View previous topic - View next topic |
Author |
Message |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Fri Oct 01, 2004 2:08 pm Post subject: Matrices for super filters! |
[quote] |
|
I wrote up a buch of matrix algorithms. Should be easy to make custom graphics filters with, as well as any other filter or gradient effect you can think of. Just tinker with the variables! :D
I know that mathematicians long ago developed these techniques already, but not everyone needs things explicitly spelled out for them to have adequate understanding, and it is for those people that I work.
Quote: |
// |*| Matrix JS - A set of operative functions for matrices.
//
function CreaseMatrix (Destination, Auxillary, Source) {
for (Destination.Index.valueOf =
Destination.Origin.valueOf;
Destination.Index.valueOf
< Destination.Limit.valueOf;
Destination.Index.valueOf++) {
Destination.valueOf[Destination.Index.valueOf].valueOf =
Source.valueOf[Destination.Index.valueOf].valueOf
+ Auxillary.valueOf;
}
}
function CreaseVector (Destination, Auxillary, Source) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.X.valueOf].valueOf
+ Auxillary.valueOf;
}
return Destination;
}
function CreasePlane (Destination, Auxillary, Source) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
< Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
+ Auxillary.valueOf;
}
}
return Destination;
}
function CreaseSpace (Destination, Auxillary, Source) {
for (Destination.Index.Z.valueOf =
Destination.Origin.Z.valueOf;
Destination.Index.Z.valueOf
< Destination.Limit.Z.valueOf;
Destination.Index.Z.valueOf++) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
< Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
+ Auxillary.valueOf;
}
}
}
return Destination;
}
function CreaseTimeframe (Destination, Auxillary, Source) {
for (Destination.Index.T.valueOf =
Destination.Origin.T.valueOf;
Destination.Index.T.valueOf
< Destination.Limit.T.valueOf;
Destination.Index.T.valueOf++) {
for (Destination.Index.Z.valueOf =
Destination.Origin.Z.valueOf;
Destination.Index.Z.valueOf
< Destination.Limit.Z.valueOf;
Destination.Index.Z.valueOf++) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
< Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
+ Auxillary.valueOf;
}
}
}
}
return Destination;
}
function CreaseUniverse (Destination, Auxillary, Source) {
for (Destination.Index.U.valueOf =
Destination.Origin.U.valueOf;
Destination.Index.U.valueOf
Destination.Limit.U.valueOf;
Destination.Index.U.valueOf++) {
for (Destination.Index.T.valueOf =
Destination.Origin.T.valueOf;
Destination.Index.T.valueOf
Destination.Limit.T.valueOf;
Destination.Index.T.valueOf++) {
for (Destination.Index.Z.valueOf =
Destination.Origin.Z.valueOf;
Destination.Index.Z.valueOf
Destination.Limit.Z.valueOf;
Destination.Index.Z.valueOf++) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.U.valueOf]
[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.U.valueOf]
[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
+ Auxillary.valueOf;
}
}
}
}
}
return Destination;
}
function CreaseMultiverse (Destination, Auxillary, Source) {
for (Destination.Index.T.valueOf =
Destination.Origin.T.valueOf;
Destination.Index.T.valueOf
< Destination.Limit.T.valueOf;
Destination.Index.T.valueOf++) {
for (Destination.Index.T.valueOf =
Destination.Origin.T.valueOf;
Destination.Index.T.valueOf
< Destination.Limit.T.valueOf;
Destination.Index.T.valueOf++) {
for (Destination.Index.T.valueOf =
Destination.Origin.T.valueOf;
Destination.Index.T.valueOf
< Destination.Limit.T.valueOf;
Destination.Index.T.valueOf++) {
for (Destination.Index.Z.valueOf =
Destination.Origin.Z.valueOf;
Destination.Index.Z.valueOf
< Destination.Limit.Z.valueOf;
Destination.Index.Z.valueOf++) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
< Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.M.valueOf]
[Destination.Index.U.valueOf]
[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.M.valueOf]
[Destination.Index.U.valueOf]
[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
+ Auxillary.valueOf;
}
}
}
}
}
}
return Destination;
}
function CreaseContinuum (Destination, Auxillary, Source) {
for (Destination.Index.valueOf =
Destination.Origin.valueOf;
Destination.Index.valueOf
< Destination.Limit.valueOf;
Destination.Index.valueOf++) {
Destination.valueOf[Destination.Index.valueOf].valueOf =
Source.valueOf[Destination.Index.valueOf].valueOf
+ Auxillary.valueOf;
ScaleContinuum(Destination.valueOf[Destination.Index.valueOf],
Auxillary.valueOf[Auxillary.Index.valueOf],
Source.valueOf[Source.Index.valueOf]);
}
}
function ScaleMatrix (Destination, Auxillary, Source) {
for (Destination.Index.valueOf =
Destination.Origin.valueOf;
Destination.Index.valueOf
< Destination.Limit.valueOf;
