 |
|
View previous topic - View next topic |
Author |
Message |
DeveloperX 202192397
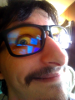
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Oct 28, 2003 10:39 pm Post subject: Using SCREEN 13 BSAVEd images in SCREEN 12 |
[quote] |
|
Do you use Pixel Plus 256 or any other SCREEN 13
Program for making graphics for QB games?
Wanted that 640x480 SCREEN 12 resolution, but couldn't
find a tool for drawing graphics in screen 12?
Well, I'm about to show you how to use PP256 for SCREEN 12
graphics! :)
NOTE: this only works with single-image .PUT files,
not the multi/animated frame .PUT files.
Just go draw something in PP256, save it.
Now fire up QB, and type this little program in:
Code: |
CLS
SCREEN 12
W = 15 ' 15 cause we start counting at 0
H = 15 ' 15 cause we start counting at 0
' for square images, the formula for array bytes is:
' bytes& = width * height \ 2 + 2
' 16*16\2+2 = 256\2+2 = 128+2 = 130
BYTES& = 130
DIM IMG%(BYTES&)
Filename$ = "TEST.PUT"
'set the default memory segment to the begining of the array:
DEF SEG = VARSEG(IMG%(0))
'use BLOAD to load the file:
BLOAD Filename$, VARPTR(IMG%(0))
'loop through the width of the image
FOR x = 0 TO W
' loop through the height of the image
FOR y = 0 TO H
' grab the pixel color from the array:
' NOTE the W+1 because we want the actual size, not the zero-based size.
PixelColor = PEEK(4& + x + y * W&+1)
'draw the pixels
PSET (x, y), PixelColor
NEXT
NEXT
'restore default segment:
DEF SEG
' use GET to grab the image back into the array
' using the SCREEN 12 format:
GET (0, 0)-(W, H), IMG%
'draw it to be sure it worked:
PUT (320, 240), IMG%, PSET
|
I've placed the code into an easy to use SUB for you:
Code: |
CLS: SCREEN 12:DIM BALL%(130)
BLOAD12 "ball.put", BALL%()
PUT(50, 50), BALL%, PSET
SUB BLOADS12 (Filename$, IMG%())
DEF SEG = VARSEG(IMG%(0))
BLOAD Filename$, VARPTR(IMG%(0))
FOR x = 0 TO 15
FOR y = 0 TO 15
PixelColor = PEEK(4& + x + y * 16)
PSET (x, y), PixelColor
NEXT
NEXT
DEF SEG
GET (0, 0)-(15, 15), IMG%
END SUB
|
Questions? Email me. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
Last edited by DeveloperX on Thu Nov 18, 2004 11:24 pm; edited 1 time in total
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
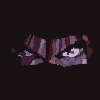
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
|
Back to top |
|
 |
DeveloperX 202192397
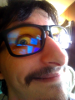
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
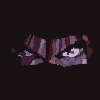
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Wed Oct 29, 2003 2:47 am Post subject: |
[quote] |
|
Oh wait, yours are in screen 12, aren't they?
Neeeeeeeeeeeeeeeeeeeevermind.
|
|
Back to top |
|
 |
akOOma Wandering Minstrel

Joined: 20 Jun 2002 Posts: 113 Location: Germany
|
Posted: Wed Oct 29, 2003 11:31 am Post subject: |
[quote] |
|
There's a little problem. PP256 pics are stored in Screen 13 format (8 Bit colors = 256 colors). But Screen 12 only features 4 Bite Colors (16 colors). So you can only use 16 colors in PP256 and must change the saved file to another format. _________________ Keep on codin'
-----------------
-----------------
Just another post to increase my rank...
|
|
Back to top |
|
 |
DeveloperX 202192397
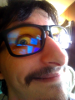
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Thu Oct 30, 2003 5:40 am Post subject: |
[quote] |
|
akOOma wrote: | There's a little problem. PP256 pics are stored in Screen 13 format (8 Bit colors = 256 colors). But Screen 12 only features 4 Bite Colors (16 colors). So you can only use 16 colors in PP256 and must change the saved file to another format. |
true, well.. somewhat.
