although I have to admit that the current code is much better than the original.
|
|
Back to top |
|
 |
Rooter Copyright Infringer

Joined: 09 Feb 2003 Posts: 61
|
Posted: Tue Oct 26, 2004 3:29 am Post subject: |
[quote] |
|
Mandrake is a dickhead. :)
|
|
Back to top |
|
 |
Terry Spectral Form
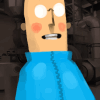
Joined: 16 Jun 2002 Posts: 798 Location: Dublin, Ireland
|
Posted: Tue Oct 26, 2004 5:34 am Post subject: |
[quote] |
|
Quote: | Mandrake is a dickhead. :) |
What the hell is wrong with you? Do you just stalk the threads here waiting for opertunitities to tell mandrake off?
Get a life!
(edit) p.s. before you start, I'm not nessicarly trying to stand up for mandrake - I just think you're being a prick. _________________ http://www.distractionware.com
|
|
Back to top |
|
 |
Rooter Copyright Infringer

Joined: 09 Feb 2003 Posts: 61
|
Posted: Tue Oct 26, 2004 11:18 pm Post subject: |
[quote] |
|
Chaotic Harmony wrote: | Quote: | Mandrake is a dickhead. :) |
What the hell is wrong with you? Do you just stalk the threads here waiting for opertunitities to tell mandrake off?
Get a life!
(edit) p.s. before you start, I'm not nessicarly trying to stand up for mandrake - I just think you're being a prick. |
And to think I gave you 1st in the contest!
BTW, I want to rip you limb from limb Mandrake!
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|