View previous topic - View next topic |
Author |
Message |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sun Aug 15, 2004 4:55 pm Post subject: |
[quote] |
|
The benefit of script is that there is a minimum of overhead. The effort is to model the system first; perfect it; and port it over here, there, wherever.
Earlier there was some discussion about using cookies to transfer data between a server-side script and a JS webclient. This code does that.
Code: |
// |*| InstantMessengerJS - a basic instant messenger client for
// Javascript/CGI.
//
//
// (+) Author
//
// -=> Anthony Caudill, August 15 2004
//
//
// (+) Message Structure (Sender)
//
// -=> [Author]: [Body]
//
//
// (+) Message Structure (Recipient)
//
// -=> [Author] ([Date] [Time]): [Body]
//
//
// (+) Concept
//
// -=> Use cookies as means of information exchange between a
// clienside Javascript and a server-side CGI program or
// script, such as that written in C, PERL, PHP, etc.
//
//
//
//
function CreateIM (IM) {
// |*| The CreateIM Function
//
//
// |!| The CreateIM function is an IM object prototype. The IM
// object has two properies Author and Body.
//
IM.Author = "";
IM.Body = "";
}
function ResetTextNode (Destination, Source) {
// |*| The ResetTextNode Function
//
//
// |!| The ModifyTextNode function replaces the source of a
// text node with new source.
//
// (+) Craft a new text node with the W3C DOM createTextNode function.
// method. Employ the replaceNode DOM method upon the Destination
// node to reset its source to that of the Source.
//
var Destination = Destination.childNodes(0);
Destination.replaceNode (document.createTextNode (Source));
}
function WriteNodeToCookie (Destination, Source) {
// |*| The WriteNodeToCookie Function
//
//
// |!| The WriteNodeToCookie function is an instance of the
// WriteStringToCookie function that writes a single text node
// to a cookie.
//
//
// (+) Call the WriteStringToCookie function with the first child
// node of the source acting as the source string.
//
WriteStringToCookie (Destination, Source.childNodes(0));
}
function WriteStringToCookie (Destination, Source) {
// |*| The WriteStringToCookie Function
//
//
// |!| The WriteStringToCookie function writes a source string
// to a cookie. If the source string is too large for the
// cookie, the browser agent may truncate it.
//
//
// (+) Create a cookie header by concatenating the Destination
// with an "=" character. Store the cookie header in an
// Auxillary variable.
//
//
// (+) While the SourceIndex is less than the Source.length....
//
// -=> Amend the Auxillary by concatenating it with the Source
// vector entry at the SourceIndex.
//
//
// (+) Reset the document.cookie to the fusion of the Auxillary and
// the cookie terminator character ";".
//
var Auxillary = Destination + "=";
for (SourceIndex = 0;
SourceIndex < Source.length;
SourceIndex++) {
Auxillary = Auxillary + Source.nodeValue.charAt (SourceIndex);
}
document.cookie = Auxillary + ";";
}
function ReadStringFromCookie (Source) {
// |*| The ReadStringFromCookie Function
//
//
// |!| The ReadStringFromCookie function reads a single cookie
// into a string.
//
//
// (+) While the Auxillary is less than the length of the cookie
// source and the document.cookie vector entry at the
// cookie's index within the cookie source combined with the
// Auxillary is not equal to the ";" cookie terminator
// character....
//
//
// (+) Combine the Destination with the document.cookie vector entry
// at the index of the cookie in the cookie source combined with
// the Auxillary.
//
var Destination = "";
for (Auxillary = 0;
Auxillary <= document.cookie.length
&&
!(document.cookie.substring(document.cookie.indexOf(Source) + Auxillary,
document.cookie.indexOf(Source) + Auxillary + 1) == ";");
Auxillary++) {
Destination =
Destination
+ (document.cookie.charAt(document.cookie.indexOf(Source)
+ Auxillary));
}
return Destination;
}
function ReceiveIM (Source) {
// |*| The ReceiveIM Function
//
//
// |!| The ReceiveIM function integrates the date and time into an
// incoming instant message.
//
//
// (+) Concatenate the date and time into a single Auxillary string.
//
//
// (+) Concatenate the Source string fragment beginning at close of
// of the IM header with the " (" string, the Auxillary, the ")"
// character, and the message body.
//
Auxillary = new Date();
Auxillary = Auxillary.getDate() + Auxillary.getTime();
var Destination =
Source.substring (0, Source.indexOf(":"))
+ " ("
+ Auxillary
+ ")"
+ Source.substring (Source.indexOf(":"));
return Destination;
}
function WriteIMToString (Source) {
// |*| The WriteIMToString Function
//
//
// |!| The WriteIMToString function assembles an instant message
// from the properties of an IM object.
//
//
// (+) Concatenate the SourceName property, the ": " string, and
// the Source.Body property into a single Destination string.
//
var Destination =
Source.Name
+ ": "
+ Source.Body;
return Destination;
}
|
I've tested it throughly. It's perfect. There is one problem, but it's the fault of the makers of the cookie data structure, not mine. That problem is semicolons. Semicolons terminate the cookie. A workaround must be devised if binary data is to be transferred through the cookie, or even if semicolons are to be used in IMs.
