View previous topic - View next topic |
Author |
Message |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Wed Jun 18, 2003 3:50 am Post subject: Inheriting STL Classes |
[quote] |
|
I want to create a class which will manage my event flags; I was thinking the best way to store the data would be to use a std::vector . At first, I thought I'd implement it with inheritance, but then I decided it would be simpler and more sensible just to use composition. However, I still would like to know if my inheritance code would make sense, for future reference.
Code: | class EventList : public std::vector <bool> {
public:
void aFunc ( bool );
};
void EventList::aFunc ( bool aFlag ) {
// Here I create a new element based on the argument.
this -> push_back ( aFlag ); // does this make any sense?
// And here I access that new element.
bool anotherFlag = * this [ 0 ]; // and does this make sense?
} |
Well, does that make sense? I'm not exactly sure why it shouldn't, it just seems really odd and kludgey to me. Thanks for your help.
P.S., I'm allowed to ask straight programming questions here, right? It is for an RPG game, at least.
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
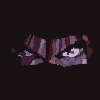
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Wed Jun 18, 2003 4:11 am Post subject: |
[quote] |
|
Heh, I wouldn't use inheritance, I'd just have a member variable be a vector. If you're going to use inheritance, it should be because you want to rewrite some of vector's code, which is polymorphism. Since you don't have the original source to it anyway, it's kinda pointless.
|
|
Back to top |
|
 |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Wed Jun 18, 2003 4:51 am Post subject: Composition Is Good |
[quote] |
|
Yes, I said I'd use composition, i.e., use of a member variable. My actual code looks something like this:
Code: | class EventList {
private:
std::vector <bool> events;
public:
bool operator[] ( int index ) {
return events [ index ];
}
// all that other junk
}; |
I was just curious about whether the previous code is kosher. I'd have tested it out myself, but just because something "works" doesn't mean that it isn't hiding serious design flaws.
Oh, and I've heard some stuff about an STL bit_vector, being basically a souped-up vector, and that vector is sometimes implemented as a bit_vector, something, something. Anyone have any light to shed on this?
|
|
Back to top |
|
 |
DrV Wandering Minstrel

Joined: 15 Apr 2003 Posts: 148 Location: Midwest US
|
Posted: Wed Jun 18, 2003 5:01 am Post subject: |
[quote] |
|
Hmm... in Perl, a bit vector is just a string of integers that can be modified bit by bit, just like you had done something like but I don't know if that's the same thing... _________________ Don't ask no stupid questions and I won't send you away.
If you want to talk fishing, well, I guess that'll be okay.
|
|
Back to top |
|
 |
Guest
|
Posted: Wed Jun 18, 2003 5:13 am Post subject: |
[quote] |
|
No! You should not inherit for multiple reasons
1: inhertiance implies an "Is-A" relationship
what this means is that if you would inherit your event list
from vectro everything that's valid on such should
be valid on the EventList and I figoure this is hardly the case
2: STL classes aren't designed to be inherited from
Any class designed to inherit from have both of theese:
a) a virtual destructor
b) at least 1 virtual memberfunction
STL classes have none of theese making them ill suited for
inhertiance opening the door for "undefined behaviour"
so just don't.
You should always use the weakest relationship between classes
possible, and apart from friends inhertiance is as strong as they
come.
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
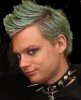
Joined: 29 May 2002 Posts: 559
|
Posted: Wed Jun 18, 2003 5:13 am Post subject: |
[quote] |
|
And that was me... _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Wed Jun 18, 2003 6:44 am Post subject: Composition Is Still Good |
[quote] |
|
Gee, some people sure are scary when their OOP is threatened. Heh heh.
Anyway, like I said, I decided to use composition instead of inheritance. I thought of inheritance first, but then I realized I only wanted a couple functions, and was scared of opening up the whole interface (since EventList "Has A" vector of bools, but "Is Not A" vector of bools itself).
But you're right, I never should have considered inheriting from an STL member in the first place.
And, um, what's a virtual constructor? That sounds profoundly mad.
And DrV, thanks for actually reading my post, unlike some people who skip straight to the code block (heh). Yes, I think that's exactly the idea behind a bit_vector, although I'm talking about an STL version (which I've just found: http://www.sgi.com/tech/stl/bit_vector.html, so no one worry about it anymore).
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
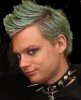
Joined: 29 May 2002 Posts: 559
|
Posted: Wed Jun 18, 2003 8:36 am Post subject: |
[quote] |
|
I never said virtual constructor there's no such thing (altough it's often usefull to mimic that behavoir) I said virtual destructor
not having a virtual destructor in any class you inherit from
is a really bad thing the best thing that can happen is a memory
leak... the worst... well it's undefined behavoir so mostly anything.
the problem arise when you try do delete a derived object
through a base class pointer, the wrong destructor gets called
and I think we all can agree that's a really bad thing
but on the topic of virtualizing constructors that is often achived through
an abstract base and something called a factory _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Wed Jun 18, 2003 2:53 pm Post subject: |
[quote] |
|
DrunkenCoder wrote: |
not having a virtual destructor in any class you inherit from
is a really bad thing the best thing that can happen is a memory
leak... the worst... well it's undefined behavoir so mostly anything.
|
Actually it's perfectly safe as long as you don't try to delete the object through a pointer to the base class.
|
|
Back to top |
|
 |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Wed Jun 18, 2003 8:23 pm Post subject: Destructors |
[quote] |
|
Sorry; I always seem to say constructor when I mean destructor.
Oh, I see. I'd thought that the correct destructor would automatically get called, even if you're dealing with a pointer to a base class. That's scary; I'll go fix that now, thanks.
Now, in the following:
Code: | class ClassA {
~ClassA () { /* whatever */ }
};
class ClassB : public ClassA {
~ClassB () { /* whatever */ }
}; |
and you create an instance of ClassB, classBInstance, when classBInstance goes out of scope, first ClassB's destructor get's called, and then ClassA's (right?). If you make ClassA's destructor virtual, does that mean that ClassB then has to take care of ClassA's clean-up?
|
|
Back to top |
|
 |
grenideer Wandering Minstrel

