View previous topic - View next topic |
Author |
Message |
Captain Vimes Grumble Teddy
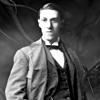
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Wed Mar 11, 2009 5:48 pm Post subject: |
[quote] |
|
Okay, okay. I've been digging around in the code, since I tried to make some changes to the Sprite class so that it could be animated. But I unfortunately chose the same time to switch to Code::Blocks, so now I'm not sure of whether this is a problem made from my own faulty programming or the IDE.
DevX, you made this version of the Sprite. I'm not really sure what everything after the colon in the Sprite::Sprite() constructor is for, but I think that might have something to do with it since I experimented with changing the order when I was getting obscure errors pointing to those lines. What do they do? I think you explained this before, but I didn't really get it then.
Oh, and the current constructor:
Code: | Sprite::Sprite(SDL_Surface* image, SDL_Surface* surface, float x, float y, bool visible,
int w, int h, float xVel, float yVel) :
velocityX_(0.0f),
velocityY_(0.0f),
visible_(false),
image_(0),
surface_(0),
source_(0),
destination_(0)
{
// first check that we have valid SDL_Surface pointers
if (0 == image || 0 == surface)
{
// failed!
// show the address and the values of the pointers
fprintf(
stderr,
"Fatal Error in Sprite::Sprite()\n\t"
"image and or surface are NULL!\n\t"
"image (%8X) = %8X\n\t"
"surface (%8X) = %8X\n",
&image,
&surface,
image,
surface);
exit(1); // I'm bailing out the application here - you might want to use logfiles instead but its not needed
// as things should never crash like this when you are releasing your game.
}
// ok, we have good pointers, set the member variables
image_ = image;
surface_ = surface;
// create the source and destination rectangles
source_ = new SDL_Rect;
destination_ = new SDL_Rect;
// test x
if (-1 != (int)x) { positionX_ = x; }
// test y
if (-1 != (int)y) { positionY_ = y; }
// set visibility
visible_ = visible;
// test w
if (-1 != w) { source_->w = w; } else { source_->w = image_->w; }
// test h
if (-1 != h) { source_->h = h; } else { source_->h = image_->h; }
source_->x = 0;
source_->y = 0;
// test xVel
if (-1 != (int)xVel) { velocityX_ = xVel; }
// test yVel
if (-1 != (int)yVel) { velocityY_ = yVel; }
// set the colorkey to magenta (magic pink) RGB(255, 0, 255) #FF00FF
SDL_SetColorKey(image_, SDL_SRCCOLORKEY, SDL_MapRGB(image_->format, 255, 0, 255));
} |
_________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
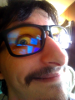
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Wed Mar 11, 2009 6:58 pm Post subject: |
[quote] |
|
The stuff after the colon is called an initializer list.
Its just short-hand for writing the assignments yourself.
See below:
Lets take this simple class for example:
Code: |
class Person
{
public:
Person(int age, const char* name);
int age_;
std::string name_;
};
|
these two pieces of code do the same thing:
Code: |
Person::Person(int age, const char* name)
{
age_ =age;
name_ = name;
}
|
Code: |
Person::Person(int age, const char* name) :
age_(age),
name_(name)
{
}
|
Get it?
Once thing about the errors regarding those lines.
The order of the variables in the initializer list need to be the same order that the variables are defined in the class.
For example,
Code: |
Person::Person(int age, const char* name) :
name_(name),
age_(age)
{
}
|
would give the warning/errors about assignments happening first.
Ok?
I don't see anything wrong in the code you posted. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
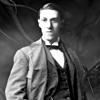
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Thu Mar 12, 2009 6:10 pm Post subject: |
[quote] |
|
Okay, that helps. Now I know why they're there.
But then what's the point of all the code in the constructor that just redefines them? If they're already defined in the initializer list, can't you just leave them alone? _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
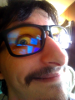
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Thu Mar 12, 2009 9:34 pm Post subject: |
[quote] |
|
Captain Vimes wrote: | Okay, that helps. Now I know why they're there.
But then what's the point of all the code in the constructor that just redefines them? If they're already defined in the initializer list, can't you just leave them alone? |
We use the initializer list to ensure that everything gets zeroed out and in the constructor we do the actual initialization.
And if you pay attention to what each line is doing, we are not just redefining. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Thu Mar 26, 2009 10:10 pm Post subject: |
[quote] |
|
It doesn't really make sense to set image_ to 0 first and then only assign "image" if "image != 0". Better just do image_(image) in the first place. :-)
Similarly you could just do "source_(new SDL_Rect)" in the initializer list. Also, positionX_/Y_ are only properly initialized if x/y don't cut off to -1.
|
|
Back to top |
|
 |
 |
Page 2 of 2 |
All times are GMT Goto page Previous 1, 2
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|