View previous topic - View next topic |
Author |
Message |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Tue Jan 23, 2007 1:47 am Post subject: |
[quote] |
|
Quote: | That is not necessarily true: the wikipedia article on garbage collection suggests that it can execute faster than manual memory management in C++. |
The section which you read that suggests that GC can actually be faster than explicit allocation, provided two dubious examples:
C#
Code: |
class A {
private int x;
public A() { x = 0; ++x; }
}
class Example {
public static void Main() {
for (int i = 0; i < 1000000000; ++i) {
A a = new A();
}
System.Console.WriteLine("DING!");
}
}
|
vs the C++, equivalent
Code: |
#include <iostream>
class A {
int x;
public:
A() { x = 0; ++x; }
};
int main() {
for (int i = 0; i < 1000000000; ++i) {
A *a = new A();
delete a;
}
std::cout << "DING!" << std::endl;
}
|
This comparison is unfair because C++ example is clearly poorly written compared to the C# version. For one thing, when programming games, one would be stupid to do memory allocations in the way the example does it as above. The way to do it in C++, is to write custom memory allocators that work on pools of memory. By using memory pools, one reduces the overhead for allocation/deallocation by strategically arranging data into area of memory which can reused and would be optimized for data locality.
Furthermore, the wikipedia article in that section states that:
Quote: | For example, the tendency of the runtime system to pause program execution at arbitrary times to run the garbage collector may make GC languages inappropriate for some embedded systems, high-performance server software, and applications with real-time needs. |
which is exactly repeating what I said about GC in my previous post.
Code: | Regardless, your argument is slightly naive: you can turn off garbage collection in C# for classes you write, as well as provide explicit finalizers and destructors. |
Actually how do you turn it off? Destructors and Finalizers do not free the memory. It is stated in the MSDN that the GC does the memory freeing.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
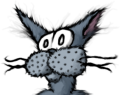
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Tue Jan 23, 2007 3:16 am Post subject: |
[quote] |
|
RedSlash wrote: | For one thing, when programming games, one would be stupid to do memory allocations in the way the example does it as above. The way to do it in C++, is to write custom memory allocators that work on pools of memory. By using memory pools, one reduces the overhead for allocation/deallocation by strategically arranging data into area of memory which can reused and would be optimized for data locality. |
Not to denigrate your point, but who does that? I can see that being done for, say, the latest 3d whiz-bang games that major game firms create, but for the usual fair output by the denizens of these fora? Please. The very need of having to come up with something that GC languages do automatically seems suspicious to me.
Quote: | [...]which is exactly repeating what I said about GC in my previous post. |
Sure, but not all GC schemes are, as the article refers to them, "stop-the-world" issues.
Quote: | Code: | Regardless, your argument is slightly naive: you can turn off garbage collection in C# for classes you write, as well as provide explicit finalizers and destructors. |
Actually how do you turn it off? Destructors and Finalizers do not free the memory. It is stated in the MSDN that the GC does the memory freeing. |
You can suppress finalization by invoking GC.SuppressFinalize; this can be called in a Dispose method, should you want to clean up your own resources. Useful if you are writing any unmanaged code, although I grant not exactly relevant to the conversation at hand. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Tue Jan 23, 2007 4:34 am Post subject: |
[quote] |
|
Quote: |
Not to denigrate your point, but who does that? I can see that being done for, say, the latest 3d whiz-bang games that major game firms create, but for the usual fair output by the denizens of these fora? Please. The very need of having to come up with something that GC languages do automatically seems suspicious to me. |
You bring up a good point though. If people aren't optimizing their C++ memory allocations schemes, they need better arguments for not using a GC.
Quote: | Sure, but not all GC schemes are, as the article refers to them, "stop-the-world" issues. |
No, GC is not stop-the-world when used in normal applications which tends to be 99% idle most of the time. The GC runs smoothly during those idle times and will have no noticable effect. In gaming however, where the CPU is 100% full, a 0.01 sec delay when playing Counterstrike due to the GC is quite stop-the-world for me. And yes, C# apps are noticably slower on my system.
Quote: | You can suppress finalization by invoking GC.SuppressFinalize; this can be called in a Dispose method, should you want to clean up your own resources. Useful if you are writing any unmanaged code, although I grant not exactly relevant to the conversation at hand. |
Yes, but this doesn't prevent the GC from running. The performance hit from the GC comes from its execution rather than having the capability of being able to explicitly deallocate resources. If we can disable its execution, then that would be a step forward.
