 |
|
|
View previous topic - View next topic |
Author |
Message |
Adam Mage
Joined: 30 Dec 2002 Posts: 416 Location: Australia
|
Posted: Fri Nov 14, 2003 5:13 pm Post subject: Variables, the use of globals and such |
[quote] |
|
Im just wondering what you guys do about setting up the variables in your C++ engines. Do you set things all as globals or have a single class with all that in it and pass the pointer. I assume you all have a better idea than i do :D _________________ https://numbatlogic.com
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Fri Nov 14, 2003 6:45 pm Post subject: |
[quote] |
|
I tend to make things global when there's no way that I would ever want more than one of them. For the most part that's the graphics, sound effects, maps, and other game resources. I also make the game state global, just because I don't want to pass around pointers to the game state.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
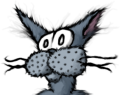
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Fri Nov 14, 2003 11:29 pm Post subject: |
[quote] |
|
Globals are nasty, nasty things. Like a hangover. Yes, that's a good analogy: Globals are a nasty hangover from C. You should attempt to rid your code of globals as much as possible.
You can mimic global variables (while adding a modicum of encapsulation and protection to your code) by throwing anything that you would have a single instance of into a singleton class. As singletons have (generally) only a single instance of themselves instantiated during a given run-time environment, they are perfect for this mimicry. You don't even have to pass pointers around to your functions: you just grab a new "instance" of the singleton (really: it's just a pointer to the "global" instance).
Globals are bad because they pollute the global namespace, and can be accessed and edited from anywhere in your code. Things could especially be nasty if you had global variables which were volatile. Bug hunting is (usually) hampered by the presence of globals, especially if you have a large object hierarchy with a high degree of interaction between those objects.
[edit]
There are two idealogies where global paranoia stem from: something that Edsger Dijkstra called "Seperation of Concerns", which mostly has to do with clear delineations between conceptual modules of a program; and the prinicple of least privilege, which in normal programs can be stated thusly: do not give a block of code more privilege than it needs to complete its task. Introducing globals into the mix violates that principle.
Also: globals break object hierarchies; if we want to be good, UML abiding programmers, we should strive to eliminate procedural handicaps. Globals are such a handicap, as they break the flow of information between objects. Objects should explicitely have to state what other objects they need to perform their duties; otherwise, they don't have a clearly defined behaviour, and thus do not have a clearly defined purpose. If an object lacks either, then why have it? There are acceptible ways (such as singletons) to ensure that object hierarchies are not broken.
[/edit] _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Sat Nov 15, 2003 5:45 am Post subject: |
[quote] |
|
LeoDraco wrote: | Globals are nasty, nasty things. Like a hangover. Yes, that's a good analogy: Globals are a nasty hangover from C. You should attempt to rid your code of globals as much as possible.
You can mimic global variables (while adding a modicum of encapsulation and protection to your code) by throwing anything that you would have a single instance of into a singleton class. As singletons have (generally) only a single instance of themselves instantiated during a given run-time environment, they are perfect for this mimicry. You don't even have to pass pointers around to your functions: you just grab a new "instance" of the singleton (really: it's just a pointer to the "global" instance).
Globals are bad because they pollute the global namespace, and can be accessed and edited from anywhere in your code. Things could especially be nasty if you had global variables which were volatile. Bug hunting is (usually) hampered by the presence of globals, especially if you have a large object hierarchy with a high degree of interaction between those objects. |
I think I disagree with you, although I can't be sure because you haven't defined what you mean by "global". Globals don't have to be placed in the global namespace. They can be made local to a file (by placing them in an anonymous namespace), local to a class (by declaring them as static member variables), or placed in a named namespace. Globals don't have to give universal write access. They can be made const, or they can be instances of a class that doesn't expose any mutating operations.
A singleton is one way to expose global data, but it isn't the only way and it's not necessarily the best way. The fkyweight pattern is another option, as is a set of standalone functions. Sometimes the simplest way - exposing the global directly - is the best. I rarely encounter problems related to global variables, and I've never encountered one that would have been fixed somehow by hiding the global behind a singleton.
When you get down to it, singletons don't offer much advantage over global variables. The variable is still there, it's still global, it can still be accessed, and the namespace is still "polluted". Singletons offer one huge advantage in C++ - you can control when they are created and, to a lesser degree, when they are destroyed - but that's only sometimes needed, and there are other ways of obtaining the same effect.
I think the original poster's question not about how best to expose global data, but about whether one should use global data at all.
|
|
Back to top |
|
 |
Adam Mage
Joined: 30 Dec 2002 Posts: 416 Location: Australia
|
Posted: Sat Nov 15, 2003 6:24 am Post subject: |
[quote] |
|
Quote: | I think the original poster's question not about how best to expose global data, but about whether one should use global data at all. |
... and if not globals, what should be used?
Im looking into singletons now, seeing how them boys work. _________________ https://numbatlogic.com
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
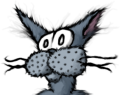
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sat Nov 15, 2003 6:28 am Post subject: |
[quote] |
|
Rainer Deyke wrote: | I think I disagree with you, although I can't be sure because you haven't defined what you mean by "global". Globals don't have to be placed in the global namespace. They can be made local to a file (by placing them in an anonymous namespace), local to a class (by declaring them as static member variables), or placed in a named namespace. Globals don't have to give universal write access. They can be made const, or they can be instances of a class that doesn't expose any mutating operations. |
Which was why I said the global namespace. But still, globals at all are bad, as there is always a safer alternative. But, those others are better alternatives than simply polluting the global namespace, simply because they provide a level of indirection about the global.
Quote: | A singleton is one way to expose global data, but it isn't the only way and it's not necessarily the best way. The fkyweight pattern is another option, as is a set of standalone functions. Sometimes the simplest way - exposing the global directly - is the best. I rarely encounter problems related to global variables, and I've never encountered one that would have been fixed somehow by hiding the global behind a singleton. |
I didn't say it was the way; just an example of a way.
Quote: | When you get down to it, singletons don't offer much advantage over global variables. The variable is still there, it's still global, it can still be accessed, and the namespace is still "polluted". Singletons offer one huge advantage in C++ - you can control when they are created and, to a lesser degree, when they are destroyed - but that's only sometimes needed, and there are other ways of obtaining the same effect. |
Very true. Singletons really just hide the pollution -- to a degree, better than simple dumping of the variables at global scope. To their credit, though, they do prevent multi-instantiation, which is their primary use. They also provide a nice way to wrap functions that must conform to a set of function pointers (like those used by GLUT) in an object.
Quote: | I think the original poster's question not about how best to expose global data, but about whether one should use global data at all. |
To which I say that global data should not be used at all, if it can be prevented. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
XMark Guitar playin' black mage

