 |
|
View previous topic - View next topic |
Author |
Message |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Sat Aug 02, 2003 1:14 am Post subject: Some C++ Questions |
[quote] |
|
Well, first, so I know in future, should questions about programming which are not strictly about RPGs go in here, or in the Development forum?
I have some questions about C++; these are questions which aren't covered in any tutorial I've seen, and I couldn't find them answered on the 'net, so I'm asking them here, in hopes that some clever person will help me out.
1. Which of these two methods for passing arguments would you recommend?
Code: | void doSomething ( ArbitraryClass & arg ) {
arg . doSomethingToo ();
}
ArbitraryClass * something = new ArbitraryClass;
doSomething ( (*something) ); |
vs.
Code: | void doSomething ( ArbitraryClass * arg ) {
arg -> doSomethingToo ();
}
ArbitraryClass * something = new ArbitraryClass;
doSomething ( something ); |
2. I've heard that in C++, simple constant variables work like defines (something about being kept in a "symbol table" instead of memory, or something). In the following example,
Code: | void drawSomething ( int x, int y ) {
const int X1 = x;
const int Y1 = y;
const int X2 = x + something . w;
const int Y2 = y + something . h;
something . draw ( X1, Y1, X2, Y2 );
} |
, there's a fair bit of repetition, but it's to my mind simpler and more explicit and I prefer this way of doing. If const ints work like defines, it's perfectly alright, I think, because they're all thrown away when it compiles. If they work like regular variables, occupying memory, then it could slow down the program significantly if I do this all over the place. So do they work the way I hope they do?
Thanks for any light you can shed, I'm really very grateful.
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Sat Aug 02, 2003 3:18 am Post subject: Re: Some C++ Questions |
[quote] |
|
Jihgfed Pumpkinhead wrote: |
1. Which of these two methods for passing arguments would you recommend?
Code: | void doSomething ( ArbitraryClass & arg ) {
arg . doSomethingToo ();
}
ArbitraryClass * something = new ArbitraryClass;
doSomething ( (*something) ); |
vs.
Code: | void doSomething ( ArbitraryClass * arg ) {
arg -> doSomethingToo ();
}
ArbitraryClass * something = new ArbitraryClass;
doSomething ( something ); |
|
First off, manual memory management is a filthy habit from C. Do not ever do manual memory management in C++.
Do this:
Code: | ArbitrayClass something; |
or
Code: | std::auto_ptr<ArbitraryClass> something(new ArbitraryClass); |
or
Code: | boost::shared_ptr<ArbitraryClass> something(new ArbitraryClass); |
or
Code: | MySmartPointerClass<ArbitraryClass> something(new ArbitraryClass); |
Second, I tend to use pointers as arguments in the following cases:
- When I want to allow the caller to pass in null. (There is no such thing as a null reference.)
- When I am transferring ownership of a heap-allocated object.
- When I am passing a C-style array.
- When dealing with pointer-based APIs.
In all other cases, I use references.
Quote: |
2. I've heard that in C++, simple constant variables work like defines (something about being kept in a "symbol table" instead of memory, or something). In the following example,
Code: | void drawSomething ( int x, int y ) {
const int X1 = x;
const int Y1 = y;
const int X2 = x + something . w;
const int Y2 = y + something . h;
something . draw ( X1, Y1, X2, Y2 );
} |
, there's a fair bit of repetition, but it's to my mind simpler and more explicit and I prefer this way of doing. If const ints work like defines, it's perfectly alright, I think, because they're all thrown away when it compiles. If they work like regular variables, occupying memory, then it could slow down the program significantly if I do this all over the place. So do they work the way I hope they do?
|
There are two categories of constants in C++: those that are known at compile time and those that are not. The C++ Standard defines which the difference. Even if you think that a certain constant should be known at compile time, it may not be according to the C++ Standard. I'm not sure of the exact details, but I think it goes something like this:
- Compile-time constants are restricted to built-in types.
