View previous topic - View next topic |
Author |
Message |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Wed Feb 08, 2006 7:27 am Post subject: |
[quote] |
|
Nephilim wrote: | If it's any consolation, Director now understands Javascript. |
Hsssst! You'll draw He-Whose-Name-Shall-Not-Be-Spoken back here! :o _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Wed Feb 08, 2006 11:12 pm Post subject: |
[quote] |
|
Are there any good scripting tutorials that you guys know about?
I mean to actually implement scripts in the game.
I quite honestly have no idea where to even begin with that, so if you have anything for me let me know.
No big deal though. I'm sure I'll figure it out sooner or later,
Jason
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Wed Feb 08, 2006 11:48 pm Post subject: |
[quote] |
|
Eh, it's not all that hard. You just need to parse some strings.
Endless Saga's maps are binary. They contain the environment data (the map, polygone per polygone), the object data (reusable models that are only stored once and scaled/rotated/translated throughout the scene, like trees and rocks), and finally the event data. Event data includes scripts, which are associted with sprites or map events (like startup.) I keep a text version of my maps so I can quickly modify things by hand, and wrote a little program to convert them to binary for in-game use.
A typical script may look a little something like this (none of these commands are actual commands, but it should give you an idea how I do things...)
Code: | ::StartUp
PlayMusic 0x04 #Chapter 1's 'Large village' theme.
Encounters 0x00 #No encounters for this map!
End
::Sprite01 #The old man by the gates
FacePlayer
SetVar 01 Random 1, 4
IfVar 01 Eq 1
Message "The government is out to get you!"
EndIf
IfVar 01 Eq 2
Message "I am a fake sprite!"
EndIf
IfVar 01 Eq 3
Message "Next message is going to be a little more complex."
EndIf
IfVar 01 Eq 4
IfVar 02 Eq 1
Message "I already gave you a sandwich. Go bug someone else."
Otherwise
Message "Here, have a sandwich."
SetVar 02 Eq 1
EndIf
EndIf
End
::Sprite02 #The hungry hobo.
SetFrame 0x08 ;Hands outstretched.
FacePlayer
IfVar 02 Eq 1
Message "A sandwich!"
Message "[The hobo stole your 'wich, dude!]"
SetVar 02 Eq 0
Otherwise
Message "Got any food?"
EndIf
End |
Since I don't want players mucking about with the script, I don't store it as text in my binary, "compiled" map. My conversion utility goes through every script and replaces reserved keywords by a special code (don't just blindly replace every instance of the keyword; if "End" is a reserved keyword and a character says "Well guys, looks like this is the end!", you're gonna run into problems. ;) ) This comes out something a little like this... (I've seperated lines with a | character and left plain text as plain text to make it easier to see what maps to what.)
Code: | ::StartUp
46 04 | 47 00 | FF
::Sprite01 #The old man by the gates
20 | 0A 01 0F 01 04 | 10 01 CC 01 | 20 "The government is out to get you!" | 11 | 10 01 CC 02 | 20 "I am a fake sprite!" | 11 | 10 01 CC 03 | 20 "Next message is going to be a little more complex." | 11 | 10 01 CC 04 | 10 02 CC 01 | 20 "I already gave you a sandwich. Go bug someone else." | 12 | 20 "Here, have a sandwich." | 0A 02 CC 01 | 11 | 11 | FF
::Sprite02 #The hungry hobo.
22 08 | 20 | 10 02 CC 01 | 20 "A sandwich!" | 20 "[The hobo stole your 'wich, dude!]" | 0A 02 CC 00 | 12 | 20 "Got any food?" | 11 | FF |
When I load these in the actual game, I have an array which maps each code to a function (function pointers; you can just do a big switch() statement too, if you feel uncomfortable with function pointers). For instance, 0x46 would be the command which called the "SetMusic" function, which in turn reads a byte from the script and uses that as the music to set.
You don't have to work with binary at all. I do it because I don't want users fiddling with my scripts and because I want to cut down on space (ShowMessage is 11 bytes; 0x22 is 1) due to the large amount of maps and events in the game. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Wed Feb 08, 2006 11:55 pm Post subject: |
[quote] |
|
So are scripts basically just string files that you can input into your game? Like a map.txt file that loads in the tiles?
If that's so, then my only question is: how can you use the script constantly? Do I just have to continuously input it into my game each loop?
