 |
|
View previous topic - View next topic |
Author |
Message |
Unknown Moira's Silly Little Slave Bitch
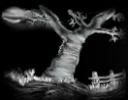
Joined: 19 Jul 2005 Posts: 82 Location: Behind you...
|
Posted: Sat Sep 30, 2006 2:03 pm Post subject: RPG Stats |
[quote] |
|
In a rpg how do you keep track of what each player/npc is able to do as far as attacks/spells ect....
This is some simple pseudocode that I came up with...
Code: |
...
int NameOfNPC_Attack_Counter = 0;
int NameOfNPC_Attack_Chance = 40;
boolean NameOfNPC_Attack_Hit = false;
int NameOfNPC_Attack_Strength = 10;
array NameOfNPC_Attack_Per[100];
for(lp=0;lp<NameOfNPC_Attack_Per.size+1;lp++){
chance = int(rnd*1);
if(chance == 1 && NameOfNPC_Attack_Counter < NameOfNPC_Attack_Chance){
NameOfNPC_Attck_Per[lp] = 1;
NameOfNPC_Attack_Counter++;
}
}
NameOfNPC_Attack_Counter = 0;
...
if(WantToAttck){
if(NameOfNPC_Attack_Counter == 100){
NameOfNPC_Attack_Counter = 0;
}
NameOfNPC_Attack_Counter++;
chance = int(rnd*100);
if(NameOfNPC_Attack_Per[chance] == 1){
NameOfNPC_Attack_Hit = true;
}
}
if(NameOfNPC_Attack_Hit){
NameOfNPC_Attack_Hit = false;
Hit_Value = int(rnd * NameOfNPC_Attack_Strength+1)
}
...
|
Well, what do you use? (code/algorithm wise) _________________ Most people would succeed in small things if they were not troubled with such great ambitions.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
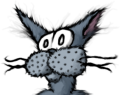
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sun Oct 01, 2006 8:02 am Post subject: |
[quote] |
|
Say what you will about the non-applicability of a given tool (in this case of this advocacy message, object oriented design) to all problem domains, but that pseudocode, notably for its lack of design, makes my brain explode in pain. I believe JonA over at GDR said it best when he expressed bafflement at (modern-day) programmers equating programming with tedious chores, at least as far as the fruits of their efforts portrayed their capability for design.
Now, I thought about offering an alternative using that most sexy of OOPLs, Ruby, but that would require me to actually grok what it is you are attempting to do here.
Knowns: you seem to be calculating hit percentages, or, to be slightly more precise, the probability that a given attack would hit. This supposition seems vindicated by the last line of code which does anything.
Unknowns: probability is, ipso facto, something inherently calculable for a given domain, so what's with the looping and the percentage array?
Alternative: while the following will, more than likely, be way off of what you implemented (this follows from the fact that you do not provide a hard specification for what your code does), it falls within the realm of possibility that it does approximate your code.
Code: | class Character
def initialize( strength, accuracy )
@strength = strength
@accuracy = accuracy
end
def hit_successful
rand(@accuracy)
end
def damage_dealt
rand(@strength)
end
def attack( foe )
foe.health -= damage_dealt if hit_successful
end
attr :health
end
# Lob'st thy Holy Hand Grenade at thy foe, who, being naughty in my eyes, shall snuff it...
Character.new( 10, 40 ).attack( Character.new( 20, 50 ) )
|
Even this is idiotic: for instance, attacks do not take into account an enemy's evasiveness nor defense; compounding this, I have not addressed your namespace pollution problem: you are creating, presumably, a gigantic number of variables for each character in your game, which is a maintenance nightmare; you are even hardcoding in the various characters your game contains, rather than segregating that data into some other location (such as a config file, some sort of game script, etc.).
