 |
|
View previous topic - View next topic |
Author |
Message |
valderman Mage
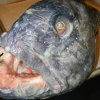
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Mon Apr 21, 2008 7:57 am Post subject: Features of a game scripting language |
[quote] |
|
Hello people, long time no see. How come everything has become so... Subdued, since my last visit?
Anyway, I'm currently working on (yet another) scripting engine, and I'm curious about what features people think might be useful.
The engine so far har the following features planned:
* Stack-based virtual machine executing precompiled bytecode (though programs can be passed directly from the compiler to the VM.)
* C-like syntax; the control structures if, switch, for and while are implemented, as are arrays, functions, all arithmetic operators, basic string manipulation and three basic data types: int, float and string.
* Ability to call native code from within scripts. This is accomplished by having the host program running VM.Map(namespace, func_name, func_ptr, num_args) to register native functions with the VM, then calling them from scripts with Namespace.Function(args) syntax.
* Ability to access native data from within scripts. Variables are registered and accessed in the same way as functions.
* Object-awareness. An instance of a C++ class may register itself as the owner of a script, and then register data and functions, in the same manner as described above, to be accessible to the script through the reserved 'this' namespace. This could be accomplished using the normal data and function bridges, but is provided for convenience.
Is there anything more that might be convenient? Something that you don't think should be in there? Something to implement differently?
I've already decided not to take it OO, as I want a simple scripting system, not some inner platform effect-ish C++ clone.
|
|
Back to top |
|
 |
Verious Mage

Joined: 06 Jan 2004 Posts: 409 Location: Online
|
Posted: Mon Apr 21, 2008 12:42 pm Post subject: |
[quote] |
|
I always found the following to be very handy shortcuts in C-style languages:
Code: | variable += someValue
variable++
variable-- |
In JavaScript, I really like the condensed "if" statements:
Code: | variable = (condition) ? true : false; |
|
|
Back to top |
|
 |
valderman Mage
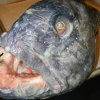
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Mon Apr 21, 2008 2:19 pm Post subject: |
[quote] |
|
Verious wrote: | I always found the following to be very handy shortcuts in C-style languages:
Code: | variable += someValue
variable++
variable-- |
In JavaScript, I really like the condensed "if" statements:
Code: | variable = (condition) ? true : false; |
| The operators ++, --, +=, -=, /= and *= are already planned for inclusion.
I'll probably avoid the ternary operator though, as it's far too easy to write clever (as in completely unreadable and unmaintainable after two weeks) code with it. I might add it later on though, as it's particularly well suited to a stack based architecture (far easier to implement than 'real' if constructs.)
|
|
Back to top |
|
 |
Mattias Gustavsson Mage

Joined: 10 Nov 2007 Posts: 457 Location: Royal Leamington Spa, UK
|
Posted: Mon Apr 21, 2008 4:10 pm Post subject: |
[quote] |
|
For me, the most important feature of any scripting language is that it is specifically tailored to the game it is to be used for. I've found that different types of games need different things from scripts, and if you custom build the scripting system for the game, you can end up with something which is very quick and easy to work with, which for me was always the point of scripts.
I see little use for generic scripting languages which does pretty much what c++ can already do better and faster. If that's the case, I might as well use c++ to do it in the first place...
That being said, a full-fledged scripting language generating bytecode for a virtual machine can be a very fun and rewarding thing to work on (I'm doing one myself right now, though for a slightly different purpose), and having fun is what's really important :-) _________________ www.mattiasgustavsson.com - My blog
www.rivtind.com - My Fantasy world and isometric RPG engine
www.pixieuniversity.com - Software 2D Game Engine
|
|
Back to top |
|
 |
valderman Mage
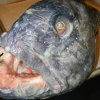
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Mon Apr 21, 2008 5:12 pm Post subject: |
[quote] |
|
Mattias Gustavsson wrote: | For me, the most important feature of any scripting language is that it is specifically tailored to the game it is to be used for. I've found that different types of games need different things from scripts, and if you custom build the scripting system for the game, you can end up with something which is very quick and easy to work with, which for me was always the point of scripts. | I disagree. The important thing is to have a scripting system that is generic enough to be reusable, yet easy to tailor to whatever game you want to use it for. What I'm aiming to create is a just a bunch of control structures and an easy way to interface with the game engine.
