View previous topic - View next topic |
Author |
Message |
Captain Vimes Grumble Teddy
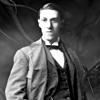
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Fri Sep 12, 2008 2:08 pm Post subject: Speed issues |
[quote] |
|
Okay, so I finished Space Invaders a while ago, like I said. It ran at normal speed on my crappy home computer, but when I took it to the school's better computers it was blindingly fast.
What is happening? I assume it's because I use SDL_GetTicks() to regulate game cycle times and the computers measure ticks at different rates, but how do I fix it so that the game runs at the correct speed on all computers? _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
Hajo Demon Hunter
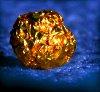
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Fri Sep 12, 2008 3:40 pm Post subject: |
[quote] |
|
SDL_GetTicks gets the number of milliseconds since the SDL library initialization.
Milliseconds should be the same on each computer ;) More seriously, I used this for timing in a former project of mine and it worked for me.
I think the problem must be elsewhere.
|
|
Back to top |
|
 |
DeveloperX 202192397
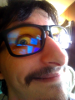
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Fri Sep 12, 2008 9:21 pm Post subject: |
[quote] |
|
hmm
you want to move your stuff by a certain amount of pixels over a certain amount of time, the same on old computers as on faster computers.
well try something like this.
Code: |
float deltaTime = 0.0f;
int currentTime = 0;
int lastTime = 0;
// main game loop
while(1)
{
currentTime = SDL_GetTicks();
deltaTime = (float)(currentTime - lastTime) / 1000;
lastTime = currentTime;
// assume we have this sprite to move
// both position and speed vars are floats
player.x += player.speedX * deltaTime;
player.y += player.speedY * deltaTime;
// call it to be drawn using a fictional
// routine
Draw_Sprite(backBuffer,
playerImage,
(int)player.x,
(int)player.y);
SDL_Delay(1);
}
|
goodluck _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
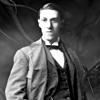
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Fri Oct 10, 2008 1:27 pm Post subject: |
[quote] |
|
Okay, I'm back. Sorry for not posting. I've been extremely busy in my computer classes, which is the only place where I have access to the internet.
Anyway, I see that you're calculating the difference between the total time and time since the last cycle, but why are you dividing by 1000? And how does this regulate game speed? I've never seen SDL_Delay() before, either. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
Hajo Demon Hunter
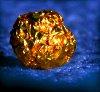
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Fri Oct 10, 2008 2:19 pm Post subject: |
[quote] |
|
SDL_Delay(1) is there to be multitasking friendly, to give CPU time to other programs.
The divide by 1000 just converts microseconds to seconds, I think. that does not really change the logic for calculating moves based on time, just the precision of timing. I used to use milliseconds directly in my projects, one of last ones was near-realtime and even a jitter of a few milliseconds was noticeable, so I wanted it as precise as possible.
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Fri Oct 10, 2008 6:32 pm Post subject: |
[quote] |
|
The SDL_Delay also helps reduce your program from consuming 100% CPU and making it really hot (my macbook CPU gets up to about 80 ºC/176 ºF).
|
|
Back to top |
|
 |
DeveloperX 202192397
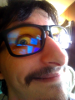
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
|
Back to top |
|
 |
Hajo Demon Hunter
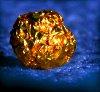
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Mon Oct 13, 2008 8:45 am Post subject: |
[quote] |
|
Sometimes it might be simpler to try to keep a fixed framerate. That is less versatile than the approach DevX proposed, but sometimes simpler, particularly if the target computers are comparably fast and your game is simple.
It'd look something like this
Code: |
int frametime = 100; // Desired time per frame in milliseconds
while(true) {
int t0 = current_time_millis(); // Current time in milliseconds
do_stuff_for_one_frame(); // Your game's actions in here
int t1 = current_time_millis(); // Second timer point, to find out how long stuff took
sleep(frametime - (t1 - t0)); // Sleep for the rest of the frametime
}
|
This will make your code run at 10 FPS, on all computers that are fast enough to achieve 10 FPS with your game, and on the others will just run slower.
