 |
|
View previous topic - View next topic |
Author |
Message |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Fri Jul 04, 2003 1:44 pm Post subject: C Questions |
[quote] |
|
Well, I've been messing around in C for a while now, and I have some non-googlable (or at least, very difficult to google (I just love using google as a verb)) questions, and I'd be grateful to anyone who could shed some light on them.
1. Looking at source, I've noticed two very different approaches to choosing variable types. Some people just use ints for regular numbers, floats for real numbers, and chars for characters, while others are careful about picking shorts, longs, doubles, w_chars, what-have-you. I've heard that ints are faster (while the others are obviously more space-efficient), but is does it make enough a difference either way that I should worry about optimizing?
2. Why are functions like "exit ()", "abort ()", and those <stdarg> functions in the library, instead of in the language itself? Would it actually be possible for me to duplicate these functions myself (if, that is, I knew what I was doing)?
3. Where are NULL, EXIT_SUCCESS, and EXIT_FAILURE defined? I seem to get them whenever I include any standard library header, so it's not a priority, but I would like to know where they're actually kept in case I ever need to change the value of NULL (yes, that's a joke).
4. I have only the vaguest idea of what a "profiler" is; where can I learn more?
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Fri Jul 04, 2003 3:46 pm Post subject: Re: C Questions |
[quote] |
|
Jihgfed Pumpkinhead wrote: |
1. Looking at source, I've noticed two very different approaches to choosing variable types. Some people just use ints for regular numbers, floats for real numbers, and chars for characters, while others are careful about picking shorts, longs, doubles, w_chars, what-have-you. I've heard that ints are faster (while the others are obviously more space-efficient), but is does it make enough a difference either way that I should worry about optimizing? |
Using "packed" data types can be important when dealing with large arrays, such as tile maps. A 200x200 map with 4 layers of 'int' takes 625 KB while a map of the same dimensions of 'char' obviously only takes one fourth of that. In most other cases, just use 'int'.
Quote: |
2. Why are functions like "exit ()", "abort ()", and those <stdarg> functions in the library, instead of in the language itself? |
Because it keeps the language simple, and because those functions are abominations that should never be used.
Quote: | Would it actually be possible for me to duplicate these functions myself (if, that is, I knew what I was doing)? |
Not in portable C.
Quote: |
3. Where are NULL, EXIT_SUCCESS, and EXIT_FAILURE defined? I seem to get them whenever I include any standard library header, so it's not a priority, but I would like to know where they're actually kept in case I ever need to change the value of NULL (yes, that's a joke). |
<stdlib.h>?
|
|
Back to top |
|
 |
valderman Mage
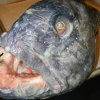
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Fri Jul 04, 2003 4:58 pm Post subject: |
[quote] |
|
1: When porting code, ints are generally faster, since the compiler can make them whatever size the target CPU likes best.
4. A profiler is (if I got this right) a program which checks which parts of your code needs optimizing (takes the longest time to run). _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
BigManJones Scholar
Joined: 22 Mar 2003 Posts: 196
|
Posted: Fri Jul 04, 2003 6:29 pm Post subject: |
[quote] |
|
Quote: | 1. Looking at source, I've noticed two very different approaches to choosing variable types. Some people just use ints for regular numbers, floats for real numbers, and chars for characters, while others are careful about picking shorts, longs, doubles, w_chars, what-have-you. I've heard that ints are faster (while the others are obviously more space-efficient), but is does it make enough a difference either way that I should worry about optimizing?
|
I've noticed this too. Most of the people I've seen being really picky about data types are 1) old timers from the 386/486 days 2) REAL old timers from the atari st/amiga days and 3) GBA coders (at least what little gba code i've seen indicates you are twiddling bits quiet frequently.)
Me personally, I'm not concerned with speed at all since I merely use libs made (and optimized) by other people; ie allegro. Any code I write will definitly be in the 90% thats least used compared to the 10% most often executed in the drawing routines.
|
|
Back to top |
|
 |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Sun Jul 06, 2003 9:18 am Post subject: Satiation of Curiosity |
[quote] |
|
Thanks, everybody, for sating my curiousity... well, almost.
Rainer, why is exit () bad style? I use it whenever I bump into a fatal error. Should I not be?