One strategy would be to make two cookies and get all of the data between the start of the first cookie and the beginning of the second. Then the semicolon wouldn't be an issue. The question to my mind is, what in the client's mind is the end of a cookie, and what qualifies as the beginning of a new one? I don't know either for sure yet, but they are on my mind....
Links:
http://unitedinfinity.atspace.com/Projects/JS/CellWalker.js
http://unitedinfinity.atspace.com/Projects/JS/TimerKernalJS.js
http://unitedinfinity.atspace.com/Projects/JS/IMJS.js
http://unitedinfinity.atspace.com/Projects/DHTML/IMJS/IMClient8152004.html
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Tue Aug 17, 2004 9:12 am Post subject: |
[quote] |
|
I completed an animation algorithm for tile-based movement scenarios.
Code: |
function TranslateSprite (Sprite) {
// |*| The TranslateSprite Function
//
//
// |!| The TranslateSprite function translates a sprite over
// the display pixel by pixel over an axis, until its
// new position reflects the union of its old coordinate
// on the axis with its applied velocity of movement.
//
//
// (+) If the Sprite.Movement.Index is less than the
// Sprite.Movement.Velocity....
//
// -=> If the quotient of the Sprite.Movement.Velocity
// divided by the Sprite.Movement.Index is more than
// or equal to the quotient of the Sprite.Frame.Limit
// divided by the Sprite.Frame.Index....
//
// -=> Increase the Sprite.Frame.index by 1.
//
// -=> Increase the Sprite.Movement.Index by the
// Sprite.Movement.Moment.
//
// -=> Increase the Sprite.Axis by the Sprite.Movement.Moment.
//
// -=> Set the Sprite.Translation.Flag.
//
Sprite.Frame.Index = 1;
if (Sprite.Movement.Index <= Sprite.Movement.Velocity) {
if ((Sprite.Movement.Velocity / Sprite.Movement.Index)
>=
(Sprite.Frame.Limit / Sprite.Frame.Index)) {
Sprite.Frame.index = Sprite.Frame.Index + 1;
}
Sprite.Movement.Index =
Sprite.Movement.Index + Sprite.Movement.Moment;
Sprite.Axis = Sprite.Axis + Sprite.Movement.Moment;
Sprite.Translation.Flag = 1;
}
}
|
Next are the script interpreter and tile layers. As I see it, if the engine will be driven with CGI, then wouldn't it be possible to make the map engine compatible with Tiled?
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Tue Aug 17, 2004 1:47 pm Post subject: |
[quote] |
|
What's CGI?
Btw, do you find it convenient to paste all that code in here? Maybe it would be better to start building a prototype on a website somewhere. Although I'm not interested in a JavaScript implementation, I could still set you (and any people that want to help out) up an rpgdx.net subdomain to host it.
|
|
Back to top |
|
 |
Aptyp Egg-Sucking Troll Humper
Joined: 09 Jun 2004 Posts: 36
|
Posted: Tue Aug 17, 2004 6:33 pm Post subject: |
[quote] |
|
CGI is Common Gateway Interface. I think by that he means server-side scripts.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Fri Aug 20, 2004 9:55 pm Post subject: |
[quote] |
|
I put the code in as a means of communication and education. The code is unimportant, the alogorithm it represents--and understanding of it--are vital.
The animation algorithm is excellent, I think. Maybe I should submit it to the articles section. It's a very unusual structure, kinda hard to figure out by oneself. Extremely powerful though.
There's a lot on the 'Net about programming for specific cases, but very little analysis of the algorithmic structure of indefinitely extensible source code except at the academia levels. (and the bar for understanding anything written for that level is specialized and high) I'd like to change that.
By making key algorithms better known and the techniques of their implementation understood, we can quickly lay the groundwork for an era when good games are the rule, not the exception.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sat Aug 21, 2004 4:21 pm Post subject: |
[quote] |
|
Wasn't there a wiki for the virtual convention project? :?
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Sun Aug 22, 2004 12:53 pm Post subject: |
[quote] |
|
There's the RPGDX Wiki you are free to add pages about the convention project to.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Mon Aug 23, 2004 9:31 pm Post subject: |
[quote] |
|
It doesn't preserve my code without corrupting the entire wiki.... :(
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Mon Aug 23, 2004 10:13 pm Post subject: |
[quote] |
|
I noticed. Also, I reorganized the pages a bit, I hope you don't mind.
The Wiki doesn't seem the best place for code... to make it monospace it needs at least one space before each line. Further more, I don't know how to prevent it from using Wiki links easily... In the long run, we should probably switch to a better Wiki system, that I could also integrate with the user database of phpBB...
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Tue Aug 24, 2004 11:13 pm Post subject: |
[quote] |
|
Good idea. I made a lot of progress on the scripting engine today. It should be a little less confusing now to any who care to look at it.
I'm trying to design a dynamic object oriented architecture. Such a thing may or may not have been done before, I'm not sure. By "dynamic", I am referring to virtual machine nature of the engine, complete with a "code segment" that is user controlled. It's like the CS register on an Intel processor, but without an OS to regulate access to it. A line of script can literally rewrite itself.