Of course different games differ in their additional requirements - an RPG would do well to have some kind of lightweight markup-like way to define items and dialogue, for example - but the basic building blocks tend to stay the same.
|
|
Back to top |
|
 |
Mattias Gustavsson Mage

Joined: 10 Nov 2007 Posts: 457 Location: Royal Leamington Spa, UK
|
Posted: Mon Apr 21, 2008 5:29 pm Post subject: |
[quote] |
|
I dunno, for the games I've worked on, having a generic, reusable scripting language wasn't very useful. It just meant the scripters needed to be full-fledged programmers, who just got frustrated by having to use a scripting language rather than C++...
A couple of times I've worked on project which had VERY specific scripting languages (one even used Visio, a diagram drawing tool, to define scripts), and with those, all of the designers could get their hands dirty with scripting the game, which really helped.
In my experience, reuse rarely works well in the games industry. I personally think it is because of the staff turnover, in that new leads decide to throw away a lot of the old stuff because they can't be bothered to learn it... :P _________________ www.mattiasgustavsson.com - My blog
www.rivtind.com - My Fantasy world and isometric RPG engine
www.pixieuniversity.com - Software 2D Game Engine
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Tue Apr 22, 2008 1:58 am Post subject: |
[quote] |
|
These would be my requirements would I choose to use your scripting vm.
1. Supports threads or co-routines
2. Automatic variable types instead of having to declare int, float or string
3. Automatic garbage collection or reference counting.
4. Associative arrays
5. For each loop
6. Array/string splicing (like in python, e.g. mystring[1,5])
7. JIT would be nice
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Tue Apr 22, 2008 3:54 am Post subject: |
[quote] |
|
Automated serialization. In particular, serialization of coroutine states.
|
|
Back to top |
|
 |
valderman Mage
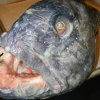
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Tue Apr 22, 2008 10:10 am Post subject: |
[quote] |
|
RedSlash wrote: | These would be my requirements would I choose to use your scripting vm.
1. Supports threads or co-routines
2. Automatic variable types instead of having to declare int, float or string
3. Automatic garbage collection or reference counting.
4. Associative arrays
5. For each loop
6. Array/string splicing (like in python, e.g. mystring[1,5])
7. JIT would be nice | Threads are sort-of in there, in that you can run several scripts in parallell, in their own separate sandbox. Building on its capability to call native code, adding IPC and synchronisation would probably be trivial. I'm thinking of adding a kind of "standard library" (functionality not included in the language itself, but callable through standard interfaces - again, think C (stdlib, except way way smaller)) that does just that, better string manipulation, trig math, etc.
Maybe it could even be used to implement coroutines.
Types are strictly enforced, though casting between all three types is supported. Variables don't need to be declared, but inherits their type from the expression first assigned to them.
No dynamic management, so no need for garbage collection. Again, might add it to the standard library.
Associative arrays not originally planned, though they would definitely be useful. Adding them probably wouldn't be too much of a hassle. Thanks a lot for that one!
Same thing with foreach, going to look into that.
Better string manipulation is definitely going into the standard library.
JIT would indeed be nice, though that's low-priority for now.
Mattias Gustavsson wrote: | I dunno, for the games I've worked on, having a generic, reusable scripting language wasn't very useful. It just meant the scripters needed to be full-fledged programmers, who just got frustrated by having to use a scripting language rather than C++...
A couple of times I've worked on project which had VERY specific scripting languages (one even used Visio, a diagram drawing tool, to define scripts), and with those, all of the designers could get their hands dirty with scripting the game, which really helped.
In my experience, reuse rarely works well in the games industry. I personally think it is because of the staff turnover, in that new leads decide to throw away a lot of the old stuff because they can't be bothered to learn it... :P | It's a world of difference between being able to do something like if(player.level < 10) player.GiveItem(items.funny_hat); and being a full-fledged programmer.
