 |
|
View previous topic - View next topic |
Author |
Message |
Hajo Demon Hunter
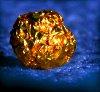
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Thu Aug 02, 2007 3:42 pm Post subject: Efficient capitalizing of first letter of a word in Java? |
[quote] |
|
I use to store all item names and similar lowercase, since most often they appear in the middle of a sentence, where they are needed lowercase.
But sometimes I should capitalize the first letter, if the word stands alone or at the beginning of a sentence.
I found code like this:
String firstLetter = word.substring(0,1);
String rest = word.substring(1);
String capitalizedWord = firstLetter.toUpperCase() + rest;
Now this works, but it generates some objects as side effect, which the garbage collector has to clean up later. And one of the places where I need to capitalize words is in the main display loop. I certainly do not want to generate a lot of garbage objects in the main display loop.
Does someone know a better solution? Any help will be greatly appreciated. If everything else fails, I'll keep the words twice in memory, lowercase and capitalized.
|
|
Back to top |
|
 |
Verious Mage

Joined: 06 Jan 2004 Posts: 409 Location: Online
|
Posted: Thu Aug 02, 2007 4:40 pm Post subject: |
[quote] |
|
You could always write the code as:
Code: | String capitalizedWord = word.substring(0,1).toUpperCase() + word.substring(1).toLowerCase(); |
This should eliminate a couple variables and somewhat reduce garbage collection.
From a performance point of view, it might be better to store the names as mixed (proper) case and then simlply convert to lowercase where necessary, since this would only require one function call instead of four (two substrings and two change case).
|
|
Back to top |
|
 |
Hajo Demon Hunter
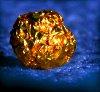
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Fri Aug 03, 2007 8:46 am Post subject: |
[quote] |
|
Verious wrote: |
From a performance point of view, it might be better to store the names as mixed (proper) case and then simply convert to lowercase where necessary, since this would only require one function call instead of four. |
Sometimes I feel a bit blind. Thanks for opening my eyes :)
The capitalized version is needed every frame, the lowercase only in the message log. So your suggestion fits much better into the structure. Thanks!
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|