That isn't quite as nice as DevX proposal, but also works to some extend.
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
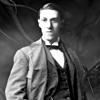
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Thu Oct 16, 2008 11:50 am Post subject: |
[quote] |
|
DevX wrote: | So...how is your game coming along?
Got any links for beta testing? or screenshots? |
Sorry, not yet. I've made the mistake of trying to code a full game before I'm ready for it before, and this time I'm trying to get everything figured out and set up before moving on to a specific project. The next one just looks to be a simple platformer, though, along the lines of Mario.
@Hajo: that code looks perfect, actually. That's what I was looking for. Thanks.
DevX, with your code, I see this...
Code: | Declare three variables for time
while(always)
{
get the current time
change in time = current time - last time converted into seconds
last time = current time
do stuff
delay for a bit
} |
So there's no point in changing the value of the variables that count time, since they're not used for anything. Or am I missing something glaringly obvious? Please explain.[/code] _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
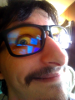
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Thu Oct 16, 2008 2:01 pm Post subject: |
[quote] |
|
Captain Vimes wrote: |
So there's no point in changing the value of the variables that count time, since they're not used for anything. Or am I missing something glaringly obvious? Please explain. |
Yes the value is used to scale the amount to move your objects so that no matter what speed your computer is running at, your objects will take the same amount of time to travel the distance that you want. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
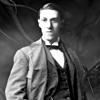
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Thu Oct 16, 2008 11:23 pm Post subject: |
[quote] |
|
How? The deltaTime, totalTime, and currentTime aren't passed to anything. There's only one time-related function as far as I can see, and that's SDL_Delay(), which you're passing a 1 to.
Please explain! I'm sorry if I'm getting on your nerves, but I am new to this. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
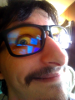
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Fri Oct 17, 2008 1:59 pm Post subject: |
[quote] |
|
Captain Vimes wrote: | How? The deltaTime, totalTime, and currentTime aren't passed to anything. There's only one time-related function as far as I can see, and that's SDL_Delay(), which you're passing a 1 to.
Please explain! I'm sorry if I'm getting on your nerves, but I am new to this. |
Its no problem. You're not getting on my nerves. I enjoy teaching.
If you join NOGDUS (my group) #nogdus @ irc.afternet.org I'd be more than happy to help you out when I can.
OK, look at this snippet of code from above again:
Code: |
currentTime = SDL_GetTicks();
deltaTime = (float)(currentTime - lastTime) / 1000;
lastTime = currentTime;
// assume we have this sprite to move
// both position and speed vars are floats
player.x += player.speedX * deltaTime;
player.y += player.speedY * deltaTime;
// call it to be drawn using a fictional
// routine
Draw_Sprite(backBuffer,
playerImage,
(int)player.x,
(int)player.y);
|
the part that is being updated by scaling by deltaTime is the player's position.
player.x += player.speedX * deltaTime;
player.y += player.speedY * deltaTime;
THAT is where it is being used.
If that doesn't make sense, then I can always make a demo to show the different between using that code and not.
(run the demo on more than one computer to see the effects)
goodluck, and let me know if there is anything you need.
If I'm not on #nogdus (I am w1nt0p on there) then simply email me. ccpsceo@gmail.com _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
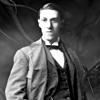
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Wed Oct 22, 2008 2:46 pm Post subject: |
[quote] |
|
*facepalms*
Oohhh. I get it now. Thanks much, DevX. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
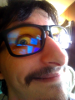
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Fri Oct 24, 2008 1:30 pm Post subject: |
[quote] |
|
Captain Vimes wrote: | *facepalms*
Oohhh. I get it now. Thanks much, DevX. |
:)
happy to help!
good luck with your learning.
And let me know if there is anything else I can do for ya. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |