View previous topic - View next topic |
Author |
Message |
Mandrake elementry school minded asshole

Joined: 28 May 2002 Posts: 1341 Location: GNARR!
|
Posted: Sat Aug 27, 2005 3:02 am Post subject: |
[quote] |
|
SDL is much better for programming on Linux. It works right out of the box, and no need to worry about dependencies cause every single Linux distro has it.
And here's an excellent article on makefiles:
http://www.elitecoders.de/mags/cscene/CS2/CS2-10.html
Sheesh. I never had anyone tell me this stuff. Had to all find it out myself. Kids these days, I tell ya, _________________ "Well, last time I flicked on a lighter, I'm pretty sure I didn't create a black hole."-
Xmark
http://pauljessup.com
|
|
Back to top |
|
 |
biggerUniverse Mage
Joined: 18 Nov 2003 Posts: 326 Location: A small, b/g planet in the unfashionable arm of the galaxy
|
Posted: Sat Aug 27, 2005 3:12 am Post subject: |
[quote] |
|
Mandrake wrote: | Sheesh. I never had anyone tell me this stuff. Had to all find it out myself. Kids these days, I tell ya, |
Amen to that. When I started in Linux, the mascot was an egg! If you wanted network, you had to scrounge around for a DEC-based NIC, so the tulip driver would see it. We called it X10R1! The boot spelled out LILO, then finished in 30 seconds! There was no USB. I could fit my distro and all my stuff on a 2Gb drive, and it was big, loud, and slow. Kids these days! "Where's my fufu little laptop that's silent and only weighs 10 ounces and has auto-negotiating DHCP wireless AND a 40Gb HDD?" Weenies! In my day, the email clients were named after trees and dogs! If you surfed the web, you did it with your minicom 0.X and a z-modem!
Anyway. Some of that might possibly be almost true.
EDIT: yeah, ya did have to scrounge. My card of choice was the SMC 9332. Solid. Ah, those were the days... _________________ We are on the outer reaches of someone else's universe.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
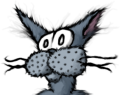
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sat Aug 27, 2005 5:07 am Post subject: |
[quote] |
|
DeveloperX wrote: | I figured out how to use a single makefile for multiple projects...but
how do you use multiple source files for a single project?
I've never compiled a multi-source program on the commandline.
Like, say I have 2 source files and a header file:
main.c
functionlib.c
functionlib.h
how would I write the makefile for building it?
I think that its something like
gcc -c main.c functionlib.c main.o functionlib.o -o program
..help.. :D |
This is the exact reason why make exists: eliminating the necessity of having to think about these types of problems.
The most naive approach would be the following:
Code: | all: program
program: main.o functionlib.o
gcc -o program main.o functionlib.o
main.o: main.c functionlib.h
gcc -c main.c -o main.o
functionlib.o: functionlib.c functionlib.h
gcc -c functionlib.c -o functionlib.o |
However! That would happen to be the worst type of makefile one could write. Way too much information. The make grammar provides a whole slew of opportunities to refactor it down. (While the following will be as explicative as I can possibly make it, you should definitely read through the info-pages for make, as well as the info-pages for gcc.)
So! First, you will notice that the make definition for compiling the two object files is exactly the same, save for the file names. Make recognizes a wildcard character, %:
Code: | %.o: %.c functionlib.h
gcc -c $< -o $@ |
All variables in make are prefixed with a dollar-sign; the general practice is to refer to all variables as the following:
with the exception of the predefined variables (of which $< and $@ are two). $< refernces the first file dependency in the dependecy list. (If you don't know (exactly) what dependency lists are, they are that bit right after the colon:
Code: | target: dep1 [dep2 ...] |
Make functions off of the dependencies; namely, it checks the last modification time of each file in the dependency list (making those files, should they need to be).) $@ references the target of the make definition.
The second thing you should note is that make does not require all of the dependencies for a file to be listed in a single definition, and that multiple targets may be specified at once. So, we can rewrite the above as the following:
Code: | main.o functionlib.o: functionlib.h
%.o: %.c
gcc -c $< -o $@ |
While the benefit of this is not immediately apparent, suffice to say that it is good for either of two situations: first, when you are manually specifying dependencies, you can cut down some space by grouping together files that share dependencies; second, when relying upon auto-dependency generation (which gcc provides), you only have to worry about the rule to compile a generic source file and not about any silly dependencies.
Now, I mentioned make variables above; it is good form to bind all (relevent) commands and command flags to variables. For instance, let's say the compiler you are using is "gcc", you want debugging info compiled into the binary so that you can trace through gdb (-g), and you want all warning messages turned on (-Wall); at the beginning of your makefile you would do the following:
Code: | CC=gcc
CFLAGS=-g -Wall |
you would then replace all references in the makefile to "gcc" with "$(CC)", and on all lines that invoked the compiler, add "$(CFLAGS)". According to the GNU info pages, it is good form to place the latter towards the end of the command.
