 |
|
View previous topic - View next topic |
Author |
Message |
MadQB I wanna be a ballerina!
Joined: 31 May 2002 Posts: 24
|
Posted: Thu May 13, 2004 3:08 am Post subject: 3D Rotation |
[quote] |
|
Hey all, been busy lately and haven't been on the board much.
Anyhow... Been working on a 3D engine and I can't seem to find any good 3D rotation tutorials out there. The one's I have found so far are good in the area of teaching me how to do it and understanding it. However it would be even better if what they taught me would work. I need a good tutorial that really explains in depth because I'm doing this for an exit presentation and I wanna know it cold before I go up there and make an ass of myself. So far I have pretty much tried 2 different ways (both matrix ways):
parameters: x_degree_of_rotation,y_degree_of_rotation,z_degree_of_rotation
[Roto about x Matrix]*[Roto about y Matrix]*[Roto about z Matrix]=[Total Rotation Matrix] with dimensions of 4 rows and 4 columns. then you multiply the vector (x,y,z,1) by [Total Rotation Matrix] to get (xrotated,yrotated,zrotated,1).
also i have tried the:
parameters:
theta=degree of rotation in xy plane
phi=degree of rotation off the z axis
p=distance to point from origin
[translation matrix]*[xy roto matrix]*[yz roto matrix]=[total rotation matrix]. then just like the other one, multiply by a point vector to get the rotated point.b
I am using a left hand rule system (ie +y goes up, +x goes right, and +z goes straight into screen) and have gotten the appropriate rotational matricies for this system. Maybe i'm doing something crazily wrong, but i would like some help if at all possible. :)
Thanks! _________________ yep...
|
|
Back to top |
|
 |
valderman Mage
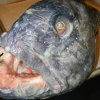
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Thu May 13, 2004 11:20 am Post subject: |
[quote] |
|
OpenGL Game Programming has some info on how to do the rotations yourself - but you probably don't have that book or you wouldn't have asked. I'll see if I can find it when I get home tonight. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
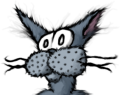
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Thu May 13, 2004 8:38 pm Post subject: |
[quote] |
|
You do know that most rotation algorithms only rotate about the origin, yes? So, unless you are attempting to rotate the object's centroid about the origin, you'll have to perform a series of transformations to a fixed point on the object, besides the basic rotation. For most types of rotations, this would imply that you need to first translate the object's fixed point (the point about which you wish to rotate) to the origin, perform the rotation, and then translate the object back. In matrix form:
T(x,y,z) * Rx(θx) * Ry(θy) * Rz(θz) * T(-x,-y,-z)
Also note that the order of your matrix multiplications matters: muliplication is not commutative for matrices. The "application" of a matrix multiplication collapses from right to left: e.g. T(-x,-y,-z) would have Rz applied to it, then that composite matrix would have Ry applied to it, etc.
Foley's Computer Graphics: Principles and Practice is a really excellent source of information for all of this. You should grab yourself a copy. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
MadQB I wanna be a ballerina!
Joined: 31 May 2002 Posts: 24
|
Posted: Tue May 18, 2004 2:40 am Post subject: |
[quote] |
|
Thanks Leo, but I did alread know that stuff, I just couldn't get what I Had to work. I'm not really trying to rotate any objects right now, just points.
But, I finally got it to work. It rotates my points just fine. I obviously have the points (8 of them) assembled into a cube because that is just what people like to see -- spinning cubes.... However, if i translate my points/cube away from their original positions, the grouping becomes distorted and they no longer resemble any sort of cubical object. I can't seem to figure out what is wrong.
Anyway, here's what I have so far:
(a=x-axis rotation angle, b=y-axis rotation angle, and d=z-axis rotation angle)
x-axis rotation matrix:
Code: | | 1 0 0 0 |
| 0 cosa -sina 0 |
| 0 sina cosa 0 |
| 0 0 0 1 |
|
y-axis rotation matrix:
Code: | | cosb 0 sinb 0 |
| 0 1 0 0 |
| -sinb 0 cosb 0 |
| 0 0 0 1 |
|
z-axis rotation matrix:
Code: | | cosd sind 0 0 |
| -sind cosd 0 0 |
| 0 0 1 0 |
| 0 0 0 1 |
|
and i multiply (xrot)*(yrot)*(zrot)=(final roto matrix) which is another 4x4 matrix. Then, my 3D point (x,y,z) is represented by the vector/matrix (x y z 1) a 1x4 matrix, and I multiply like so:
(x y z 1) * (final roto Matrix) obtaining in essence a rotated 3D vector, or rotated 3D point. Or in more general terms, some formulas to obtain it:
Code: | xrotated=x*cosb*cosd+y*(sina*sinb*cosd-cosa*sind)+z*(-cosa*sinb*cosd-sina*sind)
yrotated=x*cosb*sind+y*(sina*sinb*sinc+cosa*cosd)+z*(-cosa*sinb*sind+sina*cosd)
zrotated=x*sinb+y*(-sina*cosb)+z*cosa*cosb
|
Those are basically all I have right now. If there is some trick i don't know, or if I've done something wrong, could someone please give me a hand? If not, then I will have to "slicken" my presentation and be careful not to translate any points before i rotate them... heh. _________________ yep...
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
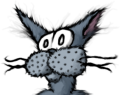
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Wed May 19, 2004 2:21 am Post subject: |
[quote] |
|
Where exactly is the original center of the points before the distortion you describe? See, assuming that your rotation algorithm is working properly, a cube constructed of points should maintain the angles and lengths between those points, as long as the rotation is done about the origin. This was a point I made in the last post: if you wish to maintain the angles/lengths of points on an object for a rotation, you have to translate the fixed point of the object about which the rotation is to take place to the origin. So, for each of the points on your cube, are you translating them such that their centriod is at the origin, prior to rotation? If you are not, then I would suggest you attempt that, then, after the rotation is complete, translate the points back.
