View previous topic - View next topic |
Author |
Message |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Fri Jul 09, 2010 11:13 pm Post subject: Gamestar |
[quote] |
|
The GCS module project is on indefinite hold... I've kinda lost interest.
What I'm working on right now (have been working on in terms of my more recent developments) is a Javascript-based engine that is nearing completion. This engine runs on a dialect of BASIC and fulfills the "virtual machine" requirement of the GCS project. Although I could just , I haven't needed to so far because it's Javascript/DHTML which is kinda modular in itself.
The design is an entity-based system similar to DIV. It's like the SceneLion scene graph software I made earlier, but has interactivity and multiple maps. My aim is for it to be capable of doing action RPGs, tactical RPGs, and anything in between.
I intend to run an online tactical RPG with it. I'm thinking of making the game an open world where people can create whatever characters they want -- the only condition is that they must be "free" (CC-SA or something similar). The play will be similar to Shining Force, and will feature the persuasion system I discussed in this thread. The game will have an overarching storyline, the theme of which will be "the challenge of promoting a new idea".
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Thu Jul 22, 2010 11:55 pm Post subject: |
[quote] |
|
I said I'd make this thing easy, and easy it damn well is. I have added several commands specific to the design of inventories and equipment.
The inventory system relies on three fundamental elements: keys, lists, and slots. The list is an array vector that can also be accessed as a hash. Lists consist of items that fit in designated slots. Slots are created with preset limits which constrain the number of items of a given type that a list can hold.
Here's an example.
Code: |
Dim inventory as list
add slot "apple" to inventory as type "food" limit 99
add item "apple" to inventory
dim key recoveryEquip as type "food"
recoveryEquip = inventory["apple"]
|
The first line declares the variable "inventory "as type "list". The second line adds a slot "apple" to inventory, specifying both the type of the item and the limit it can hold. The third line inserts an apple into inventory's "apple" slot, raising the count of that slot by 1. The fourth line declares recoveryEquip as a key/value pair of value type "food", and the fifth line assigns an apple from the inventory to recoveryEquip.
recoveryEquip will only accept items from a slot that is both filled and of the same type as itself. Else, it returns an error.
Here's how it works in practice:
- keys, lists, and slots are established when the game loads
- items are added to inventories by actors.
- the variables whose values are to become the values of the keys are changed when actors interface with the inventory/equipment system. (however that system is designed).
That's really about as easy as I can make it without getting more concrete. Hmm... maybe I should get more concrete.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Mon Jul 26, 2010 6:34 pm Post subject: |
[quote] |
|
I made it more concrete. The key system became an equip system, and the list system became a simple inventory system. However, I may refine it to allow for multiple equip systems and multiple inventories on the same character.
I also added a new variable type, "counter". Counter variables are variables that have an arbitrary limit. When this limit is passed, a specified flag variable is set and the overflow is voided out. Variables like these are great for HP and magic limits (like 999) and for terminal velocity values when applying gravity. When the value falls below the limit, the flag is unset.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Fri Sep 24, 2010 8:17 pm Post subject: |
[quote] |
|
I added in a map definition language, based on BASIC. It seemed a choice between something mostly numeric and XML, so I went down the middle and wrote the thing in BASIC. It's somewhat bulky, but it's probably cleaner than XML.
Here's a sample. It defines a 2x2x2 map.
Code: |
BEGIN MAP NewMap
@SIZE : 32 'Tile size
@WIDTH : 2
@HEIGHT : 2
BEGIN TILES
1 : shore.png
2 : water.png
END TILES
BEGIN LAYER Water
1 : 2 'Tile offsets are numbered, and are assigned the numbers of tiles in the tiles section. This line sets the first tile to water.png.
2 : 2
3 : 2
4 : 2
END LAYER
BEGIN LAYER Land
1 : 1
2 : 1
3 : -1 '-1 tells the map renderer not to draw the tile.
4 : -1
END LAYER
END MAP
|
|
|
Back to top |
|
 |
Malignus Scholar
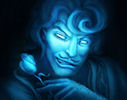
Joined: 12 May 2009 Posts: 198
|
Posted: Fri Sep 24, 2010 10:41 pm Post subject: |
[quote] |
|
How does the tile numbering go: across or vertically? Which is to say:
1 2
3 4
or
1 3
2 4
?
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sat Sep 25, 2010 7:01 pm Post subject: |
[quote] |
|
Across.
I like it because BASIC syntax is so much easier to parse than XML, although I grant that XML has its uses.
BASIC is ideal for parsing key/value pairs.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sun Oct 24, 2010 9:52 am Post subject: |
[quote] |
|
Got the map editor functional... layers, persistent world scripting (that feature I had planned for Worldyne, where you could edit the world in real time). So now I'm at the tweaking phase.
For my first game, going to make it something like Tower of Druaga, I think; that is, tile-x-tile movement. There will be a jumping function similar to Final Fantasy Mystic Quest. Think of it as FFMQ with a Tower of Druaga-like fighting system.
