 |
|
View previous topic - View next topic |
Author |
Message |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Tue Oct 18, 2005 2:19 am Post subject: Mathematical Art |
[quote] |
|
We've all heard of this, I'm quite sure. Mostly chaos theory and the likes; fractals, attractors, iterative systems...
So, who's into this stuff? Has anyone written their own attempts at modifying one of the more basic systems?
I used to be a big fractal buff, but eventually took interest in attractors. The basic code behind an attractor is REAL simple...
Code: | x = RandomValue
y = RandomValue
while(numIterations++ < maxIterations)
{
newX = EquationA()
newY = EquationB()
setPixel(X, Y)
x = newX
y = newY
} |
Of course, X and Y are scaled up when drawing. Typically an equation should fall between (-4,-4) and (4,4), so you'd do something like ((X - MIN_X) / SCALED_WIDTH) * TOTAL_WIDTH to get X, same with Y. Say... drawX = ((X + 4) / 8) * 640...
Often you'd create a palette and increment the color by 1 where the pixel would be drawn.
This was rendered by a program I wrote in my early C++/GDI days.
I came up with a cool twist on these while trying to create some kind of mapping of potentially good starting x/y "positions." What I did was go through every pixel on the screen, use those as positions (after scaling them down; see above), then color them by how far the new x/y were from the original one. Sometimes I'd iterate a few times. The results were stunning.
So, does anyone else do stuff like this? Give us your algorithms! :D _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
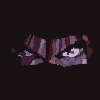
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Tue Oct 18, 2005 2:41 am Post subject: |
[quote] |
|
I've wanted to learn how to do this stuff. Any tips?
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Tue Oct 18, 2005 3:15 am Post subject: |
[quote] |
|
Just let your imagination run wild, lol.
There's two things to know about this stuff.
1- Being able to scale things properly is important. Usually, you work with values between -1 and 1. This can easily equate to a color if you know how, or a position, or whatever.
2- Understanding what an iterative process is. The first image, the attractor, is drawn by iterating over an equation which moves a "dot" around and mapping its position every time it moves. The second one iterates over the same equation, but for every possible position (and not as often), mapping a pixel colored based on how far away it has moved.
The easiest way to write something like this is to have a loop going over every pixel. Then, you just fill in the actual loop, figuring out something to do with the position you have. For instance, R could be the X, G the Y, and B (X + Y) / 2. This gives a nice gradiant, but it's nothing too impressive.
More complex stuff could involve, say, applying a formula over and over on the position and counting how many times it must be done before your pixel exits the screen, and using that as a color. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
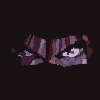
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Tue Oct 18, 2005 4:00 am Post subject: |
[quote] |
|
Have any example source?
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Tue Oct 18, 2005 5:37 am Post subject: |
[quote] |
|
I never give out source. Personal beleif that I don't wish to argue. ;) But I can give you some pretty easy to follow pseudocode.
Code: | MAXCOLORS = 255
SCALE = 20
Pal[MAXCOLORS] = GenerateRandomPalette()
X = Random(-1..1)
Y = Random(-1..1)
P1 = Random(-2..2)
P2 = Random(-2..2)
Repeat Indefinately
{
Z = X * Sin(Y) + Cos(Y) * 4 * P1
Y = Y * Cos(X) + Sin(Y) * 4 * P2
X = Z
RealX = (X + 5) * SCALE
RealY = (Y + 5) * SCALE
Col = GetPixel(RealX, RealY) + 1
If Col < MAXCOLORS
{
SetPixel(RealX, RealY, Col)
}
} |
You might want to run that a few times, as the end result depends on the parameters that get generated. You don't have to actually generate a palette; just making the display black and plotting a greyscale color works the same, if you want to save some time and see what the end result would be like.
The formula used to calculate X and Y can and should be tweaked to give different sets. This is an Attractor, btw; there are many different things you can do if you vary the algorithm (for instance, that map I posted as my second picture in the first post.) _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Nephilim Mage