Destination.Index.valueOf++) {
Destination.valueOf[Destination.Index.valueOf].valueOf =
Source.valueOf[Destination.Index.valueOf].valueOf
* Auxillary.valueOf;
}
}
function ScaleVector (Destination, Auxillary, Source) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.X.valueOf].valueOf
* Auxillary.valueOf;
}
return Destination;
}
function ScalePlane (Destination, Auxillary, Source) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
< Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
* Auxillary.valueOf;
}
}
return Destination;
}
function ScaleSpace (Destination, Auxillary, Source) {
for (Destination.Index.Z.valueOf =
Destination.Origin.Z.valueOf;
Destination.Index.Z.valueOf
< Destination.Limit.Z.valueOf;
Destination.Index.Z.valueOf++) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
< Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
* Auxillary.valueOf;
}
}
}
return Destination;
}
function ScaleTimeframe (Destination, Auxillary, Source) {
for (Destination.Index.T.valueOf =
Destination.Origin.T.valueOf;
Destination.Index.T.valueOf
< Destination.Limit.T.valueOf;
Destination.Index.T.valueOf++) {
for (Destination.Index.Z.valueOf =
Destination.Origin.Z.valueOf;
Destination.Index.Z.valueOf
< Destination.Limit.Z.valueOf;
Destination.Index.Z.valueOf++) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
< Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
* Auxillary.valueOf;
}
}
}
}
return Destination;
}
function ScaleUniverse (Destination, Auxillary, Source) {
for (Destination.Index.U.valueOf =
Destination.Origin.U.valueOf;
Destination.Index.U.valueOf
Destination.Limit.U.valueOf;
Destination.Index.U.valueOf++) {
for (Destination.Index.T.valueOf =
Destination.Origin.T.valueOf;
Destination.Index.T.valueOf
Destination.Limit.T.valueOf;
Destination.Index.T.valueOf++) {
for (Destination.Index.Z.valueOf =
Destination.Origin.Z.valueOf;
Destination.Index.Z.valueOf
Destination.Limit.Z.valueOf;
Destination.Index.Z.valueOf++) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.U.valueOf]
[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.U.valueOf]
[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
* Auxillary.valueOf;
}
}
}
}
}
return Destination;
}
function ScaleMultiverse (Destination, Auxillary, Source) {
for (Destination.Index.M.valueOf =
Destination.Origin.M.valueOf;
Destination.Index.M.valueOf
< Destination.Limit.M.valueOf;
Destination.Index.M.valueOf++) {
for (Destination.Index.U.valueOf =
Destination.Origin.U.valueOf;
Destination.Index.U.valueOf
< Destination.Limit.U.valueOf;
Destination.Index.U.valueOf++) {
for (Destination.Index.T.valueOf =
Destination.Origin.T.valueOf;
Destination.Index.T.valueOf
< Destination.Limit.T.valueOf;
Destination.Index.T.valueOf++) {
for (Destination.Index.Z.valueOf =
Destination.Origin.Z.valueOf;
Destination.Index.Z.valueOf
< Destination.Limit.Z.valueOf;
Destination.Index.Z.valueOf++) {
for (Destination.Index.Y.valueOf =
Destination.Origin.Y.valueOf;
Destination.Index.Y.valueOf
< Destination.Limit.Y.valueOf;
Destination.Index.Y.valueOf++) {
for (Destination.Index.X.valueOf =
Destination.Origin.X.valueOf;
Destination.Index.X.valueOf
< Destination.Limit.X.valueOf;
Destination.Index.X.valueOf++) {
Destination.valueOf[Destination.Index.M.valueOf]
[Destination.Index.U.valueOf]
[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf =
Source.valueOf[Destination.Index.M.valueOf]
[Destination.Index.U.valueOf]
[Destination.Index.T.valueOf]
[Destination.Index.Z.valueOf]
[Destination.Index.Y.valueOf]
[Destination.Index.X.valueOf].valueOf
* Auxillary.valueOf;
}
}
}
}
}
}
return Destination;
}
function ScaleContinuum (Destination, Auxillary, Source) {
for (Destination.Index.valueOf =
Destination.Origin.valueOf;
Destination.Index.valueOf
< Destination.Limit.valueOf;
Destination.Index.valueOf++) {
Destination.valueOf[Destination.Index.valueOf].valueOf =
Source.valueOf[Destination.Index.valueOf].valueOf
* Auxillary.valueOf;
ScaleContinuum(Destination.valueOf[Destination.Index.valueOf],
Auxillary.valueOf[Auxillary.Index.valueOf],
Source.valueOf[Source.Index.valueOf]);
}
} |
Last edited by tcaudilllg on Sun Oct 03, 2004 3:56 pm; edited 1 time in total
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
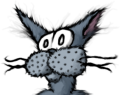
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Fri Oct 01, 2004 7:27 pm Post subject: |
[quote] |
|
I hardly see as how that is useful, especially in a language that fails to connote type information to the user. Along with that, you seem to have applied pseudo-intellecutal, obscure names to functionality that is more commonly expressed via some sort of linear-algebra operator. If your phobia of proper OOP wasn't so preventative, much of this could be simplified.
