View previous topic - View next topic |
Author |
Message |
valderman Mage
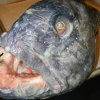
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Sat Nov 01, 2008 2:08 pm Post subject: |
[quote] |
|
Captain Vimes wrote: | I still don't really get why anyone would use references | You get most of the benefits of a pointer, but with the simplicity and ease of use of a normal variable. Take this method, for example:
Code: | void RenderManager::Draw(Sprite *s, int x, int y) {
// draw the sprite to (x, y)
} |
It takes a pointer to a sprite and a position, and then draws the sprite to that position. Now, this isn't a very good method definition. Why? Because that Sprite pointer might be just about anything - chances are it will be a pointer to a Sprite object in most cases, but it might just as well be NULL, -1, a pointer to a deleted Sprite object, a pointer som another kind of object or just random garbage because somewhere a pointer wasn't initialised correctly.
If you write a method like this, it's pretty much compulsory to at least check that it isn't NULL. This doesn't fit very nicely with OO though, because the method imposes additional restrictions on the pointer, other than the ones imposed by its type.
If you were to instead write it like this:
Code: | void RenderManager::Draw(Sprite &s, int x, int y) {
// draw the sprite to (x, y)
} |
You would have accomplished the following improvements:
* s will never be an invalid pointer. One huge source of errors out of the way.
* You don't have to care that s is not really an actual object; for all intents and purposes, it is.
* You don't impose additional restrictions on your input. Any value for s that is accepted by the compiler will also be OK to use within the method.
* You still have the performance and memory gains of passing a pointer instead of passing by value.
* Since a reference is more locked down than a pointer, the compiler has a better chance of doing fancy optimisations.
However, this would be even better:
Code: | void RenderManager::Draw(const Sprite &s, int x, int y) {
// draw the sprite to (x, y)
} |
A constant reference!
This has all the benefits of the normal reference, but also shows that the method will never modify s. This is good because you don't have to look for bizarre side effects in the method before using it, you'll never accidentally change s while writing the method, and the compiler, having even more information at its disposal, has an even better chance at making the best possible optimisations.
Not bad for such a tiny change, eh? _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Sat Nov 01, 2008 5:22 pm Post subject: |
[quote] |
|
Quote: | A constant reference!
........
Not bad for such a tiny change, eh? |
This would require his Sprite class to be 'const correct' as well. Here's an example, from Sprite.h
Code: |
class Sprite
{
.
.
int GetX();
int GetY();
.
.
};
|
If you pass Sprite as a const reference, you will not be able to call GetX or GetY, because as it stands, these methods say they might modify variables inside the Sprite class.
To make it 'const correct':
Code: |
class Sprite
{
.
.
int GetX() const;
int GetY() const;
.
.
};
|
Now, GetX() and GetY() are declared to not modify variables inside Sprite, and thus will work when passed as a const reference.
|
|
Back to top |
|
 |
DeveloperX 202192397
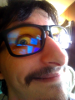
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Nov 01, 2008 5:26 pm Post subject: |
[quote] |
|
Captain:
I generated some html docs using Doxygen of your original sources and the refactored sources I made for you; for no real reason, other than to test doxygen out. But I think it may be useful to you maybe.
So here it is.
Original
Refactored _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
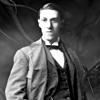
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Tue Nov 04, 2008 1:11 pm Post subject: |
[quote] |
|
Ummm... thanks? I'm sorry, I opened up the file and still couldn't figure out exactly what Doxygen is. Could you explain please? And why are the classes now .html files? _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
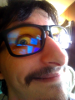
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Nov 04, 2008 2:00 pm Post subject: |
[quote] |
|
Captain Vimes wrote: | Ummm... thanks? I'm sorry, I opened up the file and still couldn't figure out exactly what Doxygen is. Could you explain please? And why are the classes now .html files? |
HAHA
Doxygen is a program that automatically generates documentation for your projects. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Verious Mage

Joined: 06 Jan 2004 Posts: 409 Location: Online
|
Posted: Tue Nov 04, 2008 2:03 pm Post subject: |
[quote] |
|
Doxygen generates documentation from source code. Unzip the files and then open "index.html" to see the results.
I believe the files DeveloperX prepared only include the source files in documentation format (HTML), not the original source files (used to build the application).
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
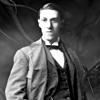
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Tue Nov 04, 2008 2:51 pm Post subject: |
[quote] |
|
Oh, okay. I get it now. That does help a lot. I just didn't see the index.html file in the middle of all that stuff.
Thanks for all your help, everybody - especially you, DevX. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
valderman Mage
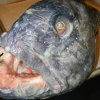
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Tue Nov 04, 2008 4:18 pm Post subject: |
[quote] |
|
RedSlash wrote: | Quote: | A constant reference!