Joined: 30 May 2002 Posts: 870 Location: New Westminster, BC, Canada
|
Posted: Sat Nov 15, 2003 6:49 am Post subject: |
[quote] |
|
The method I use is to try to use as few globals as possible, but when passing variables around methods and objects starts cluttering up the code and causing more confusion than it's worth, I globalize it, or change it from private to public. _________________ Mark Hall
Abstract Productions
I PLAYS THE MUSIC THAT MAKES THE PEOPLES FALL DOWN!
|
|
Back to top |
|
 |
grenideer Wandering Minstrel

Joined: 28 May 2002 Posts: 149
|
Posted: Sat Nov 15, 2003 7:38 am Post subject: |
[quote] |
|
I'm surprised there haven't been more responses, since globals are usually a heated topic. A lot of people hate them and there are a lot of ideologies that view them as evil. It really all depends what you're doing.
Still, as someone who used to love globals, when designing a larger program or game that has different modules, it's a good idea to keep everything in its own place. Globals are usually used because they are easier to implement, but when code gets abstract I could see how globals could be a bad thing.
I'm a fan of singletons. Their purpose isn't simply to keep a single instance of variables, but more importantly a single instance of the classes which encapsulate them. If you have a display manager you probably only want one, and it's possible that you can accidentally instantiate a second one and not know it, but wonder why the data isn't being set correctly. _________________ Diver Down
|
|
Back to top |
|
 |
akOOma Wandering Minstrel