Again, I reiterate: this is not terribly useful for a forum dedicated to RPG Development. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Fri Oct 01, 2004 8:14 pm Post subject: |
[quote] |
|
I'd have to agree with LeoDraco. This piece of code it at the same time geared towards general public instead of RPG developers, as well as not interesting for general public.
I've moved it over to off-topic, where nearly anything is tolerated. Don't take this too hard, just take another look at the code and try to judge its usefulness to this community, taking into account previous responses you got to other pieces of code.
|
|
Back to top |
|
 |
bay Wandering Minstrel

Joined: 17 Mar 2004 Posts: 138 Location: new jersey, usa
|
Posted: Fri Oct 01, 2004 8:38 pm Post subject: |
[quote] |
|
what is this, javascript matrix transformations?
what the hell are you writing?
.02$
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sat Oct 02, 2004 1:57 am Post subject: |
[quote] |
|
LeoDraco wrote: | I hardly see as how that is useful, especially in a language that fails to connote type information to the user. Along with that, you seem to have applied pseudo-intellecutal, obscure names to functionality that is more commonly expressed via some sort of linear-algebra operator. If your phobia of proper OOP wasn't so preventative, much of this could be simplified.
Again, I reiterate: this is not terribly useful for a forum dedicated to RPG Development. |
And you are dead wrong. Wrong like George Bush wrong. ...Oh excuse me, you used the suggestive word "seem". Hah. Obscure? How in touch with reality are you?!?!?
Proper OOP? You still haven't demonstrated the merit of methods. Redundancy, I say.
As I said, I'm not working for your tastes. I'm improving the avilability of knowledge. Not everyone is a psychophant Karl Rove-alike like LeoDraco who can parse the Einstein field equations at a whim and idly play with people's emotions.
How was this document not useful?!!??!!? Let me get this straight: we are programming virtual storytelling environments that imply practical measures like scripting languages....--I'll save the rant. The author of ika has suggestions of his own. I'll produce a sister version of the code to reflect his outlook. Then we will see what is said.
Still, matrices are a vital component in systems development. Greater use and understanding of matrix operations will vastly expand the speed at which programmers develop large systems, graphical or otherwise. I am disappointed in Bjorn for not percieving the utility of matrices use and understanding to RPG developers.
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
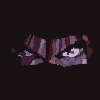
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Sat Oct 02, 2004 2:16 am Post subject: |
[quote] |
|
LordGalbalan wrote: | How was this document not useful?!!??!!? |
Anyone who seeks information on matrix math or programming matrix math will find it in a book, or on a site that does a good job explaining it. Why is this not useful? No one here programs in JS - at least not for game purposes - so you really need to stop bloating these forums with your bullshit code that no one cares to read.
I'm tired of looking at all these threads you make with stupid chunks of code. Stop it.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sat Oct 02, 2004 3:40 am Post subject: |
[quote] |
|
Maybe this version will be deemed more acceptable.