Some kind of syntax is always going to be necessary (unless you use Visio or similar tools,) so you might as well create one base to work from, so people don't need to relearn as much. The fact that it might support fancy features doesn't mean that they're going to be used in all (or even most) most cases.
Considering that most game designers are at least capable of writing Hello World, I think the power of a real language for scripting is preferrable to the ease of use of a diagram drawing tool. Though of course that depends on what you're trying to accomplish (just look at the tools used for most Japanese eroge - they could probably get by with PowerPoint.)
|
|
Back to top |
|
 |
Hajo Demon Hunter
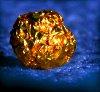
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Tue Apr 22, 2008 10:28 am Post subject: |
[quote] |
|
valderman wrote: |
Considering that most game designers are at least capable of writing Hello World, I think the power of a real language for scripting is preferrable to the ease of use of a diagram drawing tool. |
Doing a project for the fun and maybe learning is perfectly fine. I did that often too. But there are nice generic scripting language like Lua, and the more mighty like Ruby, Python and such, which will compete with our project.
But I wrote my own scripting language once, too, when I was studying compiler construction, so don't get me wrong. I'm not against such a project, just saying it's hard to find users, since there are so many options for them.
|
|
Back to top |
|
 |
valderman Mage
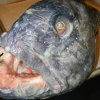
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Tue Apr 22, 2008 10:40 am Post subject: |
[quote] |
|
Hajo wrote: | valderman wrote: |
Considering that most game designers are at least capable of writing Hello World, I think the power of a real language for scripting is preferrable to the ease of use of a diagram drawing tool. |
Doing a project for the fun and maybe learning is perfectly fine. I did that often too. But there are nice generic scripting language like Lua, and the more mighty like Ruby, Python and such, which will compete with our project.
But I wrote my own scripting language once, too, when I was studying compiler construction, so don't get me wrong. I'm not against such a project, just saying it's hard to find users, since there are so many options for them. | Of course, I'm only creating this language for the fun of it and for my own use. I can't be arsed to choose one of [myriad of good free scripting languages], much less learn it. Besides, I like C-like syntax whereas most good scripting languages don't.
I definitely don't expect anyone else than me to use it, considering the many good options, but was merely arguing the usefulness of a generic, reusable scripting language.
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Wed Apr 23, 2008 12:56 am Post subject: |
[quote] |
|
Quote: | No dynamic management, so no need for garbage collection. |
The garbage collection feature would be to support the user's defined dynamic objects rather than your own scripting system's. For example, if I have a native C routine LoadImage() which I register as a function in your VM, it would nice to have the capability to register a destructor function which would automatically call my native C FreeImage() function once the object goes out of scope.
|
|
Back to top |
|
 |
valderman Mage
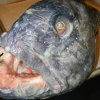
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Wed Apr 23, 2008 4:56 am Post subject: |
[quote] |
|
[quote="RedSlash"] Quote: | No dynamic management, so no need for garbage collection. |
The garbage collection feature would be to support the user's defined dynamic objects rather than your own scripting system's. For example, if I have a native C routine LoadImage() which I register as a function in your VM, it would nice to have the capability to register a destructor function which would automatically call my native C FreeImage() function once the object goes out of scope.[/quoteThat would indeed be nice, but seems better implemented in the aforementioned standard library (or the user's preferred LoadImage function) than in the engine itself.
|
|
Back to top |
|
 |
Jinroh Scholar

Joined: 30 May 2008 Posts: 173 Location: U.S.A.
|
Posted: Fri May 30, 2008 7:42 pm Post subject: |
[quote] |
|
I agree making your own Scripting Language is beneficial not to mention fun.
I recently made a BASIC Interpreter that I am morphing into a Scripting Language.
I like that you're making each Script it's own contained sandbox. I did the same thing. That way you can just fire and forget more or less.
Though mine is waaay more simple syntax wise than C it's pretty much like a C64 BASIC or some 8-Bit Equivalent.
Hope to get to see it running something soon. :)
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|