If you are doing that, and are still gaining the distortion, I have a few questions to flesh out the situation:
Are you attempting to perform the rotation with a single rotation matrix, or by, at run time, performing a series of matrix multiplications on individual rotation matrices?
a: If the latter, are you certain that your matrix multiplication algorithm is correctly multiplying the matrices together?
b: If the former, are you certain that your composite matrix was accurately formed? I started to multiply out a composite rotation matrix by hand; while I didn't entirely finish multiplying it through the rotated point (I am lazy) -- so I'm not entirely sure if it can be reduced -- the result was vastly more complicated than the equations you provided. (Again, I didn't attempt simplification, so I posit that it isn't terribly complex; however, it is a line of questioning that you should persue.) _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
Nephilim Mage

Joined: 20 Jun 2002 Posts: 414
|
Posted: Thu May 20, 2004 7:02 am Post subject: |
[quote] |
|
My recommendation, if you're not hurting for processing speed (as I doubt you are if you're only rotating six points), would be to write routines that generate identity, single-axis rotation, single-axis translation, and single-axis scale matrices, and then multiply these matrices together at run-time to get your final transformation matrix for any given frame. You can always optimize things later by multiplying out the matrices by hand and writing composite matrix generators where applicable, but your code will be much easier to debug if they're separated out like that.
(Besides, I believe that keeping the rotation matrices separate like this helps you to dodge gimbal lock issues. Could be wrong on that, though.)
Regarding why your model would deform, it's hard to say. The first thing I would do is double-check my matrix multiplication routines, as was mentioned in an earlier post. Then double-check the matrices you are generating (much easier with the above approach). Get it working in one rotation dimension first, and layer on the other dimensions later. The above approach allows you to test every transformation you want to do. If those all work, composites should work.
Another idea is to check to make sure that you are not applying your transformation matrix to the transformed points from the previous frame, but instead to your reference model.
If you apply transformations repeatedly to transformed points (i.e., with a "delta" approach), you risk gradually eroding the precision of your model as those sines and cosines drop accuracy from float precision issues.
In other words, don't keep multiplying your points by a two degree rotation matrix. Instead, keep track of the total amount of rotation, build the matrix each frame, and apply that to your original reference model. That ensures your model is preserved, even when rotations are small enough per frame that they are beyond your float precision.
|
|
Back to top |
|
 |
MadQB I wanna be a ballerina!
Joined: 31 May 2002 Posts: 24
|
Posted: Wed May 26, 2004 9:30 pm Post subject: |
[quote] |
|
Ok, Here's what I'm doing in my code:
I have some points stored in this form:
Code: |
pts(i).x=xcoord
pts(i).y=ycoord
pts(i).z=zcoord
|
and I have 8 of them so it goes from pts(1) to pts(8) with each point stored this way in that array. I start out with them arranged in a cube centered at the origin. I can translate them by incrementing their x,y, or z coordinates and that works fine (i translate them all at once so it moves the whole cube). But when i apply my rotation formulas that I stated above it does some weird stuff (once again i apply the rotation formulas to all the points at once so it rotates the entire cube). First off, it works fine if i only rotate about one axis at a time without translating it before i rotate it. But if i rotate them about the x axis then the y-axis without resetting them, they go all over the place, they still rotate about the axis, but they lose formation. Also, if i translate them first, say i move them all to the right 5 units, and then try to rotate them about any axis, it will rotate about the axis but the points lose their formation.
Still don't know what's wrong though. _________________ yep...
|
|
Back to top |
|
 |
MDenham Lowly Slime
Joined: 26 Oct 2004 Posts: 2
|
Posted: Tue Oct 26, 2004 7:10 am Post subject: |
[quote] |
|
Well, went through and did the matrix multiply by hand (oh yeah, woo hoo, :-P thank GOD I can remember how to do it still, as it's been something like eleven years since the last time I really did it), and so here's the correct form of the final matrix:
Code: |
| cos(b)*cos(d) cos(b)*sin(d) sin(b) 0 |
| sin(a)*sin(b)*cos(d)-cos(a)*sin(d) sin(a)*sin(b)*sin(d)+cos(a)*cos(d) -sin(a)*cos(b) 0 |
| -cos(a)*sin(b)*cos(d)-sin(a)*sin(d) -cos(a)*sin(b)*sin(d)+sin(a)*cos(d) cos(a)*cos(b) 0 |
| 0 0 0 1 |
|
...so I'd put in that matrix as something to check your resultant against. Your matrix multiplication routine may be wrong (if your results consistently are incorrect, then it is :->).
|
|
Back to top |
|
 |
MyNameIsJeff I wanna be a ballerina!

Joined: 24 Sep 2004 Posts: 22 Location: Nebraska, United States
|
Posted: Thu Oct 28, 2004 3:03 am Post subject: |
[quote] |
|
Get out your shovels, men - It's time to to go thread mining. _________________ Guile says: Go home and be a family man.
|
|
Back to top |
|
 |
BadMrBox Bringer of Apocalypse
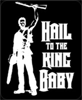
Joined: 26 Jun 2002 Posts: 1022 Location: Dark Forest's of Sweden
|
Posted: Sat Oct 30, 2004 12:47 pm Post subject: |
[quote] |
|
Surf onto rel.betterwebber.com. There is much good stuff on 3d there. _________________
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|