View previous topic - View next topic |
Author |
Message |
DeveloperX 202192397
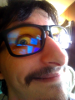
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Sep 27, 2005 8:45 am Post subject: peeking and poking around |
[quote] |
|
Okay, I know how to do this shit in basic, now, someone instruct me on how to recode this in C:
Code: |
REM * DUMP 512 BYTES OF MEMORY FROM 0xD000
C = 0
DEF SEG = &HD000
FOR N = 0 TO 511
PRINT HEX$(PEEK(N));
IF C < 15 THEN C = C + 1 ELSE C = 0 : PRINT
NEXT
DEF SEG
REM WRITE ZEROS TO 512 BYTES OF MEMORY STARTING AT 0xD000
DEF SEG = &HD000
FOR N = 0 TO 511
POKE N, 0
NEXT
DEF SEG
|
please give me a hand.
thanks.
..yeah, yeah, I know...I probably _should_ know this already...
but damnit, I cant seem to wrap my head around what to do, and I searched google and came up with ziltch.
Perhaps I'm looking up the wrong stuff, I dunno..anyway,
please help.
:) _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
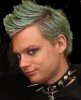
Joined: 29 May 2002 Posts: 559
|
Posted: Tue Sep 27, 2005 9:57 am Post subject: |
[quote] |
|
think "pointer" ... _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
DeveloperX 202192397
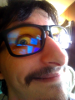
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Sep 27, 2005 2:49 pm Post subject: |
[quote] |
|
DrunkenCoder wrote: | think "pointer" ... |
I figured as much..but I cant seem to get the code right.
cmon, someone please just explain what I need to do to recode that QB code in C. Thanks. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Mokona Pretty, Pretty Fairy Princess
Joined: 26 Jun 2003 Posts: 12
|
Posted: Tue Sep 27, 2005 4:36 pm Post subject: |
[quote] |
|
Straight conversion:
Code: | // DUMP 512 BYTES OF MEMORY FROM 0xD000
unsigned char * n = (unsigned char*) 0xD000;
for (int i=0; i<512; i++)
printf("%02x\n", n[i]);
// WRITE ZEROS TO 512 BYTES OF MEMORY STARTING AT 0xD000
unsigned char * n = (unsigned char*) 0xD000;
for (int i=0; i<512; i++)
n[i] = 0;
|
Or you could use
Code: |
// WRITE ZEROS TO 512 BYTES OF MEMORY STARTING AT 0xD000
unsigned char * n = (unsigned char*) 0xD000;
memset(n, 0, 512);
|
Note: I haven't actually tested this code, but hopefully you get the idea.
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
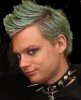
Joined: 29 May 2002 Posts: 559
|
Posted: Tue Sep 27, 2005 4:38 pm Post subject: |
[quote] |
|
your milage may vary but this should be darn close.
Code: |
//dump
int c = 0;
unsigned char *p = 0xD000
for(int n = 0; n != 512; ++n)
{
printf( "%.2x ", p[n]);
if( ++c == 16)
{
printf("\n")
c = 0;
}
}
//set
memset( 0xD000, 0, 512)
|
_________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
DeveloperX 202192397
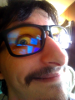
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Sep 27, 2005 7:54 pm Post subject: |
[quote] |
|
DrunkenCoder wrote: | your milage may vary but this should be darn close.
Code: |
//dump
int c = 0;
unsigned char *p = 0xD000
for(int n = 0; n != 512; ++n)
{
printf( "%.2x ", p[n]);
if( ++c == 16)
{
printf("\n")
c = 0;
}
}
//set
memset( 0xD000, 0, 512)
|
|
segment fault violation error shit.
:\ _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Tue Sep 27, 2005 8:11 pm Post subject: |
[quote] |
|
That code can't work: 0xD000 can point to anything. It's definately not going to behave the same way as you'd expect it to behave in QBasic. You can't normally access memory outside of what your program's addressing space or it'll cause problems. That wasn't the case with DOS, but chances are you're coding this in Windows or under console mode, which doesn't quite behave the same way as DOS did.