Joined: 20 Jun 2002 Posts: 414
|
Posted: Tue Oct 18, 2005 1:57 pm Post subject: |
[quote] |
|
Well, the big one is the Mandelbrot set. There's plenty of places out on the 'net that describe how to draw it, and the set is pretty famous nowadays. But I tend to like the related, but lesser-known, Julia sets (which are derived off of the Mandelbrot set, or rather, the Mandelbrot set is a mapping of all Julia sets) because they have a wide variety of small, interesting shapes. Here's a site with a lot of info on the Mandelbrot set, Julia sets, and how to compute them, but a little Googling will find you plenty more.
Of course, one big benefit of fractals for RPG purposes (and game programming in general) is for the computational generation of natural-looking landscapes. When you zoom in to the Mandelbrot set, or look at some of the Julia sets, you get shapes that look like inlets and seashores. If you were to height-map a Julia set instead of using colors for the different thresholds, you'd have an entire (nearly) resolution-independent island topography that could be stored with only a few bytes.
One objection people might have to using fractal landscapes is that they're too "regular" - for instance, Julia sets are often symmetrical about an axis - or too "defined" - for instance, you might not want to be bound by the features of the landscape a fractal generates. One thing I've thought about doing to resolve this would be to have a fractal landscape explorer that would allow you to select multiple Julia sets and overlay them on top of each other additively, with scaling, rotation, offset, and heightmap scaling. That would still give you the interesting shorelines and terrain, but you could also map out generally how you want your shorelines and mountain ranges to be laid out to serve your story and world design. Not sure how well it would work though, and I haven't had much of an opportunity to play with the idea. But it might be fun to tinker with it. _________________ Visit the Sacraments web site to play the game and read articles about its development.
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Tue Oct 18, 2005 5:44 pm Post subject: |
[quote] |
|
I find that fractals are a pretty bad idea for landscapes, personally. They're great to explore, but... just not up to snuff when it comes to landscape generation. There are far better methods. (Although they DO provide very intriguing masses of land :P )
- The easiest one is just to draw noise, smoothen it out, normalise it, and make a heightmap out of it. Perlin noise is pretty much the undisputed king of natural effects, such as landscapes and clouds, but other forms of noise can generate very nice landscapes.
- An easier but less interesting method is to generate a random number of shapes. For instance, overlaying 'x' circles with a gradient (dark on the outside, light in the center) can create a nice set of rolling hills. Not very good for a world map though.
- Faulting is a great way to generate landscapes both as a 2D grid and 3D sphere (but tends to generate symmetrical landscapes for the latter...) Basically, you generate a random vector. The land on one side gets raised by 1 unit, the land on the other gets lowered by 1. Repeat.
- There's also the classical midpoint displacement (ie, "Fractal cloud") which involves recursively averaging the four corners of a box, varying it by a small random value, splitting the box into 4 more boxes, and starting over. Looks pretty good but gives smooth, uninteresting landscapes...
A bunch of effects can be applied afterwards. Erosion comes to mind, but that's pretty computational intensive. Adding water's pretty important too. You can just create a plane at an arbitrary height and have everything under it be water, but there are better methods. I have a test program which generates land and "drops" pixels of water down slopes. It takes ages to get reasonable lakes, but they look quite realistic (and it's real easy to add erosion to it.)
Then comes the rendering phase... Voxels are a cool way to render land. Personally, I usually raytrace it; the results are pretty cool but it takes a while. :P
Particle systems; anyone working on those? :D _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Nephilim Mage

Joined: 20 Jun 2002 Posts: 414
|
Posted: Tue Oct 18, 2005 6:29 pm Post subject: |
[quote] |
|
Well, the nice thing about fractally-generated landscapes, as I mentioned, is the amount of compression you can get on them. The same would apply for those other techniques, if you store the random seed.
The way I see it, the fractal method and other methods you mentioned is a way to quickly define and store a large wilderness area or land mass. For most purposes, though, you'd still want to heavily manipulate the "interesting" places, such as towns, castles, ports, etc.
Anyway, your post prompted me to play a bit with fractals, so I just wrote a quick Mandelbrot/Julia renderer for fun. Here's some sample renders (Mandelbrot on the left, a Julia on the right):

...and here's the two above images composed and dropped into a quick and dirty mesh generator I wrote a while back:
 _________________ Visit the Sacraments web site to play the game and read articles about its development.
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Tue Oct 18, 2005 11:34 pm Post subject: |
[quote] |
|
That mesh is BEAUTIFUL.
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Wed Oct 19, 2005 5:28 pm Post subject: |
[quote] |
|
Very nice mesh; I love the way the edges form fjords. I've always found fractals to be pretty unnatural in shape to use for landscapes, but combining a number of them together and averaging them out could/should give a nice result... :o
Figured I'd post some pseudocode to illustrate how I did the rain fill type of effect I mentionned in my previous post. Really basic stuff...
Code: | (Repeat until results satisfactory)
X = Random(0..MAX_X)
Y = Random(0..MAX_Y)
Z = MAX_HEIGHT
Repeat
DropSettled = TRUE
ForEach Surrounding Grid Unit
If Height_of_Grid < Z
X = Grid_X
Y = Grid_Y
Z = Height_of_Grid
DropSettled = FALSE
Until DropSettled
Height_of_Grid(X,Y)++ |
In short, a drop falls from a random position and "follows" the terrain until it reaches a point where there is no more slope in the immediate vicinity. It then, for all intent and purpose, raises the land where it settles (so that water builds up). Keeping the actual height and the water's height in two different arrays would be wise otherwise you'd have no way of telling land and water apart. The cool thing about this method (as opposed to just raising a plane of water) is that, suppose you have a crater atop a mountain, water will pool into it. With a raised plane, water won't reach it and it'll remain dry. The same technique could probably be used with snow if the tolerance for slopes is increased slightly.
This can be VASTLY improved a good number of ways. It's a basic algorithm, after all, and not something that should be used in an actual project. Favoring the steepest slope would be more realistic than the first sloping part in the terrain it encounters, for one. Adding erosion is another possibility. And moving one drop at a time would be horrible slow (and could lead to thin, deep ravins while eroding...) But the basic idea's there.
I'll write up a quick VB demo or something and post it here when I get home tonight.
Edit: Here's a pic from an old app I found. Unrelated, but I thought it was pretty cool considering it's about 4-5 years old. :P
 _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|