(As you pointed out, this is pseudocode, so perhaps I am lambasting you unfairly? If your design (or proposed design) is cleaner than this, my apologies.) _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Nodtveidt Demon Hunter
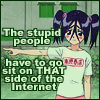
Joined: 11 Nov 2002 Posts: 786 Location: Camuy, PR
|
Posted: Sun Oct 01, 2006 5:29 pm Post subject: Re: RPG Stats |
[quote] |
|
Unknown wrote: | In a rpg how do you keep track of what each player/npc is able to do as far as attacks/spells ect.... |
I tend to keep it very very simple. Since every game I write requires a unique engine, I have no use for generic methods. I tend to simply use a list of clearly named variables (in structs when available and warranted) to store all character data, including actions. Each type of action has its own function or group of functions that carry out specific actions, and each character has flags that dictate whether they can use a specific action or not. I don't know how you'd apply this kind of thing to an OOP-heavy application but it works the same way in the end anyways, unless you have a penchant for making things unnecessarily complicated or are obsessed with genericism. _________________ If you play a Microsoft CD backwards you can hear demonic voices. The scary part is that if you play it forwards it installs Windows. - wallace
|
|
Back to top |
|
 |
Unknown Moira's Silly Little Slave Bitch
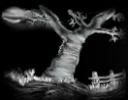
Joined: 19 Jul 2005 Posts: 82 Location: Behind you...
|
Posted: Mon Oct 02, 2006 1:34 am Post subject: Re: RPG Stats |
[quote] |
|
LOL!!! well hello Draco ^_^ ...
Quote: | (As you pointed out, this is pseudocode, so perhaps I am lambasting you unfairly? If your design (or proposed design) is cleaner than this, my apologies.) | well, mabey i should upload it when im done... then you and anyone else can blast it to hell and back and in return i might get useable code lololz... ;)
as you can tell it had been a late night and i got up a little blury minded when i posted that code... (note to self:wake up before posting) ;)
ok, well im going off to check out Ruby (its like the GOD of OOP right)
anyhow.... what the idea i posted was trying to do was this:
create an array that held the hit potential for each strike that was dealt accordding to set varibles... in the array was value 1 and 0 for hit or miss... ect ect ect
after thinkin bout it enough i guess you could just say screw that mess that i wrote and just use a rnd command each hit... DUH!!!
someone else post there ideas/psdcode/code on how an stats engine should be setup...
happy posting! _________________ Most people would succeed in small things if they were not troubled with such great ambitions.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
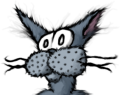
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Mon Oct 02, 2006 6:50 am Post subject: Re: RPG Stats |
[quote] |
|
nodtveidt wrote: | I don't know how you'd apply this kind of thing to an OOP-heavy application but it works the same way in the end anyways, unless you have a penchant for making things unnecessarily complicated or are obsessed with genericism. |
OOP/D do not intrinsically equate to "unnecessarily complicated," nor to "genericism;" at the very least, the ostensible gains in program readability support any percieved lack in productivity OOP yields. For instance, I, being firmly grounded in the beauty of object-oriented design and analysis, find my solution (assuming it does approximate Unknown's) much cleaner and easier to read than Unknown's solution. Maintainability and extensibility are, obviously so in practice, never much use for modern OOP, but they are not the only reasons to practice the paradigm.
If you find yourself being burdened by your design or the implementation of your design (regardless of the language you are using, or the paradigm's expressed therein), that suggests that something is wrong with your design or its implementation, not necessarily with the paradigm you are expressing it in. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Nodtveidt Demon Hunter
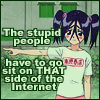
Joined: 11 Nov 2002 Posts: 786 Location: Camuy, PR
|
Posted: Mon Oct 02, 2006 1:04 pm Post subject: Re: RPG Stats |
[quote] |
|
LeoDraco wrote: | OOP/D do not intrinsically equate to "unnecessarily complicated," nor to "genericism;" |
I neither stated nor implied that they did. _________________ If you play a Microsoft CD backwards you can hear demonic voices. The scary part is that if you play it forwards it installs Windows. - wallace
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
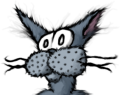
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Thu Oct 05, 2006 5:10 pm Post subject: Re: RPG Stats |
[quote] |
|
nodtveidt wrote: | LeoDraco wrote: | OOP/D do not intrinsically equate to "unnecessarily complicated," nor to "genericism;" |
I neither stated nor implied that they did. |
Not to get into a pissing match about semantics, but that was the impression I got, especially within the whole context of the sentence I was quoting from. That is, the whole, "I don't know how you'd apply this kind of thing in an OOP-heavy application" suggests that to me. You can write an entirely OO centric application (i.e. no free methods at all), and still achieve a lightweight architecture. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Nephilim Mage

Joined: 20 Jun 2002 Posts: 414
|
Posted: Sun Oct 08, 2006 7:29 am Post subject: |
[quote] |
|
Yeah, I've gotta go with LeoDraco on this one. Object-oriented design is particularly well-suited to modeling characters and objects and their interactions, especially when you have a lot of them.