Quote: |
// |*| Matrix JS_INTP
//
function creaseMatrix (dest, aux, src) {
for (i = dest.slice.start; i < dest.slice.end; i++) {
dest[i] = src[i] + aux;
}
}
function creaseVector (dest, aux, src) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[x] = src[x] + aux;
}
return dest;
}
function creasePlane (dest, aux, src) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[y][x] = src[y][x] + aux;
}
}
return dest;
}
function creaseSpace (dest, aux, src) {
for (z = dest.slice.z.start; z < dest.slice.z.end; z++) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[z][y][x] = src[z][y][x] + aux;
}
}
}
return dest;
}
function creaseTimeframe (dest, aux, src) {
for (t = dest.slice.t.start; t < dest.slice.t.end; t++) {
for (z = dest.slice.z.start; z < dest.slice.z.end; z++) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[t][z][y][x] = src[t][z][y][x] + aux;
}
}
}
}
return dest;
}
function creaseUniverse (dest, aux, src) {
for (u = dest.slice.u.start; u < dest.slice.u.end; u++) {
for (t = dest.slice.t.start; t < dest.slice.t.end; t++) {
for (z = dest.slice.z.start; z < dest.slice.z.end; z++) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[u][t][z][y][x] = src[u][t][z][y][x] + aux;
}
}
}
}
}
return dest;
}
function creaseMultiverse (dest, aux, src) {
for (m = dest.slice.m.start; m < dest.slice.m.end; m++) {
for (u = dest.slice.u.start; u < dest.slice.u.end; u++) {
for (t = dest.slice.t.start; t < dest.slice.t.end; t++) {
for (z = dest.slice.z.start; z < dest.slice.z.end; z++) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[m][u][t][z][y][x] = src[m][u][t][z][y][x] + aux;
}
}
}
}
}
}
return dest;
}
function creaseContinuum (dest, aux, src) {
for (i = dest.slice.start; i < dest.slice.end; i++) {
dest[i] = src[i] + aux;
creaseContinuum(dest[i], aux[aux.i], src[src.i]);
}
}
function scaleMatrix (dest, aux, src) {
for (i = dest.slice.start; i < dest.slice.end; i++) {
dest[i] = src[i] * aux;
}
}
function scaleVector (dest, aux, src) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[x] = src[x] * aux;
}
return dest;
}
function scalePlane (dest, aux, src) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[y][x] = src[y][x] * aux;
}
}
return dest;
}
function scaleSpace (dest, aux, src) {
for (z = dest.slice.z.start; z < dest.slice.z.end; z++) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[z][y][x] = src[z][y][x] * aux;
}
}
}
return dest;
}
function scaleTimeframe (dest, aux, src) {
for (t = dest.slice.t.start; t < dest.slice.t.end; t++) {
for (z = dest.slice.z.start; z < dest.slice.z.end; z++) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[t][z][y][x] = src[t][z][y][x] * aux;
}
}
}
}
return dest;
}
function scaleUniverse (dest, aux, src) {
for (u = dest.slice.u.start; u < dest.slice.u.end; u++) {
for (t = dest.slice.t.start; t < dest.slice.t.end; t++) {
for (z = dest.slice.z.start; z < dest.slice.z.end; z++) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[u][t][z][y][x] = src[u][t][z][y][x] * aux;
}
}
}
}
}
return dest;
}
function scaleMultiverse (dest, aux, src) {
for (m = dest.slice.m.start; m < dest.slice.m.end; m++) {
for (u = dest.slice.u.start; u < dest.slice.u.end; u++) {
for (t = dest.slice.t.start; t < dest.slice.t.end; t++) {
for (z = dest.slice.z.start; z < dest.slice.z.end; z++) {
for (y = dest.slice.y.start; y < dest.slice.y.end; y++) {
for (x = dest.slice.x.start; x < dest.slice.x.end; x++) {
dest[m][u][t][z][y][x] = src[m][u][t][z][y][x] + aux;
}
}
}
}
}
}
return dest;
}
function scaleContinuum (dest, aux, src) {
for (i = dest.slice.start; i < dest.slice.end; i++) {
dest[i] = src[i] * aux;
scaleContinuum(dest[i], aux[aux.i], src[src.i]);
}
} |
Off note: I believe that if LeoDraco took the time to restate Einstein's work in a language we can all understand he'd make some history.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
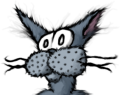
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sat Oct 02, 2004 5:17 am Post subject: |
[quote] |
|
LordGalbalan wrote: | LeoDraco wrote: | I hardly see as how that is useful, especially in a language that fails to connote type information to the user. Along with that, you seem to have applied pseudo-intellecutal, obscure names to functionality that is more commonly expressed via some sort of linear-algebra operator. If your phobia of proper OOP wasn't so preventative, much of this could be simplified.