Joined: 20 Jun 2002 Posts: 113 Location: Germany
|
Posted: Sat Nov 15, 2003 1:28 pm Post subject: |
[quote] |
|
I don't use globals in C++, I do it with the class method:
Code: |
class _npc {
int x_pos;
int y_pos;
// More variables follow here
}
class _engine {
int map_x_wide;
int map_y_wide;
// More variables follow here
void map_draw();
void map_load();
// More procedures follow here
_npc npc[100];
}
int main() {
_engine engine;
// Code follows here
return 0;
}
|
So you don't have to make variables global, because all variables are in the main class (or in a sub class like _npc) and all procedures are in the main class, too...so you can read or write to variables from every procedure without using globals _________________ Keep on codin'
-----------------
-----------------
Just another post to increase my rank...
|
|
Back to top |
|
 |
valderman Mage
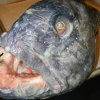
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Sat Nov 15, 2003 1:44 pm Post subject: |
[quote] |
|
I really think you should avoid globals as much as possible. Here is a link to my first (and so far, only) project where I have attempted to keep the code clean and organized. Look in "defines.h", that's where the only globals reside - and that's global constants. Globalizing more than that is generally not a good idea. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
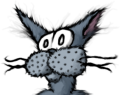
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sat Nov 15, 2003 1:46 pm Post subject: |
[quote] |
|
akOOma wrote: | So you don't have to make variables global, because all variables are in the main class (or in a sub class like _npc) and all procedures are in the main class, too...so you can read or write to variables from every procedure without using globals |
That totally disregards the topic of this thread; the point was not how to use variables in an object hierarchy, but the scope of those objects themselves in the program. At some level, you're going to find that your passing around the same pieces of data around to many different objects. The easy way to avoid the need to manage a shitload of 4-byte pointers is to just make a single, global instance that can be accessed from anywhere. _________________ "...LeoDraco is a pompus git..." -- Mandrake
Last edited by LeoDraco on Sat Nov 15, 2003 3:15 pm; edited 1 time in total
|
|
Back to top |
|
 |
BigManJones Scholar
Joined: 22 Mar 2003 Posts: 196
|
Posted: Sat Nov 15, 2003 2:36 pm Post subject: |
[quote] |
|
akOOma wrote: | I don't use globals in C++, I do it with the class method:
Code: |
class _npc {
int x_pos;
int y_pos;
// More variables follow here
}
class _engine {
int map_x_wide;
int map_y_wide;
// More variables follow here
void map_draw();
void map_load();
// More procedures follow here
_npc npc[100];
}
int main() {
_engine engine;
// Code follows here
return 0;
}
|
So you don't have to make variables global, because all variables are in the main class (or in a sub class like _npc) and all procedures are in the main class, too...so you can read or write to variables from every procedure without using globals |
Can anyone say 'stack overflow'? Err, I'm thinking in this example the _engine is allocated on the stack which is a fast allocation as opposed to the heap; but access to member variables of the stack allocated object is slower than accessing member from a heap (new()) allocated object? Somebody correct me here....
Globals SUCK and so do you if you use them!!!
|
|
Back to top |
|
 |
Adam Mage
Joined: 30 Dec 2002 Posts: 416 Location: Australia
|
Posted: Sat Nov 15, 2003 4:22 pm Post subject: |
[quote] |
|
I think akOOmas answer was valid. Mebe its just my poor english skills that didin't make that clear enough. _________________ https://numbatlogic.com
|
|
Back to top |
|
 |
akOOma Wandering Minstrel

Joined: 20 Jun 2002 Posts: 113 Location: Germany
|
Posted: Sat Nov 15, 2003 4:47 pm Post subject: |
[quote] |
|
I think so, too, Adam...
The question was if we use globals or any other way to access variables from nearly everywhere in your code.
So my answer was valid, I think. _________________ Keep on codin'
-----------------
-----------------
Just another post to increase my rank...
|
|
Back to top |
|
 |
grenideer Wandering Minstrel

Joined: 28 May 2002 Posts: 149
|
Posted: Sun Nov 16, 2003 4:08 am Post subject: |
[quote] |
|
I've never heard stack access being slower than heap access.
Either way, the variables are still global if the class is global. Sure, a singleton has the same effect since you can access it anywhere. Also, you can make the data private and only allow access through accessor functions, but if you have functions to change the data it doesn't really protect it that much anyway. _________________ Diver Down
|
|
Back to top |
|
 |
 |
Page 1 of 2 |
All times are GMT Goto page 1, 2 Next
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|
|