It IS true that mode12h only supports 16 colors..at one time.
I use some little tricks to get all 256 colors, but I only use 16 at a time on each screen, and from the looks of FFIV.. 16 colors isn't anything to shake a stick at.
Final Fantasy 5 only used 16 colors at a time, there were 256 unique colors in the virtual palette, but only 16 could be displayed at one time.
anyway...
I'm trying to devise a way to get into modeX using only qb and the out,inp,poke,peek commands.
no asm.
..anyway... _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
DeveloperX 202192397
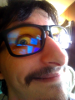
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
|
Back to top |
|
 |
akOOma Wandering Minstrel

Joined: 20 Jun 2002 Posts: 113 Location: Germany
|
Posted: Fri Oct 31, 2003 5:03 pm Post subject: |
[quote] |
|
Try this:
(It's a ModeX scroller)
Code: |
'===========================================================================
' Subject: MODE-X SCROLL Date: Unknown Date
' Author: Jason Plackey Code: QB, QBasic, PDS
' Origin: Prodigy Packet: GRAPHICS.ABC
'===========================================================================
' Regarding the example code... Change the delay% value to
'accommodate your CPU's speed. The TIMER function is
'relatively inaccurate when dealing with fractions of a
'second, and I had insufficient space to include my own timer
'routine.
'
' If you have any questions, feel free to ask.
'Happy coding! Jason Plackey
DECLARE SUB ModeX ()
DECLARE SUB OutPort (PortAddr&, vh%, vl%)
DECLARE SUB Standard ()
DECLARE SUB SetVOffset (Offset&)
SCREEN 13: DEF SEG = &HA000: CALL ModeX
FOR count% = 0 TO 63
redval& = count%
greenval& = 256 * count%
blueval& = 65536 * count%
rgbval& = blueval& + greenval& + redval&
PALETTE count%, redval&
PALETTE count% + 64, greenval&
PALETTE count% + 128, blueval&
PALETTE count% + 192, rgbval&
NEXT count%
FOR Offset& = 0 TO 15999
rgbbase% = Offset& MOD 64
POKE Offset&, rgbbase%
POKE Offset& + 16000, rgbbase% + 64
POKE Offset& + 32000, rgbbase% + 128
POKE Offset& + 48000, rgbbase% + 192
NEXT Offset&
Offset& = 0: OffsetInc% = 80: Frame% = 0
DO
Offset& = Offset& + OffsetInc%
CALL SetVOffset(Offset&): WAIT &H3DA, 8
Frame% = Frame% + 1
IF Frame% = 99 THEN OffsetInc% = 81
IF Frame% = 199 THEN OffsetInc% = 79
IF Frame% = 299 THEN OffsetInc% = 80
IF Frame% = 499 THEN OffsetInc% = -80
IF Frame% = 599 THEN OffsetInc% = -79
IF Frame% = 699 THEN OffsetInc% = -81
IF Frame% = 799 THEN OffsetInc% = -80
IF Frame% = 899 THEN
CLS : PALETTE
CALL SetVOffset(0): CALL Standard
END
END IF
FOR delay% = 1 TO 1000: NEXT delay%
LOOP
SUB ModeX
CALL OutPort(&H3C4, 6, 4): CLS
CALL OutPort(&H3D4, 227, 23)
CALL OutPort(&H3D4, 0, 20)
CALL OutPort(&H3C4, 15, 2)
END SUB
SUB OutPort (PortAddr&, vh%, vl%)
OUT PortAddr&, vl%: OUT PortAddr& + 1, vh%
END SUB
SUB SetVOffset (Offset&)
V& = Offset&
CALL OutPort(&H3D4, V& \ 256, 12)
CALL OutPort(&H3D4, V& AND 255, 13)
END SUB
SUB Standard
CALL OutPort(&H3C4, 14, 4)
CALL OutPort(&H3D4, 163, &H17)
CALL OutPort(&H3D4, 64, &H14)
CALL OutPort(&H3C4, 15, &H2)
END SUB
|
_________________ Keep on codin'
-----------------
-----------------
Just another post to increase my rank...
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|