Again, I reiterate: this is not terribly useful for a forum dedicated to RPG Development. |
And you are dead wrong. Wrong like George Bush wrong. ...Oh excuse me, you used the suggestive word "seem". Hah. Obscure? How in touch with reality are you?!?!? |
Obviously more so than you, sir. At the very least, I do not need to (A) bloat my ego, (B) post useless code in a bad scripting language (see more on this below), and (C) resort to AOL-netisms to connote meaning.
As discussed below, I have no problems with writing code in various languages: I myself know at least a dozen languages, and adequately utilize them for the jobs to which they are suited best. I hardly see javascript as an appropriate language for anything except DHTML or form-validation. Among the bad things in javascript is its total disregard to type-safety: you could practically throw anything into those functions, and suddenly the javascript system is borked. Look at ML: it's a type-strict language, with good type-checking, and it'll bitch and moan if a function is misused. And all of this transparently: the programmer needn't even provide type information for function-declarations.
Quote: | Proper OOP? You still haven't demonstrated the merit of methods. Redundancy, I say. |
I have absolutely no problem with procedural programming -- where it is the paradigm that is best applicable to the situation. Obviously, there exist languages and projects for which OOP is not the best paradigm to utilize. However, perhaps the most applicable area in which to properly utilize OOP is in modeling types. You, here, have presented a modeling of a type, whose implementation would be better served by OOP.
The merit of methods? You obviously have not designed a complex composite heirarchy, in which code is reused by deriving classes. Methods provide (at least) three functionalities, at least one of which is not adequately facilitated in non-OO languages: (1) they provide code-reuse; (2) deriving objects can selectively choose which code to reuse, which promotes the idea of extendability; (3) they provide a black-box interface for accessing the object's data, which promotes the idea of maintainability.
Now, with respect to point (2), to imbue extendability into a non-OO environment, various methods exist, most of which are kludgy, at best. One could provide to a function a hook for a callback, which the user would supply when calling the function. Alternatively, you could place a massive switch-construct in the function, which selectively executes code based upon the form of the data it is working on. By and large, both of these constructs can be avoided by utilizing methods, along with OO-Design Patterns. Take the Strategy-Pattern, for example: by utilizing the overwriting of a sub-classes vtable entry for a method, we can provide a mechanism for run-time interchangeability of the very algorithms that act upon our data-structures. Is this useless? Hardly.
Several languages allow a programmer to provide prototypical instances of a function that are separate from the implementation of the function. With respect to (3), a good OO language also provides this mechanism via a class interface. For clients that rely upon code written in a library, black-boxes are a good thing: it would be terrible for a client to have to re-compile a program they rely upon just because the implementer of a library whimsically decided to change the implementation of the library. Implementation-changes should be as transparent as possible. Black-boxes formed by interfaces promote this idea. This is something that your usage of javascript -- at the very least, the javascript that you have posted here -- fails to do.
Quote: | As I said, I'm not working for your tastes. I'm improving the avilability of knowledge. Not everyone is a psychophant Karl Rove-alike like LeoDraco who can parse the Einstein field equations at a whim and idly play with people's emotions. |
There are better ways to promote knowledge than by posting code in a poor language to a forum that will not even utilize it. Like your gravitation model. While it might be interesting, the particular forum you posted it to was geared towards RPG-related development issues. Neither of those peices of code seemed to have any immediate applicability to that topic.
Quote: | How was this document not useful?!!??!!? Let me get this straight: we are programming virtual storytelling environments that imply practical measures like scripting languages....--I'll save the rant. The author of ika has suggestions of his own. I'll produce a sister version of the code to reflect his outlook. Then we will see what is said. |
While I cannot believe I am actually using code that you have written for an example, I point to the code you wrote for the convention project. While my opinion of that code is very low, at the very least you provided documentation along with it. Along with suggested usage. None of that is here. Not only that, but this document of yours provides none of the theory behind the code; and it especially does not provide an example utilization. A person who picks up this code has absolutely no idea why it is, other than it can be used to "make custom graphics filters with, as well as any other filter or gradient effect you can think of" [sic]. That tells us nothing. This, I think, is one of the greatest problems with your posting code here (other than the size; really, a link to some source document somewhere would be much better, and, no doubt, easier on the server): you dump it without much explanation. YOUR CODE DOES NOT SPEAK FOR ITSELF.
But please, please, please! If you still persist in posting kludge here, do so in a language that, if not widely used, is at least abstract: pseudo-code is a very, very good thing.
