View previous topic - View next topic |
Author |
Message |
janus Mage
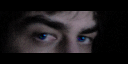
Joined: 29 Jun 2002 Posts: 464 Location: Issaquah, WA
|
Posted: Fri Aug 19, 2005 9:52 am Post subject: |
[quote] |
|
biggerUniverse wrote: | I believe that it is unnecessary to continue talking about JS (Janus, you too) in this thread. If you find that you can take the knowledge you have of JS and apply it to C++, please feel free to post that application of your knowledge (test it first). | If he said he was using C++, I must have missed it. It is pretty likely that he's not using JS, though :)
Also, you might want to note that instead of using a huge grid of 'occupation' values or a simple list of entities, you could use something like a quadtree to subdivide the world into smaller sections. That'd significantly cut down on the number of comparisons necessary in RuneLancer's theoretical setup, and probably still be more efficient than just having a grid.
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Fri Aug 19, 2005 12:15 pm Post subject: |
[quote] |
|
janus wrote: | That'd significantly cut down on the number of comparisons necessary in RuneLancer's theoretical setup, and probably still be more efficient than just having a grid. |
if(mySprite.mapHeight & world.occupation[mySprite.y * XSIZE + mySprite.x]) is a lot of comparaisons? ;)
Admittedly, comparing ranges in the case of a non-grid-based game like I described it is quite a few more comparaisons in the event of a large number of actors and could benefit from less straightforward code. But for the most part, unless you have a hundred or so sprites, this won't be a bottleneck and too much time spent optimising this in favor of, say, proper render techniques (VBOs, display lists; dirty rectangles to avoid unecessary updates in 2D...) would be wasted time.
Unless you DO have quite a lot of NPCs and they all move around. Then, seeing as this is an n^2 algorithm, yeah, it could start being a bit of a bottleneck. :P _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
biggerUniverse Mage
Joined: 18 Nov 2003 Posts: 326 Location: A small, b/g planet in the unfashionable arm of the galaxy
|
Posted: Sun Aug 21, 2005 2:38 am Post subject: |
[quote] |
|
janus wrote: | biggerUniverse wrote: | I believe that it is unnecessary to continue talking about JS (Janus, you too) in this thread. If you find that you can take the knowledge you have of JS and apply it to C++, please feel free to post that application of your knowledge (test it first). |
If he said he was using C++, I must have missed it. It is pretty likely that he's not using JS, though :)
Also, you might want to note that instead of using a huge grid of 'occupation' values or a simple list of entities, you could use something like a quadtree to subdivide the world into smaller sections. That'd significantly cut down on the number of comparisons necessary in RuneLancer's theoretical setup, and probably still be more efficient than just having a grid. |
I did assume C++, but I think it's a fair assumption. Yeah, I mentioned quadtrees earlier :). Quadtrees are the most robust solution. If you set it up correctly (allow the subdivision depth to be set at runtime), then you can scale to the mapsize and number of actors. _________________ We are on the outer reaches of someone else's universe.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Mon Aug 22, 2005 7:12 pm Post subject: |
[quote] |
|
Question: it seems to me that the easiest way to copy structured data is to read the data that starts at the address of the structure through the length of the structure, and store the read data into a variable length array. Does this work?
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Mon Aug 22, 2005 9:07 pm Post subject: |
[quote] |
|
You can do that, yes. Using memcpy, it'd go a little like...
memcpy(StructureA, &StructureB, sizeof(STRUCTURE));
Only this is very dangerous. Assuming you do know that both structures are of the right type and that the one you're copying to has the right amount of data reserved for copying, a straight copy may not always be desirable in OOP. It depends on the situation. For instance, invalidated data still gets copied, whereas a cloneObject method could verify that what's being cloned is valid data. The best example I can think of are pointers being destroyed by another "parent" object and not being set to null (a bad practice to begin with, though.)
There's no "best" way to do anything when programming. Just a "best" way to do something given a specific case. Applying different algorithms to the right situation beats doing brute-force things such as copying an entire structure. Then again, in some cases that is the best solution. :P _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
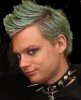
Joined: 29 May 2002 Posts: 559
|
Posted: Wed Aug 24, 2005 7:37 am Post subject: |
[quote] |
|
In C++ you should *never* I reapeat *never* use memcpy or other straight to the metal copy methods unless you're darn sure that the object being copied is of POD type. A POD (Plain Old Data) type doesn't really qualify as an object it's only a data holder, like a C-struct. Doing bitwise copying of anything that have object semantics is a sure way to give yourself a true headache.
To sum it up, in C++ if you have (a non trival)
copy ctor
dtor
op=
you should (apart from most likely having all three) never ever use memcpy or similar on it. _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
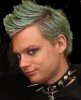
Joined: 29 May 2002 Posts: 559
|
Posted: Wed Aug 24, 2005 7:41 am Post subject: |
[quote] |
|
LordGalbalan wrote: | Question: it seems to me that the easiest way to copy structured data is to read the data that starts at the address of the structure through the length of the structure, and store the read data into a variable length array. Does this work? |
See my previous reply and you asking this show's a great deal of missunderstanding on the whole OO concept. Also "structured" data comes in more flavours than one might think and most of them simply doesn't have a (physical) "start" and "end" address.
So what do you consider "structured" in this case?
Anyhow sometimes the best way to "copy" data is to not copy it at all, COW (copy on write) somtimes called lazy copying can for rarly changed but often shared data save large amounts of memory and improve performance (due to removal of copying overhead).
The "best" way to conceptually copy something therefore large depends on context. _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Wed Aug 24, 2005 12:20 pm Post subject: |
[quote] |
|
Unless you plan on modifying that data, why not simply create a pointer to it and be done with the whole mess?
So long as you're careful not to end up in a situation where your pointers are desynchronised and you destroy the object without updating the new ones pointing to the same object (which can be very dangerous!)
To handles resources in my game, instead of stupidly reloading the same texture multiple times in memory, I increment its reference count. Whenever something ditches the texture, I decrement the reference count and ONLY delete it when it hits 0. This means I can "copy" a texture between objects in the game world (game objects, not OOP objects), but not waste space with redundant data.
And it illustrates the joys and the power of OOP. Seeing as deleting the texture is a private method that forces the whole reference count check to be done when called and that nothing can ever simply delete the texture without going through the appropriate code first, I can't end up in a dangerous situation short of doing something dumb to begin with (ie, destroying the object forcibly and trying to access its resources anyways; the deconstructor should delete the textures on its own to avoid a leak anyhow, once it goes out of scope.) _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
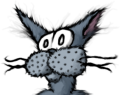
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Wed Aug 24, 2005 8:29 pm Post subject: |
[quote] |
|
RuneLancer wrote: | Unless you plan on modifying that data, why not simply create a pointer to it and be done with the whole mess? |
In C++, at least, it would probably be better to go with a const reference, as you don't have to worry about the desynchronization thing.
Quote: | So long as you're careful not to end up in a situation where your pointers are desynchronised and you destroy the object without updating the new ones pointing to the same object (which can be very dangerous!) |
Smart pointers are also good for that type of thing, as you should never end up out of sync. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
 |
Page 2 of 2 |
All times are GMT Goto page Previous 1, 2
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|