 |
|
View previous topic - View next topic |
Author |
Message |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Sat Dec 15, 2007 11:11 am Post subject: Mac porting hints! |
[quote] |
|
FYI, I am a new owner of a Mac, and as such, I had decided to make a port my game to the Mac! I thought I would share some of the things that I learned when doing the port, which may serve as some useful information for C++ programmers wishing to do so as well.
The time it took for porting was about 45 mins for a C++ project with about ~20000 lines of code. Now, the amount of time I took isn't spectacular considering the hours of prep work has been done over time in maintaining the source code to be portable.
One of the things that helped big time was using CMake as the build system. CMake is a cross platform build system that can generate project files for your platform. On Windows, it can generate Microsoft Visual Studio Solution files or MinGW/Cygwin makefiles; on Linux it generates KDevelop or unix makefiles; and on Mac it can generate Xcode project files. For my Mac, I just downloaded the Mac version of CMake, ran it, and it produced a Xcode file which I just double clicked. Once the Xcode editor was open, everything was already setup and all I had to do was click "Build" to start compiling.
I think one of the most interesting topics about the Mac development is: How compatible is Linux to Mac, and vice versa. Here's what I have discovered. There appears to be two ways of building programs. One way is the UNIX compatible way, which involves using the ./configure and friends to build programs. These link with UNIX compatible libraries and I found that this compiles most Linux command line and X11 apps without major problems. The only annoyance is that all GUI apps built this way launches the X11 server (which you will be prompted that X11 will start) and an empty terminal on startup. The other way is building apps with Xcode. This way seems to build native Mac apps which links to the Mac libraries as opposed to the UNIX compatible ones. I don't think I was able to get any X11 apps compiled this way since Xcode decided not to include X11 headers and libs when compiling in Xcode. A few things in Xcode have changed, such as header file names like GL/gl.h, GL/glut.h have changed to OpenGL/gl.h, GLUT/glut.h respectively.
Linking OpenGL code (in Leopard) will also produce an error:
Code: | ld: cycle in dylib re-exports with /usr/X11R6/lib/libGL.dylib
collect2: ld returned 1 exit status |
However, searching the net provides the solution of adding the following linker flags:
Code: | -dylib_file \
/System/Library/Frameworks/OpenGL.framework/Versions/A/Libraries/libGL.dylib:\
/System/Library/Frameworks/OpenGL.framework/Versions/A/Libraries/libGL.dylib |
If you link SDL, you must also add SDLMain.m (distributed with the Mac version of SDL) to your project or your program will crash at runtime.
Macs also include a ton of software development libraries preinstalled including OpenAL for sound or wx-widgets for building GUI.
For built executables, Macs have a concept of bundles. This is the packaging of all your executables and data files into one directory and having the Mac finder treat it all as one app file. This allows users to install your program by dragging it to anywhere and deleting it by dragging it to the trash. Too use bundles effectively, you must references all your data files through the use of Mac's bundle API. Bundles also allow you to turn Java JAR files into an app. Since Java is included with all Macs, now Java apps will look like any mac app and no one will know that it's Java (and complain about it's speed). This makes Java quite a worthy language to be developing for.
I think the bulk of the work of getting a successful Mac port is sticking strictly with code portability rules. This would mean coding strictly to the C++ standards and refraining from using compiler specific extensions. It is a good idea to develop using the GCC compiler only as it is the default compiler for Apple and it is available on most platforms. Other than that, there isn't much too special about getting it to work with Mac.
(Note that since I am a new developer to the Mac, the above information are of my own findings and may not be entirely accurate. Feel free to point out the inconsistancies.)
|
|
Back to top |
|
 |
Scrim Mandrake's Little Slap Bitch

Joined: 05 Apr 2007 Posts: 69 Location: Canada
|
Posted: Sat Dec 15, 2007 3:12 pm Post subject: |
[quote] |
|
This thread is a nice idea.
Here's one small tip that Bjorn helped me out with when I was trying to make a windows version of my contest entry (I wrote it on mac originally). Porting it was no biggie because I wrote it in python, but I did run into some problems: namely, copying plain-text files from one platform to the other.
Windows and Mac use a different format for their line breaks: Windows uses two control characters where Mac (and most unix-like systems) uses just one. This means copying text files (in my case, source code and various game configuration files) from one platform to the other can create a mess.
When I moved the above files to windows I discovered that all the line breaks were "missing" and my entire source file was one line... If you are porting in the other direction you'll find all your stuff has an extra character (usually shown as ^M if I recall) at the end of every line.
Two solutions:
1) Use ftp to move your files from mac to windows (or vice-versa). It's smart enough to convert the linebreaks.
2) Use these handy command line tools:
dosunix INPUTFILE OUTPUTFILE
unixdos INPUTFILE OUTPUTFILE
They do the conversion as well. In my case I used a little shell script to make windows versions of my various files:
for i in `ls *.txt`
do
unixdos $i ../windows/textfiles/$i
done
If you are porting the other direction you'd use "dosunix" instead.
I don't think unixdos and dosunix are installed on OSX by default, but you can download them easily enough. I use FINK, which is a nice debian-style package management ap for installing various open-source tools on the Mac.
|
|
Back to top |
|
 |
DeveloperX 202192397
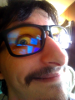
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Mon Dec 17, 2007 4:25 am Post subject: |
[quote] |
|
A solution I use for handling that is to open the files in Wordpad and immediately click the save button.
It puts the right newline characters in the file for windows.
Now, remember that is WORDPAD Not Microsoft Word.
Word will mess your code up badly.
If you cannot find Wordpad, go to start->Run, type "write" and then hit enter.
Simple solution to an annoying problem.
Don't have windows? Get a VMWare setup on whatever you use, and get a copy of windows 98 or xp to install in the vm to quickly do your tasks. Or, if that feels too much like piracy, then just walk into a computer store like bestbuy with your files on a usb pendrive, and use their windows computer to make your conversions. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Mon Dec 17, 2007 6:46 am Post subject: |
[quote] |
|
Ahh yes, python is pretty strict with the newlines and formatting. For C/C++ source code, it is better to use UNIX line feeds even on windows because windows compilers can work with UNIX line feeds, but UNIX compilers don't like windows line feed.
If you don't have dos2unix or unix2dos but you have perl:
dos2unix in perl:
Code: | perl -pi -e 's/\r\n/\n/' file.txt |
unix2dos in perl:
Code: | perl -pi -e 's/\r?\n/\r\n/' file.txt |
This reminds me that another thing to look out for between windows and macs, is filename sensitivity. Mac and other UNIX systems, use case-sensitive filenames. So it is a good idea to reference files exactly as they are named. It is also good idea to use a forward slash (/) to separate directories. It seems that all systems windows, mac, and linux understand / as the directory separator, but only windows understands backslash (\).
|
|
Back to top |
|
 |
DeveloperX 202192397
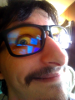
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Thu Dec 20, 2007 10:50 pm Post subject: |
[quote] |
|
More tips:
Always use lowercase filenames & extensions.
Never use spaces in filenames or directory names.
Never use odd characters such as %()[]{}'`~!@ ..etc
Try to keep directory trees to 2 levels or less.
eg:
Code: |
game/game (executable .exe on windows)
game/data/graphics/ (two levels deep from the executable)
game/data/music/
game/data/sounds/
game/data/scripts/
etc..
|
_________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|