View previous topic - View next topic |
Author |
Message |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Fri Feb 10, 2006 12:07 am Post subject: |
[quote] |
|
Yep. Just create a rectangular region encompassing the area you want to display and blit it. All's you have to do to scroll is move the rectangle's left and top coordinates. I believe many blitter functions can take a rectangle struct for their coordinates, too. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Fri Feb 10, 2006 12:19 am Post subject: |
[quote] |
|
Ok, so I junked the map scrolling part that I was working on. Basically I wanted to see if I could do it, and since I proved myself right, I started designing a map class (before I just threw it in with other functions)
However, I'm getting exceptions now and the program is compiling but stopping during execution (the first two seconds) and nothing is being drawn
When I open the debugger and try to step through the program, everything is fine until I hit my:
SDL_BlitSurface(bitmap, &source, m_screen, &destination);
function. It gives me the following exception:
Unhandled exception at 0x100226d0 in Default Project.exe: 0xC0000005: Access violation reading location 0xabababd7.
Now I don't know what the deal is, because I haven't touched that function whatsoever during the entire map class addition, and it was working fine before.
does anyone know what this means? It's driving me nuts.
If you want to look at some code, check it out here
I didn't even load the bitmap or even initialize a map class for that matter. I was trying to make sure everything worked with just blitting the character, which it didn't, so I haven't even drawn the map yet.
And it's pretty self-explanitory as to what class does what. I tried to let each class be able to handle itself fully (drawing, moving, etc.), and the part where I'm getting the error is Under the "Graphics.h" file at the method "DrawBitmap".
I'll be back in a few hours.
Jason
|
|
Back to top |
|
 |
zenogais Slightly Deformed Faerie Princess
Joined: 10 Jan 2006 Posts: 34
|
Posted: Fri Feb 10, 2006 2:02 am Post subject: |
[quote] |
|
Seems to me you are using Visual C++, and as usual the errors in the debugger aren't very helpful, unless you've seen them before. From experience I can tell you that the message you are receiving probably indicates that you are passing in a bad (invalid because you deleted or never instantiated the object) pointer to SDL_BlitSurface somewhere. Check to make sure that all images loaded properly, another way to catch this error is to set all pointers to NULL or 0 on initialization and after deletion, then use something like:
Code: |
assert(bitmap != NULL && "bitmap pointer is invalid!");
|
Or if you want something more c++y:
Code: |
if ( !bitmap ) {
throw std::runtime_error("bitmap pointer is invalid!")
}
...
try {
m_graphics->DrawBitmap(bitmap, x, y);
} catch ( std::runtime_error& error ) {
cout << "Exception Caught!" << endl << error.what( ) << endl;
}
|
This would probably be the fastest way to track this error down, besides the usual commenting out lines in order to do process of elimination as to where the error originates from.
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Fri Feb 10, 2006 2:58 am Post subject: |
[quote] |
|
What I do to handle pointers is declare them as null.
SomeType *MyVar = NULL;
I can check its existence easily enough then.
if(MyVar) { ... }
Then when I need to destroy it (possibly in the class deconstructor or during the WM_QUIT message) I just check if it exists then set it to null again when done.
if(MyVar) { delete [] MyVar; MyVar = NULL; } _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Fri Feb 10, 2006 3:15 am Post subject: |
[quote] |
|
Better not to use raw pointers at all. std::auto_ptr, boost::scoped_ptr, and boost::shared_ptr are your best friends. Even if these smart pointer classes do not meet your needs, you should always use the Resource Acquisition Is Initialization (RAII) idiom for resource management in C++.
|
|
Back to top |
|
 |
zenogais Slightly Deformed Faerie Princess
Joined: 10 Jan 2006 Posts: 34
|
Posted: Fri Feb 10, 2006 3:20 am Post subject: |
[quote] |
|
Reiner Deyke: I personally use boost::shared_ptr almost exclusively as std::auto_ptr has its own share of problems which boost::shared_ptr addresses nicely.
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Fri Feb 10, 2006 3:59 am Post subject: |
[quote] |
|
Ok...?
Now it works fine. Lol, if only that could solve my problems. Wait three hours and try it again.
Voila
Jason
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Fri Feb 10, 2006 4:49 am Post subject: |
[quote] |
|
Do you guys ever leak memory, like at a rapid rate?
for some reason, whenever I'm in the demo (fullscreen) no memory is lost, but the minute I minimize that screen and look at the task manager it goes up at 40 MB per second.