- Any numeric or string(?) literal is a compile-time constant.
- The address of a function or a global/static variable is a compile-time constant.
- An expression involving only compile-time constants and no function call or pointer derefernce(?) is itself a compile-time constant.
- Variables declared 'const' may be compile-time constants if they are declared at namespace scope or declared static.
- Compile-time constants can be used to size static arrays or passed as template parameters.
In your example, the values of your constants can clearly not be known at compile time, since they depend on the arguments passed into the function. As far as C++ is concerned, your constants are normal variables with a fancy access restriction. However, the compiler is still allowed to optimize them away if it can do so without changing the semantics of the program. I have no idea if your compiler does this.
Last edited by Rainer Deyke on Sat Aug 02, 2003 4:20 am; edited 1 time in total
|
|
Back to top |
|
 |
ThousandKnives Wandering Minstrel
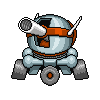
Joined: 17 May 2003 Posts: 147 Location: Boston
|
Posted: Sat Aug 02, 2003 3:32 am Post subject: |
[quote] |
|
I dont really what Rainer is talking about, since I'm a C man myself and happen to be fairly used to my "filthy habits" hehe. Whats this about "new" though? Don't you mean "malloc"? jk
I do know this: Passing a pointer to an object (second method) is almost always faster and more effecient than passing the object itself, since the object itself is almost always larger than its address. Passing the object itself creates a duplicate local instance, I believe. So, you are much better off just passing a pointer and working on the original thing itself through your pointer.
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Sat Aug 02, 2003 4:24 am Post subject: |
[quote] |
|
Jihgfed's first method was passing by reference, which is as fast as passing by pointer. (References are a C++ feature that is not found in C.) You're thinking about passing by value.
|
|
Back to top |
|
 |
ThousandKnives Wandering Minstrel
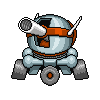
Joined: 17 May 2003 Posts: 147 Location: Boston
|
Posted: Sat Aug 02, 2003 5:09 am Post subject: |
[quote] |
|
Rainer Deyke wrote: | Jihgfed's first method was passing by reference, which is as fast as passing by pointer. (References are a C++ feature that is not found in C.) You're thinking about passing by value. |
Ah... oopo
Me not speak C++ goodly? That's unpossible!
|
|
Back to top |
|
 |
akOOma Wandering Minstrel

Joined: 20 Jun 2002 Posts: 113 Location: Germany
|
Posted: Sat Aug 02, 2003 6:18 am Post subject: |
[quote] |
|
ThousandKnives wrote: | I dont really what Rainer is talking about, since I'm a C man myself and happen to be fairly used to my "filthy habits" hehe. Whats this about "new" though? Don't you mean "malloc"? jk |
It's the same thing, but you use "malloc" in C, and "new" in C++. That's the one and only difference. _________________ Keep on codin'
-----------------
-----------------
Just another post to increase my rank...
|
|
Back to top |
|
 |
ThousandKnives Wandering Minstrel
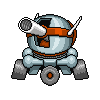
Joined: 17 May 2003 Posts: 147 Location: Boston
|
Posted: Sat Aug 02, 2003 7:45 am Post subject: |
[quote] |
|
Yeah, I know. Thats why I put the lame "jk" after it. It's my own fault for being so irc though.
I think there are some difference between malloc and new and free and delete, as well. I can't remember much from my object-oriented programming course. My professor was too busy having orgasms over how great Java was that he had trouble focusing on actually teaching C++. Funny thing is, all these years later you still don't see any serious software projects made using Java, despite the passionate affair CompSci departments had and continue to have with it.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
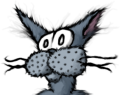
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sat Aug 02, 2003 8:53 am Post subject: |
[quote] |
|
ThousandKnives wrote: | Yeah, I know. Thats why I put the lame "jk" after it. It's my own fault for being so irc though.