By making all script elements dynamic in nature, it is possible to generate script lines from information that is completely external to the script. Dynamic script generation implies a whole new world of user interface design that my imagination only touches upon.
The obstacle before me is the object oriented model itself, and the extremely complex methods that are needed to negotiate it without error. I've been working on it a while now. It's just so complex, that it's difficult for my brain to keep up with. :\ When I do figure it out, then I can integrate my new "tensor" mechanic into the script engine, a variable whose value is intrinsically linked to another value, such that when one value changes the other changes along with it. An example would be mapping movement along a 2D plane to the surface of a sphere. Variables representing the position of the moving object on the plane would figure into every assignment of value to the position variables of the sphere. ...It's an ultimately powerful concept, I think.
Is this a good idea? Are there problems that I've not yet seen?
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
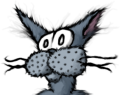
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Wed Aug 25, 2004 6:34 am Post subject: |
[quote] |
|
Dynamic code generation is, by and large, a bad idea. Not only is such a system complex to implement and design for, but is horribly difficult to debug. Any good design can circumvent the necessity for such a poor concept.
And what it sounds like you are describing is pointers. Now, it may just be your wonky usage of CS catch-phrases, but that's pretty much the gist that I get when I hear of "a variable whose value is intrinsically linked to another value". _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
valderman Mage
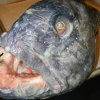
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Wed Aug 25, 2004 1:02 pm Post subject: |
[quote] |
|
I can't really see the need for self-modifying code in a scripting language for a game, unless you mean to have a really complex AI controlled by the scripting language. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Wed Aug 25, 2004 5:09 pm Post subject: |
[quote] |
|
That -COULD- be the case, although it isn't a necessity.
An intelligent functional algorithm.
A1) Draw up a list of behavior patterns. Store
the patterns in a vector. Assign each of
these patterns the same value. Call this
value the behavioral benefit ceiling.
A2) For every incoming sensation, determine the
level of benefit/detriment
brought to the intelligent entity by the
sensation.
A3) Unite all benefit and detriment levels
into a single value. (preferably, take
the absolute value of each, double the
beneficial values to distinguish them
from the detrimental ones. Apply the
Lorentz transform to each value in the
set in series, e.g. square root of
(Value1^2 / Value2^2 / Value3^2, etc.))
Call this the unified benefit value. The
unified benefit value must be less than the
behavioral benefit ceiling.
A4) Associate the unified benefit value with
the lastest behavioral pattern. Use the
unified benefit value as a key to find
a new place for the behavioral pattern
in the set of all available behavioral
patterns. Sort the behavioral pattern
vector until this is done.
A5) Choose the behavior pattern at the top of
the behavioral pattern list. At first the
beneficial qualities of each behaviorial
pattern will be tested sequentially.
However, after the initial testing phases
the algorithm will reliably assign the most
consistently beneficial pattern sets to the
top of the list. Behavior will be, in most
cases, optimal to the intelligent entity's
sensory situations.
Serial programming methods don't cut it. The brain's circuitry is much more efficient, because it has plasticity. Brain neurons may act as transistors, linking themselves together into processing systems not unlike those of CPUs. The brain can optimize its own function through random action, effectively generating ever changing sets of microprocessor-like intelligence systems of varying complexity and performance. A dynamic processing system could simulate a -true- neural network more effeciently than a sequential "hardcoded" system could.
So long as we rely on serial coding methods when we write our programs, the base of people who are capable of programming will remain small. Only a handful of people, relative to the entire population, are intelligent enough to program computers in source code. Dynamic source can tie source code generation to visual and audial input.... Ah I'll just work on it, I think the majority thinks this is a good idea, and I don't think that those who don't get it already are intuitive enough to understand until they see it with their own eyes. (at which point they will embrace and defend the new establishment just as they defended the old, LOL)
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Wed Aug 25, 2004 5:27 pm Post subject: |
[quote] |
|
At the same time, this isn't my project alone. So as I develop these technologies, it will be simple to apply them as traditional methods, if that is what is desired. Already I have completed a virtual machine with full branching, subroutine, RAM, calculation, variable, multitasking, parsing, and struct functionality. Even though I've not integrated class-based OOP into my codes because I've not felt the need to, I undestand how classes and methods evolve from structs and functions. If a traditional object-oriented script is what is desired for the virtual convention, or even less, then I can provide either quickly.
|
|
Back to top |
|
 |
cloudchild Fluffy Bunny of Doom
Joined: 09 Jul 2004 Posts: 18 Location: PANTS!
|
Posted: Wed Aug 25, 2004 6:38 pm Post subject: |
[quote] |
|
Bringing this back on topic and way from inaneness:
personally, i think either the Java idea or the PHP/Javascript would be the best (for speed of creation), with the idea of a unique networking
system coded in C/C++ falling in right behind that.
Hmm. So....
are we going to do this?
My Java skills are poor, but I can sharpen them and help out where needed. _________________ BOOGA!
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|