Quote: | Still, matrices are a vital component in systems development. Greater use and understanding of matrix operations will vastly expand the speed at which programmers develop large systems, graphical or otherwise. I am disappointed in Bjørn for not percieving the utility of matrices use and understanding to RPG developers. |
Yeah, matrices are great things. When their implementation is not obtuse. While my experience with graphics APIs is not as varied as it could be, I do know that GL has an excellent matrix paradigm that it utilizes to perform its graphics operations. And all of the computations and such are pushed to the metal, which implies, assuming appropriate hardware, much faster computation times than a software solution. Especially a solution as non-optimized as this. (Seriously, your "ScaleMultiverse" routine is O( n^5 ).) _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
janus Mage
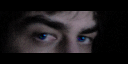
Joined: 29 Jun 2002 Posts: 464 Location: Issaquah, WA
|
Posted: Sat Oct 02, 2004 11:52 am Post subject: |
[quote] |
|
Wow, Draco. You really live that 'Scholar' title out.
I would have just responded with something along the lines of 'cocks', but you really went into detail there. Very well-written post.
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Sat Oct 02, 2004 12:11 pm Post subject: |
[quote] |
|
LordGalbalan wrote: | Still, matrices are a vital component in systems development. Greater use and understanding of matrix operations will vastly expand the speed at which programmers develop large systems, graphical or otherwise. I am disappointed in Bjørn for not percieving the utility of matrices use and understanding to RPG developers. |
I can see very well that matrices are useful in many fields and have lots of applications. Many people would be doing themselves a favor by learning about them, including the casual RPG developer. Your code however, is useless to both those that don't know the math, and the people that do know the math. Writing it down in JavaScript didn't help with that.
Now the situation would be totally different if somebody was actually asking how to write a rotation matrix, scale a vector or draw a complex gradient. But at the moment, you just don't seem to be helping anybody.
|
|
Back to top |
|
 |
BigManJones Scholar
Joined: 22 Mar 2003 Posts: 196
|
Posted: Sat Oct 02, 2004 3:59 pm Post subject: |
[quote] |
|
1. javascript isn't a 'bad' language and it does have the prototypical oop ability Leo mentioned. Matrix operations are way outside of the set problems its suited for.
2. You code was a very incomplete subset of matrix operations; no rotations, no transform to a screen space, and no applying a matrix to a point which are very useful when imlementing a software renderer for example.
3. The variable names are unintuitive.
4. The code box sucks; it should preserve tabs.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sun Oct 03, 2004 9:09 am Post subject: |
[quote] |
|
To all:
After carefully considering your arguments, I am in agreement that I overstepped the bounds of good taste by presenting the full scope of my routines on these boards. They are, in full, too lengthly to merit positive discussion. Although I will continue to share my derivations and consolidations with this community, because I believe that it is meritous, I will endevor also in the future to document my work in full before it is released; to communicate only as much source to the board as is needed to demonstrate its purpose; and to present working RPG-related implementations of my models alongside them.
LeoDraco:
Thank you for your vote of dignity. Although I continue to disagree with you on many things, I am certain that we can learn from one another in the future.
Ninkazu:
Matrix operations aren't easy to research. The information is there for all who can find it, but it is very difficult for all but a very mathematically talented few to understand. I would like to make it more understandable to the population en mass, and although I understand that my approach thus far has not been without problems I maintain that I have made necessary strides in the right direction.
Bjorn:
Although I realize your position on "reinventing the wheel", I must take issue with it anyway. I am, and will remain, hesitant to employ libraries that are under any form of license or of copyright. In a worse case scenario problems could emerge, and I for one would prefer to have public domain information available to me in a form that I can understand, that I may restate that information in my own prose without fear of legal quagmire, as opposed to running the risk of being shanghied by an unexpected turn of events. The free can become unfree, and I have witnessed the phenomenon firsthand.
BigManJones:
Thank you for your support! :D I would appreciate the benefit of your assistance further. How can I make the variables names more intuitive for you? You are right in assessing that my work is incomplete: what I presented here was only a fraction of what I hope to accomplish. However, what I have done already is more effective than may at first be apparent.... I agree, the code box should preserve tabs and spaces! :)
Finally:
I am in no way repentant for my actions. Something must be done. The revolution that Mandrake is working hard to foment, although unstalled, is slowing. The indie RPG scene is now defined, and unless effort is made to improve the baseline quality standards of the games it would produce, it will grow stagnant. The road to better games lies not in architectural agreements alone, but in the power of the individual. The power of the individual, who's drive and ambition is the lifeblood of indie development, can only be accented through greater understanding. A man must walk before he can run, but if he is used to walking fast he can run faster and longer. As LeoDraco noted, my source codes here have modeled type definitions, and in so doing have created a plank upon which to bulid better architecture. Stronger architecture, and the implicity it provides, is the key to progress and evolution. It is through architecture that a problem is modeled and implemented; therefore stronger, more complex architecture will allow larger problems to be modeled as easily as smaller ones. It will be that much easier to craft creative vision into physical form once the weaker, simple architectures of today's indie scene are supplanted by the stronger architectures that matrix operations in particular can provide. Massively multidimensional programming is the way to the future.