What's supposed to be located at 0xD000? I THINK it's the graphic buffer, but that might be 0xB000? It's been quite a while, heh...
For the sake of clarity, though, what are you trying to do? If it's just accessing RAM directly, that isn't too hard so long as you reserve it first (malloc in C, new in C++). But if you're trying to write into hardware addresses the way you could in QBasic, I'm not sure how... _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Tue Sep 27, 2005 8:20 pm Post subject: Re: peeking and poking around |
[quote] |
|
DeveloperX wrote: | Okay, I know how to do this shit in basic, now, someone instruct me on how to recode this in C:
Code: |
REM * DUMP 512 BYTES OF MEMORY FROM 0xD000
C = 0
DEF SEG = &HD000
FOR N = 0 TO 511
PRINT HEX$(PEEK(N));
IF C < 15 THEN C = C + 1 ELSE C = 0 : PRINT
NEXT
DEF SEG
|
In C:
Code: |
int C = 0;
/* declare a far pointer, to access the full memory spectrum*/
char far *Segment = 0xd0000000h;
/* you know what this does */
for (n = 0; n < 512; n++) {
/* increment the offset */
Segment = Segment + n;
/* not sure about this part... maybe d% instead */
/* nevermind I looked it up: %x is the c format spec for hex */
/* When working with pointers, the asterisk refers to the data pointed to. */
printf("%x\n", *Segment);
/* again you know this */
if (C < 15) { C++; } else { C = 0; }
}
*
|
Code: |
REM WRITE ZEROS TO 512 BYTES OF MEMORY STARTING AT 0xD000
DEF SEG = &HD000
FOR N = 0 TO 511
POKE N, 0
NEXT
DEF SEG
|
In C:
Code: |
char far *Segment = 0xd0000000h;
for (n = 0; n < 512; n++) {
Segment = Segment + n;
*Segment = 0;
}
|
please give me a hand.
thanks.
..yeah, yeah, I know...I probably _should_ know this already...
but damnit, I cant seem to wrap my head around what to do, and I searched google and came up with ziltch.
Perhaps I'm looking up the wrong stuff, I dunno..anyway,
please help.
:) |
Last edited by tcaudilllg on Tue Sep 27, 2005 8:50 pm; edited 1 time in total
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Tue Sep 27, 2005 8:46 pm Post subject: |
[quote] |
|
There's a difference between using the address in question to acheive an effect in hardware (the graphic buffer comes to mind) and simply writing directly to RAM (which is what most people have been explaining.) _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Tue Sep 27, 2005 8:52 pm Post subject: |
[quote] |
|
No, there isn't. Hardware memory is the same as RAM. You know you can't have 4gigs of straight RAM on a 32-bit pc, don't you? :)
What do you think malloc is in C code? A loop that sets all offset addresses in an arbitrary range to 0, but at addresses that are chosen by C.
I think what you're thinking about, RuneLancer, is that you need near pointers to address the first 64k, and far pointers to address the rest. Although in the 32bit world you don't need to address pointers as "far", am I right?
I've never done 32bit programming, so I don't know. I'd still address them as far anyway, just to be sure. :)
|
|
Back to top |
|
 |
DrV Wandering Minstrel

Joined: 15 Apr 2003 Posts: 148 Location: Midwest US
|
Posted: Tue Sep 27, 2005 9:36 pm Post subject: |
[quote] |
|
LordGalbalan wrote: | No, there isn't. Hardware memory is the same as RAM. | Not really; sure, it's mapped into the same memory space sometimes, but not necessarily.
LordGalbalan wrote: | What do you think malloc is in C code? A loop that sets all offset addresses in an arbitrary range to 0, but at addresses that are chosen by C. |
Actually, malloc() does not touch the memory it allocates, and it usually calls an underlying OS memory allocator... calloc() does set the allocated memory to 0, though.
