 |
|
View previous topic - View next topic |
Author |
Message |
DeveloperX 202192397
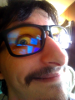
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Wed Oct 08, 2008 12:16 pm Post subject: (need help) C/C++ void pointers and string array issues |
[quote] |
|
Okay I have been using too many other languages recently and I cannot remember a few things regarding C/C++. hopefully there is someone here who can give me a shove in the right direction. :)
I have a need to pass an array of C strings to a function for processing.
My code does not work, and I'm unfortunately both too tired, and too out of practice to find the problem.
The setup is here
Code: |
SetFunc(&Demo::TestFunction);
char* test[] =
{
(char*)"This is",
(char*)"a more",
(char*)"complicated",
(char*)"test",
(char*)"function."
};
CallFunc(test);
|
and the TestFunction source is currently this
Code: |
void Demo::TestFunction(void* args)
{
printf("This is a test.\n");
if (args)
{
char** str = (char**)args;
for (int i = 0; i < sizeof(str) / sizeof(char*); i++)
{
printf("argument %4d: %s\n", i, str[i]);
}
printf("\n");
}
}
|
*sighs*
I feel really shitty having to ask for help with this..so be kind :P _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
DeveloperX 202192397
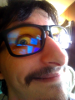
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Wed Oct 08, 2008 8:51 pm Post subject: |
[quote] |
|
okay, after rethinking the problem, I decided to try again.
I got it this time. My solution involves a new struct.
Code: |
typedef struct TextChunk_Type
{
unsigned int chunkSize;
char** chunkData;
} TextChunk, *TextChunkPtr;
|
and here is the main setup code
Code: |
SetFunc(&Demo::TestFunction);
char* test[] =
{
(char*)"This is",
(char*)"a more",
(char*)"complicated",
(char*)"test",
(char*)"function."
};
TextChunk chunk;
chunk.chunkSize = sizeof(test) / sizeof(char*);
chunk.chunkData = test;
CallFunc((void*)&chunk);
|
and the new TestFunction:
Code: |
void Demo::TestFunction(void* args)
{
printf("This is a test.\n");
if (args)
{
TextChunk* chunk = (TextChunk*)args;
char** str = chunk->chunkData;
for (int i = 0; i < chunk->chunkSize; i++)
{
printf("argument %4d: %s\n", i, str[i]);
}
printf("\n");
}
}
|
see any better ways outside of using any STL code?
Let me know. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Thu Oct 09, 2008 12:43 am Post subject: |
[quote] |
|
Another common method to do passing variable argument arrays is to use NULL as a end of items indicator. e.g.
Code: |
char* test[] =
{
(char*)"This is",
(char*)"a more",
(char*)"complicated",
(char*)"test",
(char*)"function.",
NULL // <----- indicates end of items
};
|
Your code knows when to stop iterating when it reaches the NULL pointer. This works well when the number of items cannot be determined at compile time.
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|