(The reason behind this abstraction is (possibly) threefold: first, it allows a given user whom is compiling your project with make to specify overriding values for the make variables --- it is a matter of courtesy; second, it can potentially cut down on the amount of redundant text found throughout the makefile, which would obviously cut out the amount of text you have to write; third, following the KISS principle, you only have to alter project-wide options in a single place, rather than all over the place.)
Let us look at the "simplified" makefile:
Code: | CC=gcc
CFLAGS=-g -Wall
# .PHONY informs make that the targets in the dependency list do
# not build anything; this is not strictly needed, but it can resolve
# some issues...
.PHONY: all clean
all: program
program: main.o functionlib.o
$(CC) -o $@ main.o functionlib.o $(CFLAGS)
# Note! unlike the above, this will cause each built object to
# depend upon functionlib.h, which may not be entirely truthful.
%.o: functionlib.h
%.o: %.c
$(CC) -c $< -o $@ $(CFLAGS)
# It is customary to include a command that removes all generated
# files; this is referred to as "cleaning", and is generally bound to
# the "clean" target. I usually also have this remove file backups
# (which can be dangerous!)
clean:
rm -f *.o program |
In my personal opinion, a "good" makefile would be one that requires as little maintenance as possible. The above makefile does not fit that measure, and for good reason: first, their is needless duplication of "main.o functionlib.o" in the rule to generate "program"; this can be resolved by adding another variable:
Code: | OBJS=main.o functionlib.o |
and replacing the obvious references; second, the makefile needs updating everytime a new source/header file is added to the project, as well as whenever a given file changes its dependencies. All of this is tedium that you should not have to do. Enter automatic dependency resolution, where make is responsible for determining when it needs to update the makefile. While several options exist for doing this, my favorite way is to use gcc to generate them for you.
edit: that $(OBJS) variable is not very kosher with my standard, and should not be interpreted as a good method to go about doing that type of thing. Make provides a wildcard function for obtaining files that match a pattern in a given directory. You can then use the string substitution functions to shape the automatically obtained file listings into whatever form you need.
edit2: D'oh! Noticed Mandrake's link after the fact. _________________ "...LeoDraco is a pompus git..." -- Mandrake
Last edited by LeoDraco on Sat Aug 27, 2005 5:35 am; edited 1 time in total
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
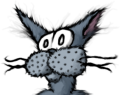
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sat Aug 27, 2005 5:27 am Post subject: |
[quote] |
|
biggerUniverse wrote: | ...In my day, the email clients were named after trees and dogs!... Anyway. Some of that might possibly be almost true. |
I still use "pine" from time to time, if it is any consolation. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
DeveloperX 202192397
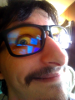
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Aug 27, 2005 7:47 am Post subject: |
[quote] |
|
Yowza!
Well, here is my current Makefile:
Code: |
#************************************************************
#
# Make file for SDL lesson projects
# type "make all" to build all projects
#
#************************************************************
all: lesson1 lesson2 lesson3
#************************************************************
lesson1: lesson1.o
gcc -g `sdl-config --libs` lesson1.o -o lesson1
lesson1.o: lesson1.c
gcc -g -c `sdl-config --cflags` lesson1.c
#************************************************************
lesson2: lesson2.o
gcc -g `sdl-config --libs` lesson2.o -o lesson2
lesson2.o: lesson2.c
gcc -g -c `sdl-config --cflags` lesson2.c
#************************************************************
lesson3: lesson3.o
gcc -g `sdl-config --libs` lesson3.o -o lesson3
lesson3.o: lesson3.c
gcc -g -c `sdl-config --cflags` lesson3.c
#************************************************************
clean1:
rm -f lesson1.o lesson1
clean2:
rm -f lesson2.o lesson2
clean3:
rm -f lesson3.o lesson3
cleanall:
rm -f *.o lesson1 lesson2 lesson3
|
I'll need to read over your posts a couple times before I can fully comprehend what I need to alter to make my Makefile more 'acceptable'
I really appreciate the help from all of you guys.
I've thought about it, and I'm thinking of sticking with SDL, a fresh start, a new direction, a new dream..well, same dream.
Perhaps Dream Destroyer will finally see the light.....or the darkness, as the story has it. ;)
Only time will tell, and I've got much to learn, and much to do.
Oh, before I forget, LUA...is it available on most distros, and is it easy to work with for scripting?
I may use Tcl or a generic self-rolled command-based scripting setup..what do you think would be best?
Thanks. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Mandrake elementry school minded asshole

Joined: 28 May 2002 Posts: 1341 Location: GNARR!
|
Posted: Sat Aug 27, 2005 1:27 pm Post subject: |
[quote] |
|
I'm not sure if it's available on mst distro's out of the box, but unlike Allegro most have a version you can yum/apt-get/whatever so it's a bit more acceptable to the common Linux user.
Yes, it's insanely simple and hella powerful at the same time. Kind of like RegExp in that way. _________________ "Well, last time I flicked on a lighter, I'm pretty sure I didn't create a black hole."-
Xmark
http://pauljessup.com
|
|
Back to top |
|
 |
 |
Page 2 of 2 |
All times are GMT Goto page Previous 1, 2
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|