........
Not bad for such a tiny change, eh? |
This would require his Sprite class to be 'const correct' as well. Here's an example, from Sprite.h
Code: |
class Sprite
{
.
.
int GetX();
int GetY();
.
.
};
|
If you pass Sprite as a const reference, you will not be able to call GetX or GetY, because as it stands, these methods say they might modify variables inside the Sprite class.
To make it 'const correct':
Code: |
class Sprite
{
.
.
int GetX() const;
int GetY() const;
.
.
};
|
Now, GetX() and GetY() are declared to not modify variables inside Sprite, and thus will work when passed as a const reference. | I didn't even think of that - another bonus, right there! _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
DeveloperX 202192397
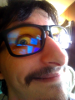
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Nov 04, 2008 10:35 pm Post subject: |
[quote] |
|
Captain Vimes wrote: | Oh, okay. I get it now. That does help a lot. I just didn't see the index.html file in the middle of all that stuff.
Thanks for all your help, everybody - especially you, DevX. |
You are most welcome.
Hey, I noticed you joined my forums, but you have not posted anywhere, nor have you signed in. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
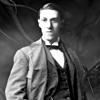
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Thu Nov 06, 2008 1:11 pm Post subject: |
[quote] |
|
Haven't got a chance. That's actually what I'm going to do now. See you there. Look for the 8-Bit D&D avatar :D _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
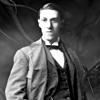
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Thu Nov 06, 2008 2:56 pm Post subject: |
[quote] |
|
Okay, so, after posting on NOGDUS, I went back and tried out the newly-updated Hammer and associated classes. The first thing I noticed was that neither the SpriteManager nor the Hammer had any function to update the position of all the sprites in the list, so I added that. I'm going to go try it out now. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
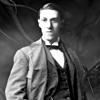
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Thu Nov 06, 2008 3:05 pm Post subject: |
[quote] |
|
Tried it. Compiles fine, everything draws okay, but I can't seem to move the little guy in the center of the screen. The current thing is just a smiley face on a blue background. The magic pink disappears as it's supposed to, and the program doesn't crash, so everything's fine except my added Update() functions. I'll go check them. More info later. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
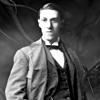
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Thu Nov 06, 2008 3:12 pm Post subject: |
[quote] |
|
QUADRUPLEPOST! IT COULD BE A NEW RECORD!
Anyway, I found out what the problem was. It wasn't my Update() functions - I was trying to use DevX's timer code (you remember, with float deltaTime and everything?), but because of the int cast when I multiplied the change in time by the sprite's velocity it always ended up with a total position change of 0, so none of the sprites would ever move. I think I was implementing the code wrong, but I decided to just scrap it and go with my old if(SDL_GetTicks() % 10 == 0) { //do stuff } code, since it has served me well in the past. It works now. I'll post some screens when I actually get a little bit of game ready.
EDIT: I figured a quintuple post would just be getting out of hand, so here's an edit instead.
I tried to run the project's .exe file as an experiment, and it said that it couldn't find SDL.dll. But I clicked Run from the Dev-C++ workstation and the program ran fine. How do I make it so that I can just run the program off the .exe file instead of having to go into Dev-C++ every time? _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Thu Nov 06, 2008 4:40 pm Post subject: |
[quote] |
|
By putting SDL.dll in the same directory of the exe.
|
|
Back to top |
|
 |
DeveloperX 202192397
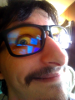
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Thu Nov 06, 2008 5:33 pm Post subject: |
[quote] |
|
Captain Vimes wrote: | QUADRUPLEPOST! IT COULD BE A NEW RECORD!
Anyway, I found out what the problem was. It wasn't my Update() functions - I was trying to use DevX's timer code (you remember, with float deltaTime and everything?), but because of the int cast when I multiplied the change in time by the sprite's velocity it always ended up with a total position change of 0, so none of the sprites would ever move. I think I was implementing the code wrong, but I decided to just scrap it and go with my old if(SDL_GetTicks() % 10 == 0) { //do stuff } code, since it has served me well in the past. It works now. I'll post some screens when I actually get a little bit of game ready.
EDIT: I figured a quintuple post would just be getting out of hand, so here's an edit instead.
I tried to run the project's .exe file as an experiment, and it said that it couldn't find SDL.dll. But I clicked Run from the Dev-C++ workstation and the program ran fine. How do I make it so that I can just run the program off the .exe file instead of having to go into Dev-C++ every time? |
The solution is to place SDL.dll in your PATH. I recommend C:\Windows\System32\
As for the timing code; Give me a moment to type up a complete example instead of a snippet as before.
(edit)
Here you go man
http://www.ccpssolutions.com/storage/captainvimes/hammer_with_timing.zip _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|