Joined: 28 May 2002 Posts: 149
|
Posted: Wed Jun 18, 2003 9:00 pm Post subject: c++ |
[quote] |
|
No. There's no downside to making a destructor virtual (except overhead). Making a destructor (or any class function) virtual makes it 'smart' (dynamic). That means at RUN-TIME the program decides which destructor (or other function) to call. If you are using a pointer to a base class that is pointing to a child class, then although the pointer is of type *base class*, the destructor is smart and sees that it is actually pointing to a *child class* and calls that destructor instead.
Rainer, although you're right about using a non-virtual destructor being safe (actually the same thing DrunkenCoder said), it REALLY is bad style. And covering your a$$ beforehand is the best way to stay outta trouble. If later on down the line you use a base class pointer (or say, someone else on your team of coders [since we're talking in terms of game-programming]) , that'll be a very hard bug to catch. _________________ Diver Down
|
|
Back to top |
|
 |
grenideer Wandering Minstrel

Joined: 28 May 2002 Posts: 149
|
Posted: Wed Jun 18, 2003 9:20 pm Post subject: c++ |
[quote] |
|
Jihgfed, to answer your original question, the first line of code you were wondering about makes sense (using the push_back() function). That second line may or may not, depending on whether or not you overloaded the [] operators. Can your EventList class objects access elements with the brackets? If they can, then the line works. Although I'm assuming you're gonna do something else with that bool you caught- otherwise it's wasted.
Um, yeh, to add to Ninkazu's original reply (that the reason to use inheritance is to overload member functions) I just wanted to state the second reason to use inheritance - which is to directly access private or protected members of the base class. And I agree with everyone else (even you) that there's no reason inheritance should ever have been considered in this case. _________________ Diver Down
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
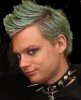
Joined: 29 May 2002 Posts: 559
|
Posted: Wed Jun 18, 2003 9:58 pm Post subject: |
[quote] |
|
Quote: | Actually it's perfectly safe as long as you don't try to delete the object through a pointer to the base class. |
and I also clarified that in my second post.[/quote] _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
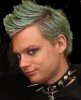
Joined: 29 May 2002 Posts: 559
|
Posted: Wed Jun 18, 2003 10:02 pm Post subject: |
[quote] |
|
to nitpick on greindeers post.
Inheriting doesn't give access to private data in the base only to protected.
And as everyone seems to be in a really nitpicky mode I can also clearify that mostly when we talked about inheritance here we have ment *public* inhertiance, don't forget the more esotroic flavours of protected and even private inhertance although not common they do have uses. _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Thu Jun 19, 2003 12:34 am Post subject: operator[] for EventList |
[quote] |
|
Okay, thanks a lot, everybody. I have one more (hopefully, one last) question.
I'm trying to set up my operator[], but I'm having difficulty.
Code: | class EventList {
vector<bool> events;
bool & operator[] ( int index ) {
return events [ index ];
}
}; |
That what I've got, but it won't compile. I use two different compilers, so you get your choice of error message:
MingW wrote: | could not convert 'std::vector::operator[] (unsigned int) [with _Alloc = std::allocator] (index )' to 'bool &' |
Borland Command-Line Compiler wrote: | Reference initialized with 'bool', needs lvalue of type 'bool' in function EventList::operator[] (int) |
It compiles fine if I do not return the value by reference, or if "events" is a normal array instead of a vector. So, I guess the problem is that indexing vector doesn't return a "real" lvalue, or something? Maybe?
Sorry I'm asking so many questions, but I really do appreciate the help.
|
|
Back to top |
|
 |