Gameplay centers around limited energy and fatigue. Everything requires energy aside from standing still. When you stand still, you gradually recover energy. Walking takes a little bit of energy. Items take a little more, and fighting takes a lot. Monsters also have energy, and change their behavior based on how much energy they have available.
Here's an example monster script that I made for testing purposes:
Code: |
BEGIN ACTOR Kobold
ON INIT
Self.Energy = 40
Self.HP = 20
Self.EXP = 5
END
ON ACTION
adjacentN = @Y - 1
adjacentS = @Y + 1
adjacentE = @X + 1
adjacentW = @X - 1
playerIsNorth1 if adjacentN = @Player.Y
playerIsSouth1 if adjacentS = @Player.Y
playerIsEast1 if adjacentE = @Player.X
playerIsWest1 if adjacentW = @Player.X
playerIsParallelAtY if @Y = @Player.Y
playerIsParallelAtX if @X = @Player.X
playerIsAdjacentN if playerIsNorth1 & playerIsParallelAtX
playerIsAdjacentS if playerIsSouth1 & playerIsParallelAtX
playerIsAdjacentE if playerIsEast1 & playerIsParallelAtY
playerIsAdjacentW if playerIsWest1 & playerIsParallelAtY
strikeIsPossible if Self.Energy >= 10
moveIsPossible if Self.Energy >= 1
if playerIsAdjacentN && strikeIsPossible {
@Frame = AttackN
playerIsAttacked = "true"
AttackingMonster = @Name
Self.Energy - 10
Idle 10
end
else
if playerIsAdjacentS && strikeIsPossible {
@Frame = AttackS
playerIsAttacked = "true"
AttackingMonster = @Name
Self.Energy - 10
Idle 10
end
else
if playerIsAdjacentE && strikeIsPossible {
@Frame = AttackE
playerIsAttacked = "true"
attackingMonster = @Name
Self.Energy - 10
Idle 10
end
else
if playerIsAdjacentW && strikeIsPossible {
@Frame = AttackW
playerIsAttacked = "true"
attackingMonster = @Name
Self.Energy - 10
Idle 10
end
else
if moveIsPossible {
Move Towards Player
Self.Energy - 1
Idle 4
else
Self.Energy + 1
Idle 4
}
END
ON CONTACT WITH PlayerSword
Self.HP - PlayerSwordPower
if Self.HP < 0 {
PlayerEXP + Self.EXP
HIDE
@Active = 0
}
END
END ACTOR
|
There are events provided for in the code: the INIT event which triggers when the actor is created; the ACTION routine which triggers in the absence of an event; and the PlayerSword CONTACT event, triggered on collision with the player's weapon. (you can guess what it does).
Property variables are prefixed with "@" -- changing them invokes a function of the game engine. Setting the "Active" property activates or deactivates an actor. Setting the frame, changes the frame number of the sprite shown. The Move statement swaps frames automatically as the sprite moves. Variables private to an actor are distinguished from public variables by the "Self." prefix.
The scripting engine isn't advanced enough for complex expressions (no parentheses), so it's important to break up conditional blocks into separate tests. Thus I've "if" as an alternative to "=" when assigning the results of comparative tests. (much better than "X = Y = D", or "X = Y == D"). One thing that is immediately apparent, given the complexities of computing adjacency, is that there is need to automate the procedure. I'm thinking about implementing a "adjacency" property which performs the computations involved in computing adjacency. These could also be used for simple line of sight algorithms.
In general I've tried to make this engine as interaction-based as possible, so as to create a situation where problems don't have to be deeply abstracted before being solved. For example, by this system, if you want to flash numbers for whatever reason (damage, healing, etc.) then you just have to put the triggering actor over the targeted actor, and the target will "react" by flashing the numbers.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Fri Oct 29, 2010 4:14 am Post subject: |
[quote] |
|
With the map system done, all that's left is the task of organizing the maps into a coherent game system. Simplifying this task isn't easy: the reigning method is a confusing "project" concept borrowed from Visual BASIC. What we want to do is make project consolidation as easy as possible without sacrificing power.
The game is made up of tile maps, which run on boards. Each board has a player character which is able to move between maps. This allows the user on the one hand to move about the game world, and on the other to interact with various menu systems, all of which use different maps. The alternative is a layers system which is altogether too confusing.
To demonstrate the power of this design, consider the case of a Zelda III clone. To faithfully reproduce the Zelda III engine, you need a HUD map, a text display layer, a layer where game play takes place, and an inventory screen. In Gamestar, each of these would use a different board, complete with its own set of maps. For the dialogue layer, you might only need 1 map; for the HUD, you might need several (especially for dungeons); 1 map for the inventory; and finally however many maps as exist in the actual game world. To transfer control between maps, you activate and deactivate action on these maps in accordance with the situation. Deactivating the player, for example, prevents further action along these maps. The maps never leave memory, and are a part of the fabric of the in-game situation. This makes complex designs trivial to implement.
Boards are setup through a 2 step process:
- Board is registered at the config menu and saved to a (user specified) configuration preset file.
- Starting maps for boards registered in the config preset are specified in game presets. This way you don't have to load a config preset for every game.
