View previous topic - View next topic |
Author |
Message |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Thu Sep 01, 2005 12:19 am Post subject: |
[quote] |
|
LeoDraco wrote: | This is where storing data in a database would be (moderately) useful. Then you need not worry about field boundaries at all! The DB does it for you! Hurray databases!
Alternately, as you seem to want these to be flat-files that anyone can edit, XML would probably be the better route:
Code: | <?xml version="1.0"?>
<icon>
<name>Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit...</name>
<image>noloveforpain.gif</image>
<text>noloveforpain.txt</text>
</icon> |
There! Perfectly capable of being externalized! Hurray externalizability! |
What about this?
Code: |
<icon>
<name>text tag closure</name>
<image>texttagclosure.gif</image>
<text></text></text>
</icon>
|
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
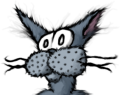
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Thu Sep 01, 2005 12:27 am Post subject: |
[quote] |
|
LordGalbalan wrote: | Code: |
<icon>
<name>text tag closure</name>
<image>texttagclosure.gif</image>
<text></text></text>
</icon>
|
|
That would be what entities would be for. I.e. the text element would become (using HTML character entities):
Code: | <text></text></text> |
Not too hard! _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Thu Sep 01, 2005 12:28 am Post subject: |
[quote] |
|
I've been considering using entities. But what if the icon text itself is the entity?
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
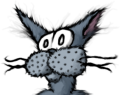
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Thu Sep 01, 2005 12:33 am Post subject: |
[quote] |
|
I'm not sure what you are getting at. You mean you want some sort of meta-entity? While that should not be needed, you could always do:
Code: | <text>&lt;</text> |
_________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Thu Sep 01, 2005 12:35 am Post subject: |
[quote] |
|
LordGalbalan wrote: | I've been considering using entities. But what if the icon text itself is the entity? |
I'm not sure I follow this, but you do realise entities can stack up?
</text>
&lt;/text&gt;
&amp;lt;/text&amp;gt;
&ampamp;lt;/text&ampamp;gt;
etc..
Where's the problem? I don't think I understood the question; if that's the case, do you have an example where problems would occure?
Edit: Beaten by LeoDraco. For now. ;) _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
Last edited by RuneLancer on Thu Sep 01, 2005 12:35 am; edited 1 time in total
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Thu Sep 01, 2005 12:35 am Post subject: |
[quote] |
|
But how do I code that? It would be possible to have meta meta meta meta meta meta meta entities!
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
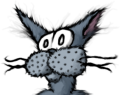
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Thu Sep 01, 2005 1:11 am Post subject: |
[quote] |
|
Given your proclivity towards JavaScript, I am going to assume that you are implementing this fascinating project with it. Taking that as given, you could probably just parse the XML tree with the DOM functions.
Now, about the character entity things: again, for what use do you forsee even needing the whole meta-entity thing? The only current reason why one would need that is for situations where entities show up that you do not want processed, and, even then, it usually only has a single level of escaping. (E.g. escaping ampersands in url's sent to a server, or escaping entities that show up in a form.) One solution would be to write some sort of parser to handle them. The better solution is to make use of JavaScript's regular expression engine to parse the entities. I.e.:
Escaping:
Code: | var re = /\&[^\;]*\;/i;
var str = "&";
var escaped = str.replace( re, "&" ); // escaped == "&amp;" |
Stripping:
Code: | var re = /\&[^\;]*\;/i;
var str = "&"
var stripped = str.replace( re, "&" ); // stripped = "&"
|
Or something like that. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Nephilim Mage

Joined: 20 Jun 2002 Posts: 414
|
Posted: Thu Sep 01, 2005 4:24 am Post subject: |
[quote] |
|
LordGalbalan wrote: | RuneLancer wrote: | Or, you know, you could just create an "icon" class instead of coming up with some convoluted means of packing everything together.. |
Stop acting like I'm an idiot. Why not tell yourself that I'm *gasp* every bit as intelligent as you and probably thought things through? Then you'd note that I'm talking about a file format, not a programming construct. |
You know, with statements like that, you just set yourself up to be shown to be *gasp* less intelligent than other people. RuneLancer's response was perfectly appropriate for your dilemma - once you have it as a class, just serialize the class, and you have a string you can store in a file. You don't have to worry about all the file formatting stuff you're wringing your hands over.