LordGalbalan wrote: | I think what you're thinking about, RuneLancer, is that you need near pointers to address the first 64k, and far pointers to address the rest. Although in the 32bit world you don't need to address pointers as "far", am I right? |
On the x86, near pointers can be either 16-bit or 32-bit, and far pointers are either 32 or 48 bits. 'Near' means 'without a segment/selector override'. _________________ Don't ask no stupid questions and I won't send you away.
If you want to talk fishing, well, I guess that'll be okay.
|
|
Back to top |
|
 |
janus Mage
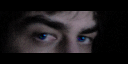
Joined: 29 Jun 2002 Posts: 464 Location: Issaquah, WA
|
Posted: Tue Sep 27, 2005 10:48 pm Post subject: |
[quote] |
|
LordGalbalan wrote: | No, there isn't. Hardware memory is the same as RAM. You know you can't have 4gigs of straight RAM on a 32-bit pc, don't you? :)
What do you think malloc is in C code? A loop that sets all offset addresses in an arbitrary range to 0, but at addresses that are chosen by C.
I think what you're thinking about, RuneLancer, is that you need near pointers to address the first 64k, and far pointers to address the rest. Although in the 32bit world you don't need to address pointers as "far", am I right?
I've never done 32bit programming, so I don't know. I'd still address them as far anyway, just to be sure. :) | Do you have any idea what you're talking about? Malloc doesn't erase allocated memory. It allocates a block of memory off the heap (or the free store; I forget which, and it probably depends on your runtime library) and returns a pointer to the beginning of that block. It doesn't have ANYTHING to do with erasing memory.
Also, yes, you can have 4GB of RAM on a 32-bit system. In fact, you can have more - Intel and other processor manufacturers provide what is called a 'Physical Address Extension' or PAE that allows the OS to manage more than 4GB of total memory. The 2gb/4gb per process address space restriction still applies, though, if memory serves, but many large scale server apps use multiple worker processes anyway.
Additionally, it is possible to have an arbitrary amount of video memory on your graphics card and then map portions of that into your address space. This is traditionally done for direct screen reads/writes with the framebuffer, and also done to upload/download texture contents.
As far as this near/far pointer crap goes, there's really no excuse to write 16-bit code anymore for desktop systems; and there's absolutely no excuse for using QBASIC. :P And unfortunately, you can't just port QB code that uses peek/poke to C, because of the drastic differences between the two. If you really want to port that code, explain what it DOES and then maybe people will be able to help you. You're getting a segment fault because the code's purpose is invalid.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Tue Sep 27, 2005 11:01 pm Post subject: |
[quote] |
|
Well I'm sure I successfully ported that code... anyway, no way you're gonna see more than 4GB of RAM on a 32-bit off-the-shelf PC, although the point could be made also that you probably aren't gonna buy a 4GB RAM PC right now anyhow....
|
|
Back to top |
|
 |
DeveloperX 202192397
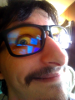
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Sep 27, 2005 11:27 pm Post subject: |
[quote] |
|
LordGalbalan wrote: | Well I'm sure I successfully ported that code... |
Sorry Tony, you are wrong as well.
All I get are segmentation fault access violation errors.
isnt there someone here that can do this?
what I am trying to do is store any misc data that I want in a 'free space' and read it back, as well as clean it up afterwards.
0xd000 has 512+ bytes of 'free space' where I am storing my data, and I want to write a memory dump utility. (I've written one in qb using the code that I showed above...now I want to rewrite it in C.)
It HAS to be possible, because there are so many programs out there that do such things.
I just want to learn HOW.
this has nothing to do with hardware registers or anything.
I'm not trying to write pixels, nor access hardware devices. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Tue Sep 27, 2005 11:59 pm Post subject: |
[quote] |
|
Man I don't know what you are doing, but that is solid code... maybe Windows doesn't think that is "free space".
|
|
Back to top |
|
 |
 |
Page 1 of 2 |
All times are GMT Goto page 1, 2 Next
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|