Any ideas on what causes that?
Thanks,
Jason
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Fri Feb 10, 2006 5:34 am Post subject: |
[quote] |
|
Gardon wrote: | Do you guys ever leak memory, like at a rapid rate? |
Not since I started using boost::shared_ptr and boost::scoped_ptr.
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Fri Feb 10, 2006 5:37 am Post subject: |
[quote] |
|
If you have a memory leak, something's wrong. It's inacceptable for an app, no matter what the circumstances, to do this.
The reason for a memory leak to occure is because, in short, you're creating something in memory but never destroying it. The memory remains reserved and Windows cannot use it. What's worse, because nothing points to that memory anymore, it will never be released (until you reboot, that is.)
If it's created, it has to be destroyed. Always make sure of that. If it's created again, the old one has to be destroyed beforehand, too.
Various libraries can help you out with pointers if you're unable to figure out what's causing the problem... though it wouldn't hurt figuring out just why you have a leak in the first place even if you let something manage your pointers for you. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Fri Feb 10, 2006 6:37 am Post subject: |
[quote] |
|
It's weird because once I quit the program all the memory frees up (so I guess it's not a memory leak).
The only thing I can think of is if it's repetitively loading something. However it isn't so I'm unsure of the problem. Whatever, I'll find it tomorrow.
I did have one other question though. I'm trying to implement scrolling between maps without stopping. I've tried a 3 x 3 box of maps that I can load and unload safely (so it won't stop movement), but I have to use for loops or some really crazy if statements to check if the boundaries are being exceeded.
I'd rather not have a for loop each frame trying to decide whether or not the boundary was crossed.
So what's the best way to do it? Or is there a more efficient way to check if the boundaries were crossed?
Jason
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Fri Feb 10, 2006 7:05 am Post subject: |
[quote] |
|
I believe SDL may be smart enough to know what memory it ends up reserving for surfaces and the likes, and frees it up once the application terminates. Quite frankly, I've made it a habit to null my pointers before and after use and to check them before reserving memory, so I'm not sure what the behavior is anymore. ^^; I'm pretty sure I remember hearing that WinXP is smarter about memory and can associated reserved memory with the process that reserves it, and free it up once the process is killed. Not sure; someone else can probably give you a better answer for this.
Regardless of the cause, if you see your memory shooting up like that, something seriously wrong is going on and should be fixed. My money's on surfaces being created every frame; you might want to check that. You mentionned loading graphical resources as they are needed before, maybe the problem's there? Don't forget to unload things after you stop needing them. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Fri Feb 10, 2006 8:04 am Post subject: |
[quote] |
|
Gardon wrote: | It's weird because once I quit the program all the memory frees up (so I guess it's not a memory leak). |
That's to be expected. The operating system releases all memory owned by an application when that application terminates.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
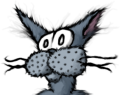
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Fri Feb 10, 2006 9:19 am Post subject: |
[quote] |
|
RuneLancer wrote: | If you have a memory leak, something's wrong. It's inacceptable for an app, no matter what the circumstances, to do this. |
Yeah, but applications --- games or otherwise --- still get away with it; while I love Firefox, its an absolute memory whore, and I know its large foot print has nothing to do with having a few tabs open. That is not to excuse the anti-pattern, but just to acknowledge that it exists in professional software, too.
Quote: | The reason for a memory leak to occure is because, in short, you're creating something in memory but never destroying it. The memory remains reserved and Windows cannot use it. What's worse, because nothing points to that memory anymore, it will never be released (until you reboot, that is.) |
That is somewhat untrue: programs allocate memory from two locations: "static memory" --- that is, non-malloc'd memory --- is allocated off of the program's stack, while "dynamic memory" --- objects that are malloc'd --- are allocated off of the program's heap, both segments of which are program* specific, and are generally collected by the operating system once the program has exited.
(*) Well, to be more precise, on systems that do not have a concept of parallel threads, one should talk about a process's memory space; when the environment supports threading, one should talk about a thread's memory space. The two may overlap, slightly, depending upon how the operating system links together interrelated threads and processes, where "overlap" should be taken to mean, "a thread's memory space is a mutually exclusive subset of its process's memory space." _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Fri Feb 10, 2006 8:14 pm Post subject: |
[quote] |
|
What about scrolling background? What's the best way to implement one?
jason
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|