Thanks,
Jason
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
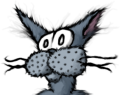
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Thu Feb 09, 2006 12:03 am Post subject: |
[quote] |
|
You can also write your own interpreter that is slightly more advanced, and OO, via a variety of routes; probably the easiest is with a parser and lexer generator, of which bison and flex are two good exemplars. You can also throw something together using either the State Pattern or the Interpreter Pattern, either of which is a good, viable OO alternative to some sort of silly look-up table. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Thu Feb 09, 2006 12:17 am Post subject: |
[quote] |
|
The way I listed isn't the only way; it's just one of the easier, if more basic, ways to handle scripts. Like LD said, there are some much more flexible and powerful ways to do it.
Just call your script when you need it. If you're talking to an NPC, go look up its script and call the appropriate code. In the meantime, just keep it tucked away in memory... _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Thu Feb 09, 2006 12:20 am Post subject: |
[quote] |
|
RuneLancer wrote: | The way I listed isn't the only way; it's just one of the easier, if more basic, ways to handle scripts. Like LD said, there are some much more flexible and powerful ways to do it.
Just call your script when you need it. If you're talking to an NPC, go look up its script and call the appropriate code. In the meantime, just keep it tucked away in memory... |
Ah, that makes sense.
As for now though I'm going to implement movement and eventually the tile engine. With any luck I'll be drawing tiles tonight!
Jason
|
|
Back to top |
|
 |
Nephilim Mage

Joined: 20 Jun 2002 Posts: 414
|
Posted: Thu Feb 09, 2006 2:54 am Post subject: |
[quote] |
|
RuneLancer wrote: | You don't have to work with binary at all. I do it because I don't want users fiddling with my scripts and because I want to cut down on space (ShowMessage is 11 bytes; 0x22 is 1) due to the large amount of maps and events in the game. |
Very true - my map format is rather verbose compared to RL's (even without the comments - heh). If you have a small scale RPG, though, it's probably not that big of a deal, because, after all, we're talking about tiny little text files here. I'd recommend just going with a simple string format for now, and converting it to a binary format later only if you find that the game data is getting burdensome. They're easier to deal with because you can just pop open a text editor to make changes and experiment with stuff - you don't need to write a custom editor. You can always refactor your map loading and saving code to create binary files later after you're settled on a final format.
I did something similar with Sacraments. During development, the map files and cutscene files were separate text files in a directory that I could edit with a text editor and see changes on-the-fly in the game if necessary. Then, when I went to publish, I had a little utility that imported all the maps and scenes in the directory into the game's internal database, which compresses the data and rolls it into the actual executable file, so that there would be no support files lying around for the end user to have to deal with. _________________ Visit the Sacraments web site to play the game and read articles about its development.
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Thu Feb 09, 2006 3:14 am Post subject: |
[quote] |
|
Just an update on what I got so far:
Engine, Input, main, Player, Timer, and Graphics classes are done.
Basically main initializes Engine, which calls everything.
The graphics class handles text, drawing and loading of bitmaps, the works.
Input and player are pretty much self explanatory, and the Timer class handles the Fps counters and stuff.
By the way I got the smooth movement to work. Basically what I do is increment a counter to find the frames for that second. Each loop I update the m_framesPerSecond variable, to give to the player so he can move the desired distance.
And I gotta hand it to you guys, that unit scale based movement is perfect. I've even tested it while overloading my machine (:P)
I can move the character around, load his bitmaps, etc. with the framework of Handling GameStates and Directions.
Oh and I got the little fps counter in the corner (for some reason before this project I could never get SDL's stupid text functions to work).
Not much, but not bad for about 4-5 hours of work. Plus I'm thinking as I go along, and have no design document to look off of.
Now I'm going to work on the map. I just have to decide how I want to scroll it (I want it to scroll).
What I'm thinking of doing is having maps the size of roughly the screen, and have each map have coordinates.
So everytime I move the player, I increment the current pos on the map, so if the player goes over halfway between one map and the next, the other map is loaded.
Or should I have larger maps?
