 |
|
|
 |
|
View previous topic - View next topic |
Author |
Message |
Captain Vimes Grumble Teddy
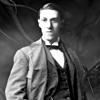
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Fri Apr 20, 2007 5:26 pm Post subject: DevX, Are you there? |
[quote] |
|
Because I need help again.
WARNING MASSIVE CODAGE WARNING
Code: | //This is the basic SDL sprite-movement program
//SDLbasicmove
#include <SDL>
#include <stdio>
//The sprite's x and y velocity and position
int xPos = 562, yPos = 384;
int xVel = 0, yVel = 0;
int DetermineEventType(SDL_Event event)
{
//This function returns an integer value corresponding to the type of event passed
//through SDL_Event event.
if(event.type == SDL_KEYDOWN)
{
//A key has been pressed
return 0;
}
else if(event.type == SDL_KEYUP)
{
//A key has been released
return 1;
}
else if(event.type == SDL_QUIT)
{
//Time to quit
return 2;
}
//This should never happen, but just in case
return 3;
}
void HandleKeyPress(SDL_KeyboardEvent key)
{
//Handle the key presses
switch(key.keysym.sym)
{
case SDLK_LEFT:
xVel = -1;
break;
case SDLK_RIGHT:
xVel = 1;
break;
case SDLK_UP:
yVel = -1;
break;
case SDLK_DOWN:
yVel = 1;
break;
}
return;
}
void HandleKeyRelease(SDL_KeyboardEvent key)
{
//Handle the key releases
switch(key.keysym.sym)
{
case SDLK_LEFT:
if(xVel <0> 0)
{
xVel = 0;
}
break;
case SDLK_UP:
if(xVel <0> 0)
{
yVel = 0;
}
break;
}
return;
}
int main(int argc, char* argv[])
{
//Initialize the SDL video subsystem
SDL_Init(SDL_INIT_VIDEO);
//Create the screen surface
SDL_Surface* pScreen = SDL_SetVideoMode(800, 600, 0, SDL_ANYFORMAT);
//Create the character sprite surface
SDL_Surface* pSprite = SDL_LoadBMP("sprite.bmp");
//Set the sprite bitmap to use pink (255, 0, 255) as a transparent color
SDL_SetColorKey(pSprite, SDL_SRCCOLORKEY, SDL_MapRGB(pScreen->format, 255, 0, 255));
//The source and destination rects
SDL_Rect srcRect, dstRect;
srcRect.w = pSprite->w;
srcRect.h = pSprite->h;
srcRect.x = 0;
srcRect.y = 0;
dstRect = srcRect;
//The event storage
SDL_Event event;
//Start the message pump
for(;;)
{
//Check for the type of message
int iMsgType = DetermineEventType(event);
//Handle the message
switch(iMsgType)
{
case 0:
//The key has been pressed
HandleKeyPress(event.key);
case 1:
//The key has been released
HandleKeyRelease(event.key);
case 2:
//The program is quitting
SDL_Quit();
return 0;
case 3:
//There was an error
printf("Error alert.");
SDL_Quit();
return 0;
}
//Clear the screen
SDL_FillRect(pScreen, NULL, 0);
//Place the sprite
SDL_BlitSurface(pSprite, &srcRect, pScreen, &dstRect);
//Move the sprite
xPos += xVel;
yPos += yVel;
//Update the screen
SDL_UpdateRect (pScreen, 0, 0, 0, 0);
} //End the pump
SDL_Quit();
return 0;
} |
Sorry about the massive codage, but I need help... the window appears for a split-second but then exits out, and that's after I seriously messed with it and placed all the key handlers in separate functions. Before it just didn't move the sprite.
HEEEEEEEEEEEEEEEAAAAAAAAAAAAAAALLLLLLLP
Are you ready?! 3 2 1 GO!
*launches into uber-hard mode Elite Beat Agents to the tune of Walkie-Talkie Man*
I have driven myself insane trying to figure out what this is. The function-separation was the last thing I tried. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
Scrim Mandrake's Little Slap Bitch

Joined: 05 Apr 2007 Posts: 69 Location: Canada
|
Posted: Fri Apr 20, 2007 5:59 pm Post subject: |
[quote] |
|
I'm not an SDL expert, but if your program is dying a moment after it starts to run, that might mean the problem is not in your event handling code but actually in the SDL initialization. Are you sure it's correct? Perhaps your "sprite.bmp" file isn't loading properly or something similar.
You can always try the old trick of putting printf("Made it here!") at various stages in your code to determine how far it is getting before it dies.
Likewise, if it is initializing properly, you can then figure out if the problems is in your main loop or in one of the functions.
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Fri Apr 20, 2007 6:04 pm Post subject: |
[quote] |
|
Several problems with this code:- You are including "SDL" and "stdio". The correct headers are "SDL.h" and either "cstdio" (in C++) or "stdio.h" (in C).
- You are not actually reading events anywhere. Use SDL_PollEvent or SDL_WaitEvent.
- You are using numeric literals when you should be using named constants. Instead of 0, use SDL_KEYDOWN. Instead of 1, use SDL_KEYUP. Instead of 2, use SDL_QUIT.
- You are not handling some important event types, such as SDL_ACTIVEEVENT and SDL_MOUSEMOTION. It is OK to ignore these events, but they are not errors and should not be treated as such.
- Your key release handler is badly broken. You are not handling the relase of SDLK_RIGHT or SDLK_DOWN, and "xVel <0> 0" is almost certainly not what you meant.
- You don't have any timing code, so your sprite will move at different speeds on different computers.
- You are missing the error checking on SDL_Init, SDL_SetVideoMode, and SDL_LoadBMP.
- You are not converting the sprite into the pixel format of the screen. This is bad for performance, but more importantly, it means that you should not use the screen pixel format for setting the color key.
- You are not freeing the sprite before quitting. This is poor form, but it should not actually break anything.
|
|
Back to top |
|
 |
DeveloperX 202192397
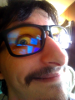
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Apr 21, 2007 2:48 am Post subject: |
[quote] |
|
{edited}
I know why its closing.
TWO reasons jump at me, now that I've spent more than 5 seconds looking at the code.
reason 1. you are assuming that anything other than SDL_KEYDOWN, SDL_KEYUP, or SDL_QUIT are not supposed to happen.
you return 3 as a default in DetermineEventType()
you fail to realize that NO events are being reported as "3" so, you're closing the program in main() when you call SDL_Quit for case 3
reason 2. your switch / case section in main() is poorly written.
you should have a break; statement for each case.
concept
Code: |
switch (value)
{
case 0:
{
handlenull();
} break;
case 1:
{
handleone();
} break;
case 2:
{
handletwo();
} break;
default:
{
handledefault()
} break;
} // end switch
|
hope that helped
:) _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
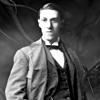
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Tue Apr 24, 2007 12:01 pm Post subject: |
[quote] |
|
Oh god... after reading your guys' posts I feel like such an idiot... durrrh. Thank you. It's working now. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
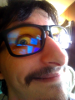
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Apr 24, 2007 5:54 pm Post subject: |
[quote] |
|
Maestro134 wrote: | Oh god... after reading your guys' posts I feel like such an idiot... durrrh. Thank you. It's working now. |
Don't feel like an idiot. Its easy to get lost/mixed up when you're just starting to learn something new. God knows I had a hell of a time learning Win32/DirectX stuff years ago....*shudders*
Let me know if you need anything else man. I'm always happy to help. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|
|