Unfortunately, the GC is only one of the concerns of C# for use in gaming. I feel that C# is better geared for rapid application development where most of its features benefit. For games, I will stick with C++.
|
|
Back to top |
|
 |
tunginobi Wandering Minstrel
Joined: 13 Dec 2005 Posts: 91
|
Posted: Tue Jan 23, 2007 5:25 am Post subject: |
[quote] |
|
Quote: | Speed is king. Period. |
This raises an interesting point. It takes time and effort to write and debug a program. The costs of a program include development time, debugging and testing, as well as runtime performance. If that isn't the case, then why aren't we all writing assembly language code?
If the engine behind Counterstrike were being developed in pure assembly, I'm sure it would have come out later, had more bugs, and those bugs would take longer to patch.
3rd level languages were a calculated cost: any performance lost in the switch from pure assembly code would be more than made up for in development time, ease of maintenance and reduced debugging time. It's exactly the same thing with garbage collection. I don't mean nearly. I don't mean almost like. I mean exactly.
Let's step back and take things in context. XNA is intended for homebrew and hobbyist developers. Last I checked, demanding, high-performance games are rather few in number in the homebrew scene. Since arguments for C++'s speed only really make a difference in demanding, high-performance games, we turn to the other costs: the cost development. The cost of maintenance. The cost of debugging. The cost of learning the codebase in order to contribute to it. These are all big costs, costs which can be reduced with a language like C#.
Do I need to remind anybody that this discussion is not about "replacing C++ with C# in game dev", but rather "using C# for homebrew game dev"?
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Tue Jan 23, 2007 6:14 am Post subject: |
[quote] |
|
We wouldn't program in complete ASM despite that it has best performance because the development time is too high. But, C# has the fastest development time at the cost of having the lowest performance. And in my case, the performance impacts the way I run programs. C# apps are noticibly slower and occupies more memory on my system. This is why I am still skeptical about using C# for gaming. C++ is quite in between, being a good tool for speed and for rapid application development, though it may not be as nice as C#.
|
|
Back to top |
|
 |
DeveloperX 202192397
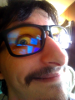
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Jan 23, 2007 6:24 am Post subject: |
[quote] |
|
I love how this thread has turned into a massive discussion, lol.
Oh so very interesting to see how you guys bitch at one another to get your point across, and everyone is thickheaded and closeminded to everyone else's suggestions...heh what a royal headache.
Honestly, we should spend more time developing and less time arguing over which language is best. I don't give a shit if C# is or is not the best to use for games, if I find it to be interesting enough for me to download the tools for 11 hours just to find that I needed another 8 hour download to make it all work, and still be interested in figuring out how it works should not really be a cause for you guys to argue its practicality. :P _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Nodtveidt Demon Hunter
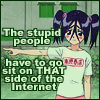
Joined: 11 Nov 2002 Posts: 786 Location: Camuy, PR
|
Posted: Tue Jan 23, 2007 9:30 am Post subject: |
[quote] |
|
LeoDraco wrote: | Pointing to a single application written in a framework which happens to be poorly written, and claiming that the framework itself must suck because that application runs poorly, is disingenuous. |
I was providing a singular example based on a program I have to use all the time. Take another look at my post; I did mention that I've used a lot of dotnet based apps and they are all slow. If your dotnet apps are fast, then obviously you're better than the so-called "professionals". Maybe you should go after their jobs. :) _________________ If you play a Microsoft CD backwards you can hear demonic voices. The scary part is that if you play it forwards it installs Windows. - wallace
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
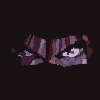
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Tue Jan 23, 2007 3:12 pm Post subject: |
[quote] |
|
I love whole argument of, "if we wanted everything fast, we'd write in ASM," since it's so wrong. Chips are being manufactured purely for compilers (lots and lots of specific functions), and compilers have been in the making for a looooong time to make things most efficient. These days you have to be some sort of genius to make an entire program in ASM run faster than the same algo in something like C or C++.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
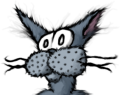
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Tue Jan 23, 2007 6:02 pm Post subject: |
[quote] |
|
nodtveidt wrote: | LeoDraco wrote: | Pointing to a single application written in a framework which happens to be poorly written, and claiming that the framework itself must suck because that application runs poorly, is disingenuous. |
I was providing a singular example based on a program I have to use all the time. Take another look at my post; I did mention that I've used a lot of dotnet based apps and they are all slow. If your dotnet apps are fast, then obviously you're better than the so-called "professionals". Maybe you should go after their jobs. :) |
I write dotnet applications for a living for a software company, which pays me for my time. I have yet to be fired for incompetency. I think that would tend to make me a professional, yes?