That said, although they'll probably require more work, LeoDraco's suggestions of a database or XML storage are probably better than storing a text file that is serialized in the manner in which, say, PHP serializes objects. Database implementations will handle all the twiddly bits you probably don't want to mess with, while the XML storage is human-readable and pioneers have solved all the problems (like storing, parsing, and the entity problems you're bringing up) long before you even came on the scene.
I know you fancy yourself as a trailblazer, but these trails have already been blazed, dude. Spend your efforts on the creative issues of your application, not reinventing the wheel. _________________ Visit the Sacraments web site to play the game and read articles about its development.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Thu Sep 01, 2005 8:48 pm Post subject: |
[quote] |
|
The only solution is to code the length of the string before the start of the string.
I want icons to be capable of binary text too, that is, text that is essentially random, binary code. Like image data, or compressed data. So it is impossible to differentiate between escape characters and valid data, because the escape characters can emerge.
Basically, this is a case of Godel's incompleteness theorem: if the one system's data system is another system's energy system, then it is impossible for the two systems to communicate, because the confusion is endless.
Unless... an auxiliary system converted energy to data and data to energy between the two. But I can't do that now.
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Thu Sep 01, 2005 9:03 pm Post subject: |
[quote] |
|
1- There are many ways to encode a variable-length string without resorting to storing the length. While not a bad solution, it means you have to constantly keep it updated. Null-terminated strings don't require such maintenance. On the flipside, you can't know the exact length until you've parsed the string. Keeping the length is definately NOT the only solution, but wether it's the best or not depends heavily on the situation.
2- There's absolutely no situation where escaping characters will cause any problems. The only reason why such a system would fail is because of a horrible parser that ignores escaped characters at random! If, for instance, a backslash escapes a character (including a backslash itself, so two backslashes would translate to a single, normal backslash), short of using a different parser that treats backslashes differently, you will never find yourself in a situation where there'd be confusion between escaped and unescaped characters. Try it.
3- There is no number 3. If there were, however, it would probably involve pie and cookies. Instead, though, I think I'd rather make it on how computer science concepts and physics concepts are not the same and don't carry over to one another. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Thu Sep 01, 2005 9:08 pm Post subject: |
[quote] |
|
LeoDraco is "a" number 3, ...
Um, hmm... I never thought about the escape thing being a problem before....
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
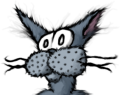
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Thu Sep 01, 2005 9:43 pm Post subject: |
[quote] |
|
LordGalbalan wrote: | I want icons to be capable of binary text too, that is, text that is essentially random, binary code. Like image data, or compressed data. So it is impossible to differentiate between escape characters and valid data, because the escape characters can emerge. |
This is where base64 encoding/decoding comes in: rather than output the raw binary data, you base64 encode it; the encoding used for MIME contains a minimal set of characters, neither of which maps to ampersand nor to semi-colon. When you want to access the raw data, simply decode it. Not too difficult!
Note! The only way in which this method might be considered flawed is if space is a concern; i.e. the encoding generally increases the size of the input data. While this is usually not a problem, you can also look into various compression algorithms to try to decrease the size of the binary data (which may or may not be possible, depending upon what the data is).
Unless they have changed their file format, Bjorn/biggerUniverse's Tiled contains binary data in the XML that has been base64 encoded. Works, and, best of all, maintains XML's human-readability. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Thu Sep 01, 2005 10:28 pm Post subject: |
[quote] |
|
I think I'd rather go with the length tracker, given the circumstances.
Base64... c^2, where 8 equals C?
Question: would c^3 be enough to create a generally relativistic environment whose maximum translation rate was c?
|
|
Back to top |
|
 |
 |
Page 2 of 2 |
All times are GMT Goto page Previous 1, 2
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|