Javascript is the best known data modeling language in the world, and DHTML is very, very powerful due to its strong and evolving architecture. It doesn't break when an operation is made upon a type that does not support the operation, although null values may appear. I can open any word processor and type in a few lines, save the file and open it in any modern browser, and behold it is functional. It is very easy to learn, free for anyone to use at a whim, and can run in cases that would break compiled codes. (albeit with incorrect results) Even the Sphere RPG engine uses it, so what is not to like?
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
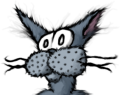
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sun Oct 03, 2004 9:48 am Post subject: |
[quote] |
|
LordGalbalan wrote: | It doesn't break when an operation is made upon a type that does not support the operation, although null values may appear. |
I would mildly contest that. Let us consider a procedure in Javascript that is buggy -- it does something wonky, that, as you put it, "would break compiled codes". While execution of said procedure will not cause the browser to seg-fault and die, execution of the procedure will immediately halt. While details about the halt may be acquired via probing the browsers javascript console (should it have one), no exceptions are, by default, thrown. (I realize that one could cause an error window to open upon error via a specialized error handler, but the point is that, be default, the browser doesn't suggest to the user that anything is wrong.) This, I hold, is bad policy.
Quote: | I can open any word processor and type in a few lines, save the file and open it in any modern browser, and behold it is functional. |
The same may be done with Java, Python, and, with a modicum of effort, C++. And various other languages, regardless of their being interpreted or compiled. Javascript is hardly revolutionary. Its only virtue is that it is imbeded within a browser.
Quote: | It is very easy to learn, |
As is any language. Programming is not about knowing a language's syntax, or even about knowing languages at all; programming is about problem solving. Languages are merely a way of conscribing the metal to facilitate in solving a particular problem.
Quote: | free for anyone to use at a whim, |
As is C++, and Python, and ML, and LISP/Scheme, and (barring various technicalities) Java, and a virtual googolplex of other languages. While it might be incorrect to make this comparison, given an infinite universe, there are practically more languages written than there are stars in the sky. And, most of them are better known, and better in syntax/functionality/ease-of-use, than Javascript.
Quote: | and can run in cases that would break compiled codes. (albeit with incorrect results) |
You know those seg-faults, core-dumps, and other heinous "break"ings that "compiled codes" generate? Yeah, that's the presence of bugs manifesting themselves in nefarious ways. Which means that the particular piece of code is improperly written. (Either syntactically, semantically, or logically.) Part of the software engineering lifecycle involves finding and eradicating bugs. I would rather have a compiler spit out syntactic/semantic errors, rather than have a function mysteriously not work, and not be informed about it. I would rather get some seg-fault or core-dump rather than, again, have code not work due to some logic bug. There is a higher degree of traceability with "compiled codes". _________________ "...LeoDraco is a pompus git..." -- Mandrake
Last edited by LeoDraco on Sun Oct 03, 2004 1:56 pm; edited 2 times in total
|
|
Back to top |
|
 |
phydeaux Pretty, Pretty Fairy Princess

Joined: 01 Sep 2004 Posts: 5 Location: Detroit
|
Posted: Sun Oct 03, 2004 1:30 pm Post subject: |
[quote] |
|
Has anyone actually read this code? It looks like the crease series just add a constant to each element of the matrix, and the scale series scale each element of the matrix. So what? Anyone could have written that without even thinking. Not only that, but for "scale Multiverse" (whatever the fuck a multiverse is- when posting code it also helps to explain the terminology and what it does) he didn't change the code he clearly copied from the crease Multiverse function, so this code is also incorrect as far as what little functionality it had despite the mistake.
Try writing a matrix inversion routine using Gaussian elimination, or a routine that calculates the eigenvalues and eigenvectors of a matrix. People have even done this in Javascript.