I think there are some difference between malloc and new and free and delete, as well. I can't remember much from my object-oriented programming course. My professor was too busy having orgasms over how great Java was that he had trouble focusing on actually teaching C++. Funny thing is, all these years later you still don't see any serious software projects made using Java, despite the passionate affair CompSci departments had and continue to have with it. |
Heh. That's quite curious; my CS department's language of choice -- at least, the language that is taught to all incoming students and that is used as the de facto standard in most courses -- is C++. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
valderman Mage
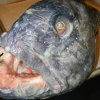
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Sat Aug 02, 2003 11:50 am Post subject: |
[quote] |
|
Sorry Rainer, but your onion about memory management is wrong (gamedev joke - i r teh funay!). Using new and delete instead of auto_ptr is perfectly fine, at least when you want to allocate something like 4096 bytes of memory, and I don't suppose an auto_ptr would be very handy in a linked list. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Sat Aug 02, 2003 2:00 pm Post subject: |
[quote] |
|
Valderman wrote: | Sorry Rainer, but your onion about memory management is wrong (gamedev joke - i r teh funay!). Using new and delete instead of auto_ptr is perfectly fine, at least when you want to allocate something like 4096 bytes of memory, and I don't suppose an auto_ptr would be very handy in a linked list. |
'std::auto_ptr' still requires 'new', and 'delete' definitely has its place. However, that place is in destructors. Preferably the destructor of 'std::auto_ptr' or another smart pointer class, but failing that any other destructor will do. Calling 'delete' outside of destructors can cause memory leaks in the presence of exceptions, and it's far too easy to forget to 'delete' something.
I'm not sure what you mean by "in a linked list". If you mean storing 'std::auto_ptr' values in a 'std::list', then yes, it can't be done. 'std::auto_ptr' doesn't play nicely with containers, so this is one of the cases where you have to call 'delete' yourself (in a destructor!) or use a more powerful smart pointer class like 'boost::shared_ptr'.
If you mean implementing the links in a linked list, I don't see why not.
Code: | class node {
std::auto_ptr<node> next;
node *prev; // optional
// other data here...
}; |
You have to be careful when adding and removing nodes that you don't prematurely delete anything, but you have to be careful when writing linked list code anyway.
|
|
Back to top |
|
 |
BigManJones Scholar
Joined: 22 Mar 2003 Posts: 196
|
Posted: Sat Aug 02, 2003 2:48 pm Post subject: |
[quote] |
|
I've taken CS courses at three different universities over the years with three different languages used; Pascal, C, and C++. I self taught myself Java. I think the answer to the origional question is "which ever way you prefer". Personally I like Rainiers method of using references unless you have to use pointers. C++ is very frustrating to me because of the absurd syntax you see sometimes and the fact that compilers STILL cannot compile source that mixes some of the features legally (like returning a private member struct from a private member method)
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Sat Aug 02, 2003 3:04 pm Post subject: |
[quote] |
|
I never had any trouble with returning private structs from member functions. Did you fully qualify the return type?
Code: | class outer {
private:
class inner {};
inner mem_fn();
};
outer::inner outer::mem_fn()
{
inner i;
return i;
} |
Of course there are plenty of other C++ features that are not widely supported.
|
|
Back to top |
|
 |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Sun Aug 03, 2003 8:17 pm Post subject: Thanks for the Programming Help |
[quote] |
|
Thanks a lot, Rainer, that's a lot of good advice there. Just what I was looking for. I've heard about these "smart pointers" before; I'll see if I can't find more information on them.
So again, thanks a lot for all the help. I'm very appreciative.
|
|
Back to top |
|
 |
BigManJones Scholar
Joined: 22 Mar 2003 Posts: 196
|
Posted: Sun Aug 03, 2003 8:41 pm Post subject: |
[quote] |
|
EDIT 3: Nevermind I'm dumb. No, I didn't fully qualify the return value.
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|