For testing purposes, I also intend to implement game state files which will permit the preloading of actor properties. This will allow that maps be play tested in various states of character development, instead of requiring that the game be gradually played through.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sat Oct 30, 2010 1:26 am Post subject: |
[quote] |
|
Actually, I'm thinking about just having every game be a list of maps. This way you could add maps to a game, then load the game itself in the editor later and choose from a list of maps to edit in the game. I guess this is closer to what's normally done, and now I see the reason.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Fri Feb 25, 2011 3:56 pm Post subject: |
[quote] |
|
Here's my new design.
- Make each game a list of maps.
- Preset configurations can be loaded into a game which specify cell size, tile size, screen dimensions (in tiles), and number of concurrent maps (useful for such features as HUDs, menus, and battle systems).
I'm thinking through a save file design now as well.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Tue Mar 08, 2011 6:23 am Post subject: |
[quote] |
|
I discarded the map list design. If I had implemented it, it would have meant using too much memory (enough to hold all the maps in memory at once).
I implemented the boards and the presets. I also implemented a variable watch system and a function for "committing" preloaded variable values.
I believe I'm a few hours away from finishing the project, but I lack motivation.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Thu Mar 17, 2011 7:39 am Post subject: |
[quote] |
|
OK I think I've found some motivation. However, this motivation is the encouragement of creativity. As such, I'm only going to release the single board version. The version I am releasing contains 16x16 tile maps which do not scroll. And, there is only one map per game.
I want to turn the clock back to the single-screen play era, and see what can be done with it.
If the response is good, I may find motivation to release a side-to-side scrolling version... something along the lines of Star Tropics.
|
|
Back to top |
|
 |
Mattias Gustavsson Mage

Joined: 10 Nov 2007 Posts: 457 Location: Royal Leamington Spa, UK
|
Posted: Thu Mar 17, 2011 8:36 pm Post subject: |
[quote] |
|
tcaudilllg wrote: |
I want to turn the clock back to the single-screen play era, and see what can be done with it. |
I think that is a very good idea - I often get surprised by how good it can be for creativity when you impose restrictions on your own work. Maybe by going single-screen, you'll come up with ideas you would never have got otherwise. _________________ www.mattiasgustavsson.com - My blog
www.rivtind.com - My Fantasy world and isometric RPG engine
www.pixieuniversity.com - Software 2D Game Engine
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Fri Mar 18, 2011 11:00 am Post subject: |
[quote] |
|
Here's that monster script revisited, this time with built-in functions:
Code: |
BEGIN ACTOR Kobold
ON INIT
Self.Energy = 40
Self.HP = 20
Self.EXP = 5
END
ON ACTION
if player is adjacent to Self {
playerIsAdjacent = 1
}
if player is N of Self playerIsN = 1
if player is S of Self playerIsS = 1
if player is E of Self playerIsE = 1
if player is W of Self playerIsW = 1
strikeIsPossible if Self.Energy >= 10
moveIsPossible if Self.Energy >= 1
if playerIsAdjacent && playerIsN && strikeIsPossible {
@Frame = AttackN
playerIsAttacked = "true"
AttackingMonster = @Name
Self.Energy - 10
Idle 10
end
else
if playerIsAdjacentS && playerIsS && strikeIsPossible {
@Frame = AttackS
playerIsAttacked = "true"
AttackingMonster = @Name
Self.Energy - 10
Idle 10
end
else
if playerIsAdjacentE && playerIsE && strikeIsPossible {
@Frame = AttackE
playerIsAttacked = "true"
attackingMonster = @Name
Self.Energy - 10
Idle 10
end
else
if playerIsAdjacentW && playerIsW && strikeIsPossible {
@Frame = AttackW
playerIsAttacked = "true"
attackingMonster = @Name
Self.Energy - 10
Idle 10
end
else
if moveIsPossible {
Move Towards Player
Self.Energy - 1
Idle 4
else
Self.Energy + 1
Idle 4
}
END
ON CONTACT WITH PlayerSword
Self.HP - PlayerSwordPower
if Self.HP < 0 {
PlayerEXP + Self.EXP
HIDE
@Active = 0
}
END
END ACTOR
|
Mattias Gustavsson wrote: | tcaudilllg wrote: |
I want to turn the clock back to the single-screen play era, and see what can be done with it. |
I think that is a very good idea - I often get surprised by how good it can be for creativity when you impose restrictions on your own work. Maybe by going single-screen, you'll come up with ideas you would never have got otherwise. |
I so miss the flavor of the games during the late 80s-early 90s. Games like "Conquest of the Crystal Palace" and "Crystal Warriors"... fantasy kingdoms that had little plot and just... escapism.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Fri Mar 18, 2011 9:00 pm Post subject: |
[quote] |
|
Next I'm going to add two distance functions, one for calculating distance between two tiles and another for reporting all the tiles within a certain distance. Also, a function to return all the actors on a given tile. "For each actor on tile", "For each actor on tiles in group".
|
|
Back to top |
|
 |
 |
Page 1 of 15 |
All times are GMT Goto page 1, 2, 3 ... 13, 14, 15 Next
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|