There's nothing wrong with Javascript, though it clearly has its place. The problem is that this code simply is not useful. Please put a little bit more effort into your developments before trying to get everyone to use your code.
|
|
Back to top |
|
 |
Happy JonA's American snack pack

Joined: 03 Aug 2002 Posts: 200
|
Posted: Sun Oct 03, 2004 2:33 pm Post subject: |
[quote] |
|
LordGalbalan wrote: | And you are dead wrong. Wrong like George Bush wrong. ...Oh excuse me, you used the suggestive word "seem". Hah. Obscure? How in touch with reality are you?!?!?
Proper OOP? You still haven't demonstrated the merit of methods. Redundancy, I say.
As I said, I'm not working for your tastes. I'm improving the avilability of knowledge. Not everyone is a psychophant Karl Rove-alike like LeoDraco who can parse the Einstein field equations at a whim and idly play with people's emotions.
How was this document not useful?!!??!!? Let me get this straight: we are programming virtual storytelling environments that imply practical measures like scripting languages....--I'll save the rant. The author of ika has suggestions of his own. I'll produce a sister version of the code to reflect his outlook. Then we will see what is said.
Still, matrices are a vital component in systems development. Greater use and understanding of matrix operations will vastly expand the speed at which programmers develop large systems, graphical or otherwise. I am disappointed in Bjørn for not percieving the utility of matrices use and understanding to RPG developers. |
LordGalbalan wrote: | To all:
After carefully considering your arguments, I am in agreement that I overstepped the bounds of good taste by presenting the full scope of my routines on these boards. They are, in full, too lengthly to merit positive discussion. Although I will continue to share my derivations and consolidations with this community, because I believe that it is meritous, I will endevor also in the future to document my work in full before it is released; to communicate only as much source to the board as is needed to demonstrate its purpose; and to present working RPG-related implementations of my models alongside them.
LeoDraco:
Thank you for your vote of dignity. Although I continue to disagree with you on many things, I am certain that we can learn from one another in the future.
Ninkazu:
Matrix operations aren't easy to research. The information is there for all who can find it, but it is very difficult for all but a very mathematically talented few to understand. I would like to make it more understandable to the population en mass, and although I understand that my approach thus far has not been without problems I maintain that I have made necessary strides in the right direction.
Bjørn:
Although I realize your position on "reinventing the wheel", I must take issue with it anyway. I am, and will remain, hesitant to employ libraries that are under any form of license or of copyright. In a worse case scenario problems could emerge, and I for one would prefer to have public domain information available to me in a form that I can understand, that I may restate that information in my own prose without fear of legal quagmire, as opposed to running the risk of being shanghied by an unexpected turn of events. The free can become unfree, and I have witnessed the phenomenon firsthand.
BigManJones:
Thank you for your support! :D I would appreciate the benefit of your assistance further. How can I make the variables names more intuitive for you? You are right in assessing that my work is incomplete: what I presented here was only a fraction of what I hope to accomplish. However, what I have done already is more effective than may at first be apparent.... I agree, the code box should preserve tabs and spaces! :)
Finally:
I am in no way repentant for my actions. Something must be done. The revolution that Mandrake is working hard to foment, although unstalled, is slowing. The indie RPG scene is now defined, and unless effort is made to improve the baseline quality standards of the games it would produce, it will grow stagnant. The road to better games lies not in architectural agreements alone, but in the power of the individual. The power of the individual, who's drive and ambition is the lifeblood of indie development, can only be accented through greater understanding. A man must walk before he can run, but if he is used to walking fast he can run faster and longer. As LeoDraco noted, my source codes here have modeled type definitions, and in so doing have created a plank upon which to bulid better architecture. Stronger architecture, and the implicity it provides, is the key to progress and evolution. It is through architecture that a problem is modeled and implemented; therefore stronger, more complex architecture will allow larger problems to be modeled as easily as smaller ones. It will be that much easier to craft creative vision into physical form once the weaker, simple architectures of today's indie scene are supplanted by the stronger architectures that matrix operations in particular can provide. Massively multidimensional programming is the way to the future.
Javascript is the best known data modeling language in the world, and DHTML is very, very powerful due to its strong and evolving architecture. It doesn't break when an operation is made upon a type that does not support the operation, although null values may appear. I can open any word processor and type in a few lines, save the file and open it in any modern browser, and behold it is functional. It is very easy to learn, free for anyone to use at a whim, and can run in cases that would break compiled codes. (albeit with incorrect results) Even the Sphere RPG engine uses it, so what is not to like? |
Cocks!
|
|
Back to top |
|
 |
 |
Page 1 of 4 |
All times are GMT Goto page 1, 2, 3, 4 Next
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|