Tell me what you think. My current fps is around 400 on my computer, without hardware acceleration (well it's 2D anyways duh), so I'm hoping this could turn out real good :)
God this is exciting, lol
Jason
|
|
Back to top |
|
 |
Nephilim Mage

Joined: 20 Jun 2002 Posts: 414
|
Posted: Thu Feb 09, 2006 3:49 am Post subject: |
[quote] |
|
That's awesome, dude. Congrats on your early success. _________________ Visit the Sacraments web site to play the game and read articles about its development.
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Thu Feb 09, 2006 4:40 am Post subject: |
[quote] |
|
Ok so I got another question. I just finished polishing up some thing, and now I'm ready for the tileEngine.
I'm trying to think of the best way to do it. I'm currently saving tile numbers in a .txt file (currently I'm only using 0 for one tile so I can get a basis for it), and want to import them into my game.
-------------------------------------------------------
Ok, after spending about 20 minutes trying to figure it out and write the code, I decided to stop.
What I was thinking about doing was having an array of strings holding all the values of the tiles I want to load.
Then I'd make a loop, pushing the loaded files onto a vector pile like so:
[code]vector<SDL_Surface*> m_tiles;
string newArray = .....
for (int i = 0; i < whatever; i++)
{
m_tiles.push_back(m_graphics->LoadBitmap(newArray[i]));
}
to push the different tiles onto the stack, using the indexes of the vector to signify their numbers on the actual map.
(I would have also already copied the map numbers into a multidimensional array)
Then when it's blitting time, I'd go through the map and blit each tile to the screen according to their location in the m_tiles vector.
The problem with this is that I'd have more Surfaces than I woul want, which takes up memory.
My second option would be to load one big bitmap and then somehow blit the images on the screen according to their location within the bitmap (haven't figured that out yet)
Do you guys have any other suggestions for me on what I could do?
Thanks,
Jason
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
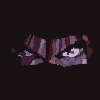
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Thu Feb 09, 2006 4:48 am Post subject: |
[quote] |
|
We always have tilesets in one or so large bitmaps (I use png for transparency, but you'll learn about that when you do 3D), and basically follow a set of rules.
Say you have 16x16 tiles. You place all tiles adjacent to each other, but you don't want the bitmap to be too lopsided (all tiles in one row), so you conform to something like a size of 256x256, so 16x16 tiles. How do you get the source rect with just an index number? Well first you take the size of the bitmap and divide by tilesize so you know how many tiles are in a row.
Code: | tilesPerRow = bitmapWidth / tileSize;
y = (index / tilesPerRow)*tileSize
x = (index % tilesPerRow)*tileSize;
sourceRect = {x, y, x+tileSize-1, y+tileSize-1} |
I think that would work. One second... ya I think so.
Last edited by Ninkazu on Thu Feb 09, 2006 5:20 am; edited 2 times in total
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Thu Feb 09, 2006 4:51 am Post subject: |
[quote] |
|
Why not simply stick all of your tiles in a single bitmap and load it into one surface?
What I used to do when I had 2D games was create a 16x16 grid of tiles (256 tiles) for any given map and store the tiles as a byte (number from 0-255) in my map file. I'd load the map into an array (1D or 2D, whichever you're most comfortable working with but it's essentially the same thing) and for every visible tile, I'd blit the tile to the screen.
To obtain the position of the tile, I'd do this... (assuming 32x32 tiles)
X = (TileID mod 16) x 32 // (ID mod nTilesX) x width
Y = int(TileID / 16) x 32 // int(ID / nTilesY) x height
Should be a piece of cake from that point on. You can also build a lookup table and just obtain the positions from it by passing the ID. Whatever works for you, really. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Thu Feb 09, 2006 9:06 pm Post subject: |
[quote] |
|
That's a good idea.
What I'm thinking of doing is messing with SDL to make some sort of huge surface (SDL_RGBSurface I think it's called) and make bitmaps according to what I want.
That way, I could easily make a scrolling background because all I'd have to do is update the position of the map to blit.
The only thing then would be to figure out how to keep items on the map. I think I might just have a struct for each item to store its attributes, position, etc.
Jason
|
|
Back to top |
|
 |
zenogais Slightly Deformed Faerie Princess
Joined: 10 Jan 2006 Posts: 34
|
Posted: Thu Feb 09, 2006 11:55 pm Post subject: |
[quote] |
|
Gardon: For smooth scrolling, instead of doing raw surface stuff, you might want to look into clipping rectangles. They'd probably be much easier to use for a surface blitted with map tiles. Check here for more info.
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|