For Sacraments, I had a generic "living creature" object which served as the basis for all living creatures that you could encounter during the game, from the player characters to the monsters. This base object class had many of the game system rules built into it, so that every living creature knew how to apply damage to itself, how to heal itself, how to defend and attack, how to report and modify its stats, how to equip and unequip objects and spells, how to save and load itself, how to display itself in the combat screen, etc.
Once you have that system set up, defining characters is as simple as setting their properties - what's their strength, what's in their spellbook, what sprite sheet do they use, etc., and the game system knows how to deal with each character.
And if you have a special character that needs to overrule the game rules, you just override that method in the object. For instance, boss monsters in Sacraments are handled just like any other monster (with better stats, of course), except that they draw themselves differently because they use a larger sprite sheet. So I created a "boss monster" class that inherited the "living creature" class and overrode the code that draws the character to the screen. Then, boss monsters knew how to draw themselves.
As a result, my "combat screen" code was very clean and wasn't bogged down with a bunch of special cases because all it did was tell all combatants to render themselves into the combat screen. The specialized code for drawing bosses was stored where it belongs - with the specialized type of character.
Another example is items and equipment. I wrote a generic "satchel" and "equipment" class that contains all the rules for inventory management - browsing, selecting, using, and selling objects. Then, to create objects for the game, I merely had to define new objects that extended those base classes, which was really easy and clean because each object only needed the code relevant for that object. For instance, the "Tanis Leaf" code just has a block of code that defines its core characteristics - it's value, when it can be used, the type of target it may be used on, etc. - and a method that gets called when the Tanis Leaf is used on a character. The only code I write for each object is code specific to that object, and the code for making inventory browsers and such may be kept very simple. This approach really allowed me to flex my creativity, because I could easily add new object types without much hassle, but they'd be flexible enough (since they call methods) that new objects could do just about anything I would want them to do.
In truth, practically everything in Sacraments is handled using an object-oriented design. Every view into the game world, every dialog box, every spell, every character, etc., are all objects. As such, I could easily mix, match, extend, and replace any part of my game from the fundamental camera view of the game world to the interplay between a particular weapon and suit of armor. Using an object-oriented design allowed me to easily modify the way my game world worked, and to quickly add content that I dreamed up, and all the relevant code stayed with the object that it related to - for instance, the special behavior of a flaming sword was kept with the flaming sword object; there was no special code to handle that special case in the combat code. This made my code easy to manage and navigate, which paid dividends for maintenance and bug hunting.
If you haven't looked into it, I *highly* recommend looking into object oriented design. It's not a silver bullet for every single application of programming practices, but it makes certain types of applications very easy to write, and one of those types of applications is the RPG, where you need to model lots of things that behave in similar ways but which still require significant variation (players, monsters, spells, attacks, weapons, armor, potions, etc.). _________________ Visit the Sacraments web site to play the game and read articles about its development.
|
|
Back to top |
|
 |
Tenshi Everyone's Peachy Lil' Bitch

Joined: 31 May 2002 Posts: 386 Location: Newport News
|
Posted: Thu Oct 12, 2006 10:48 pm Post subject: |
[quote] |
|
- The only way I can explain mine concisely is to say that Players and Enemies are Characters. All characters can perform particular actions. The "User" can interact with each Player set their behavior, and scripts (and FSM's) dictate enemy behavior. From there, all actions are fundamentally Character based. Everything is based on Active Time. _________________ - Jaeda
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|