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Sun Jul 06, 2003 3:16 pm Post subject: |
[quote] |
|
There are two problems with 'exit':
1. You still have to perform clean-up. You can do it manually before calling 'exit', or you can use 'atexit', but both options are somewhat messy and error-prone.
2. There's no way to "catch" an 'exit'. If you later decide that you just want to return to the main menu instead of exiting the program entirely, you'll have to rewrite a lot of code.
In C++, exceptions are definitely better than 'exit'. In C, the situation is less obvious, but I'd still avoid 'exit' where possible.
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
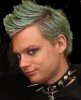
Joined: 29 May 2002 Posts: 559
|
Posted: Sun Jul 06, 2003 7:53 pm Post subject: |
[quote] |
|
In C you don't have exceptions, use setjmp and longjmp instead. _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Sun Jul 06, 2003 10:02 pm Post subject: |
[quote] |
|
'setjmp' and 'longjmp' don't really help with clean-up. I like those functions even less than I like 'exit'. If I was still working in C, I would probably just use special return values to indicate error conditions. Then again, there is very little that could convince me to use C at this point.
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
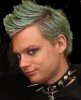
Joined: 29 May 2002 Posts: 559
|
Posted: Mon Jul 07, 2003 6:12 am Post subject: |
[quote] |
|
err, actually they do help but it requires you to think a bit, a habit not to many coders seems to have... of coures by themself they don't do anything to actually help you, but if you couple them with carfully checking your returnvalues they can be a great help _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
twinsen Stephen Hawking

Joined: 21 May 2003 Posts: 242 Location: Melbourne, Australia
|
Posted: Mon Jul 07, 2003 10:56 pm Post subject: |
[quote] |
|
Whether it be goto, exit, end, stop or whatever other BASIC function everyones talking about. I've written hundreds of C/C++ apps/games since I started programming, from boring business applications to 3D games. All I can say is I've never needed- nor missed one of these functions. If you structure your code correctly you should never need them. My two cents. _________________ Lovely girls & great prices always available at CLUB 859, 859 Glenhunly Road, Caulfield, Open 10am till late 7 days. +61-3-9523-8555. (Sorry, it was in front of me in the newspaper, I just had to use it as a signature!)
|
|
Back to top |
|
 |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Thu Jul 10, 2003 1:52 am Post subject: Error-Handling |
[quote] |
|
The more I think about it, the more I can see the value in using return values to indicate error status. But if I start doing that consistently, what do I do with functions that need to really return a value? Via arguments? Is it worth the effort learning C++ exception handling? I've managed to get by without so far, but if it'll make my life easier in the long run I'm all for it.
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Thu Jul 10, 2003 3:26 am Post subject: Re: Error-Handling |
[quote] |
|
Jihgfed Pumpkinhead wrote: | The more I think about it, the more I can see the value in using return values to indicate error status. But if I start doing that consistently, what do I do with functions that need to really return a value? Via arguments? |
In C you have a couple of options. You can use a return type that can hold all normal return values plus a special error value. You can use a global variable to hold the error state and check that each time a function returns. Or you can pass the return value out through an argument.
Quote: | Is it worth the effort learning C++ exception handling? |
If you're using C++, you should definitely use exceptions. Not only are they the best way to propagate errors across multiple function calls, but the C++ language and standard library throw exceptions that you should be able to handle. Every function that allocates memory (including 'operator new') can throw an exception. 'dynamic_cast<T&>' can throw an exception. Just about every third-party C++ librray can throw exceptions.
|
|
Back to top |
|
 |
Jihgfed Pumpkinhead Stephen Hawking

Joined: 21 Jan 2003 Posts: 259 Location: Toronto, Canada
|
Posted: Thu Jul 10, 2003 5:27 am Post subject: Yay, Exceptions. Thanks. |
[quote] |
|
Thanks, Rainer, I'll set out to learn exceptions then. C++ is just so huge (well, compared to any language with which I'm familiar) that I set about learning about it piecemeal. Now that I'm ready and have a use for it, it's time for exceptions. Hmm, I can just picture it now. No more "loadBmpCrash", "loadBmpNoCrash" to determine what to do with in case of error. Yay!
And thanks everybody for all the help here. It's been nice learning a little more about the language.
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|