Your argument still does not hold: as I said, the plural of anecdote is not data, especially as I have an experience completely contrary to your own. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Tue Jan 23, 2007 7:31 pm Post subject: |
[quote] |
|
Programs are usually slow because of a poor choice of algorithms, not because they are programmed in a high-level language. If anything, high-level languages make it easier to use the choose the right algorithms and data structures.
That said, I am not fond of the .net framework at all. It carries a ton of overhead with it, and the use of bytecode interferes with compiler optimizations.
|
|
Back to top |
|
 |
tunginobi Wandering Minstrel
Joined: 13 Dec 2005 Posts: 91
|
Posted: Tue Jan 23, 2007 9:57 pm Post subject: |
[quote] |
|
Ninkazu wrote: | I love whole argument of, "if we wanted everything fast, we'd write in ASM," since it's so wrong. |
Meanwhile, ten years in the future...
Quote: | I love the whole argument of, "if we wanted everything fast, we'd avoid garbage collection," since it's so wrong. |
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Wed Jan 24, 2007 1:05 am Post subject: |
[quote] |
|
Quote: | the use of bytecode interferes with compiler optimizations |
I'm not exactly sure if this is entirely accurate. Optimization is performed before code output and usually how it works is to transform source code into sort of intermediate form such as an abstract data tree or the static single assignment form and optimizing that. After that, it's just a matter of turning the optimized code representation to bytecode and then to native code.
Well, I think we can all come to a conclusion that most people in this forums do not have games with high enough demands to not consider the use of RAD languages like C# or Java for their gaming needs. We all have different needs and reasons for choosing one language over another and that said, we can all continue to build games and as long as it runs good on my system then that's all that matters.
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Wed Jan 24, 2007 2:35 am Post subject: |
[quote] |
|
RedSlash wrote: | Quote: | the use of bytecode interferes with compiler optimizations |
I'm not exactly sure if this is entirely accurate. Optimization is performed before code output and usually how it works is to transform source code into sort of intermediate form such as an abstract data tree or the static single assignment form and optimizing that. After that, it's just a matter of turning the optimized code representation to bytecode and then to native code. |
There are low-level optimizations (such as instruction reordering) which are only possible in the code generation step. These optimizations take time. A just-in-time compiler can't afford to spend too much time on optimization, lest the optimization itself slows down the execution of the program.
Quote: | Well, I think we can all come to a conclusion that most people in this forums do not have games with high enough demands to not consider the use of RAD languages like C# or Java for their gaming needs. We all have different needs and reasons for choosing one language over another and that said, we can all continue to build games and as long as it runs good on my system then that's all that matters. |
I must object to calling Java a RAD language. My own experience with Java is that it actually increases development time compared to C++ because it it missing all of the high-level functionality of C++ (such as function templates, operator overloading, deterministic destructors, and multiple inheritance).
Don't get me wrong. C++ is an abomination. However, Java is not the answer. (No comments on C#, as I haven't used it.) If you have CPU cycles to burn, try Python for a real RAD language.
|
|
Back to top |
|
 |
Nodtveidt Demon Hunter
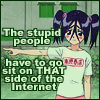
Joined: 11 Nov 2002 Posts: 786 Location: Camuy, PR
|
Posted: Wed Jan 24, 2007 3:08 am Post subject: |
[quote] |
|
LeoDraco wrote: | as I said, the plural of anecdote is not data, especially as I have an experience completely contrary to your own. |
You also have a habit of being unnecessarily wordy. :P _________________ If you play a Microsoft CD backwards you can hear demonic voices. The scary part is that if you play it forwards it installs Windows. - wallace
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
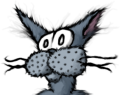
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Wed Jan 24, 2007 3:16 am Post subject: |
[quote] |
|
nodtveidt wrote: | LeoDraco wrote: | as I said, the plural of anecdote is not data, especially as I have an experience completely contrary to your own. |
You also have a habit of being unnecessarily wordy. :P |
And you have a habit of showcasing your intelligence through use of smilies. Hurray for ad hominem attacks! _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|