View previous topic - View next topic |
Author |
Message |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Tue Sep 27, 2005 11:59 pm Post subject: |
[quote] |
|
Aha! Perfect! That's exactly what I was hoping. :)
Here's the C solution. Sorry, this is off the top of my head, so the syntax for malloc may be off a little... Some debugging will be required. ;)
Code: | #define SIZETORESERVE 512
int main()
{
char* listOfBytes = NULL;
int i;
listOfBytes = malloc(SIZETORESERVE, sizeof(char));
if(!listOfBytes) return 1; //Safety check.
// Writing...
for(i = 0; i < SIZETORESERVE; i++)
listOfBytes[i] = i % 10;
// Then reading...
for(i = 0; i < SIZETORESERVE; i++)
printf("%d\n", listOfBytes[i]);
return 0;
} |
Pretty sure i'm wrong about malloc's syntax, so look into it a little if this doesn't compile. The C++ solution is a little simpler.
Code: | #define SIZETORESERVE 512
int main()
{
char* listOfBytes = NULL;
listOfBytes = new char[SIZETORESERVE];
if(!listOfBytes) return 1; //Safety check.
// Writing...
for(int i = 0; i < SIZETORESERVE; i++)
listOfBytes[i] = i % 10;
// Then reading...
for(i = 0; i < SIZETORESERVE; i++)
printf("%d\n", listOfBytes[i]);
delete [] listOfBytes;
return 0;
} |
Give that a shot. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
DeveloperX 202192397
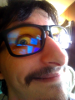
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Wed Sep 28, 2005 12:25 am Post subject: |
[quote] |
|
RuneLancer wrote: | Aha! Perfect! That's exactly what I was hoping. :)
Here's the C solution. Sorry, this is off the top of my head, so the syntax for malloc may be off a little... Some debugging will be required. ;)
Code: | #define SIZETORESERVE 512
int main()
{
char* listOfBytes = NULL;
int i;
listOfBytes = malloc(SIZETORESERVE, sizeof(char));
if(!listOfBytes) return 1; //Safety check.
// Writing...
for(i = 0; i < SIZETORESERVE; i++)
listOfBytes[i] = i % 10;
// Then reading...
for(i = 0; i < SIZETORESERVE; i++)
printf("%d\n", listOfBytes[i]);
return 0;
} |
Pretty sure i'm wrong about malloc's syntax, so look into it a little if this doesn't compile. The C++ solution is a little simpler.
Code: | #define SIZETORESERVE 512
int main()
{
char* listOfBytes = NULL;
listOfBytes = new char[SIZETORESERVE];
if(!listOfBytes) return 1; //Safety check.
// Writing...
for(int i = 0; i < SIZETORESERVE; i++)
listOfBytes[i] = i % 10;
// Then reading...
for(i = 0; i < SIZETORESERVE; i++)
printf("%d\n", listOfBytes[i]);
delete [] listOfBytes;
return 0;
} |
Give that a shot. |
I already knew that kind of shit...that isnt what I'm looking for man.
I want to get a hex dump of the contents of ram at any segment I specify.
I know all about using malloc and new for memory management.
that is not what I'm after.
I want to get listings of what is in my computer's ram ~ eventually I will be attempting to directly modify the contetns of the ram to make alterations to software ~ aka game trainers etc.. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Wed Sep 28, 2005 1:15 am Post subject: |
[quote] |
|
Ohhh, now I get it. That's a lot more complicated than you'd think, frankly. I only know how to do it via Windows, as it involves some stuff with the API. To be fair, I've only done this on Win98, and I don't know if XP has any further protections that would disallow this sort of thing. I wrote a trainer for FF3 running under SNES9x in VB quite a while ago which did some basic stuff like monitoring some values and freezing others.
First, you should know that different processes run in their own "bucket" of memory, seperate from the others. You can't just attempt to access another process's memory or Windows will throw an error your way and most likely crash your program for attempting to do something it didn't have permission to do. So you need to grab a handle to the application you want to read from in order to mess around with it.
hWnd = FindWindow(NULL, "Window Name");
This function returns a handle to the application with the name "Window Name" as a window name. There's also (name may be a little off...) GetWindowFromPoint or something like that which allows you to specify a point onscreen and returns the handle to the window there.
GetWindowThreadProcessId(hWnd, &pID);
This returns the thread process ID of the window you selected. Once you have this, you can tell Windows which thread you want to access.
hProcess = OpenProcess(PROCESS_ALL_ACCESS, 0, pID); //Note sure if that's the right constant name... I'm copying this from my VB trainer. :P
Here, you basically tell Windows you want access to a process to do stuff with it. You have to pass a flag telling it what kind of access you're looking for, a boolean telling wether the handle is inherited or not, and the process ID you got from GetWindowThreadProcessID.
WriteProcessMemory(hProcess, Address, &Value, Len_To_Write_In_Bytes, &x);
ReadProcessMemory(hProcess, Address, &ReturnValue, Len_To_Read_In_Bytes, &x);
Either of these will return how many bytes were actually written/read in "x".
Finally, make sure you release the process!
CloseHandle(hProcess);
Last but not least, ALWAYS check stuff before every step. Make sure you DID grab a handle from something before using it. Yeah, yeah, common sense, I know, but it bears mentionning. :)
Give MSDN a look if you want more info on these functions. Dunno how you can get it to work in console though, as the Win32 API isn't accessible then. Or on another OS, but as this is an OS-specific issue, using the API would probably fail even if you could. ;P _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
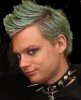
Joined: 29 May 2002 Posts: 559
|
Posted: Wed Sep 28, 2005 7:25 am Post subject: |
[quote] |
|
@DevX: If you had any sort of clue you would quite quickly realize that all the "ports" are basicly correct but that your understanding with the architechture you're working with is flawed. Basicly the code you have in QB is working under something that's called realmode a 20bit segmented memory model and if you're looking to make a straight port you'll need to dig up some ancient C compiler (I've heard that Borland Turbo C v2 or 3 is available online from their museum) that can complie to such target. Then you'll have to look at the compiler specific extensions to handle the segmented memory model, in the TC case it's the added keyword "FAR" and MAKE_FAR_PTR (or something very similary 6years since I used it).
If you're trying to compile it under anyother enviornment than plain dos without a dos-extender then basicly you'll have to rethink your approach since you're then likely in protected mode, even worse you could be under a proper OS (NT based Windows or *nix derivate/clone) that implements memory protection specifically aimed at not letting you trample the memory of other processes.
And in that case RuneLancer gave you some nice pointers, the problem is that physical memory address have very little todo with whatever memory addresses gets mapped into your process.
LordGalbalan is correct under some very very pecuilar circumstances like home-rolled memory extenders for dos or home brewn OS's without virtual memory and things like that.
Basicly your problem is that you'll have to consider your target architechture when porting code and if you're aiming to use silly unportable memory tricks from Qb then you'll simply have to stay stuck in the DOS days. _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
DeveloperX 202192397
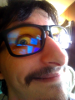
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Wed Sep 28, 2005 4:49 pm Post subject: |
[quote] |
|
DC: Oh.
I see.
Alright, well, this topic is closed then.
Sorry about all this, it makes sense now. :D
I forgot about the realmode /protectedmode thing, as I have only read about it in 1 of my books, and that book got left behind in LA. :P
Oh well, theres more fun things I can find to code. :D _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
 |
Page 2 of 2 |
All times are GMT Goto page Previous 1, 2
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|