 |
|
View previous topic - View next topic |
Author |
Message |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Wed Feb 08, 2006 2:32 am Post subject: Timing |
[quote] |
|
Ok, I've actually started coding! I started up the project and everything, and I'm all amped to go.
However, I do have one problem. I need to know how to manage the time within the game.
Is there a way to handle stuff without relying on previous frame times?
Jason
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
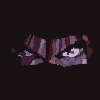
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Wed Feb 08, 2006 2:59 am Post subject: |
[quote] |
|
If you're using SDL, you can use SDL_GetTickCount() which gives you milliseconds that you can compare to later times to get time passed. If you're using anything else, just check the API for anything about ticks.
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Wed Feb 08, 2006 3:53 am Post subject: |
[quote] |
|
No problem
Last edited by Gardon on Wed Feb 08, 2006 4:20 am; edited 1 time in total
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Wed Feb 08, 2006 3:54 am Post subject: |
[quote] |
|
Maybe I have to use the system's counter, like the atomic clock of the computer itself to check time.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
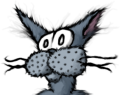
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Wed Feb 08, 2006 4:07 am Post subject: |
[quote] |
|
Hey Gardon: I realize you want answers to your questions, and see them as imperative, but could you please edit your previous posts rather than multi-post? Those whom have not seen your last message will still have something new (regardless), and it tends to keep your threads cleaner.
As it is, with the wonderfully large amount of posts per minute around this place, you hardly have to worry about keeping a topic bumped.
General exception to the above etiquette rule: if your topic has been unresponsive for a period of time greater than, say, a day or so, the multi-post thing might be excusable. However, posting again within a minute of your last post is a bit, ah, flamboyant. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Wed Feb 08, 2006 4:35 am Post subject: |
[quote] |
|
Gardon wrote: | Maybe I have to use the system's counter, like the atomic clock of the computer itself to check time. |
Woah. You have an atomic clock in your PC? ;)
The simplest way to handle timing is this.
while(!GameHasEnded)
{
x = GetTickCount();
DoGameStuff();
while(GetTickCount - x < DesiredDelayPerFrame) Sleep(0);
}
This is just fine for a small, basic game. However, if you want something more reliable, look into time-based movement. This means not restricting the game's speed at all, but rather, adapting your game to faster computers. Why penalize someone with a better PC?
Time based movement is basically moving your sprites by a unit (NOT necessarily a pixel) obtained by the average amount of time spent rendering a frame. For instance, every step forward moves you (100 / AvgMSPerFrame) units forward.
If the game runs at 60 FPS, you move 1.666 units per frame for a total of (60x1.666=...) 100 units per second.
If the game runs at 200 FPS, you move 0.5 units per frame for a total of (200x0.5=...) 100 units per second.
If the game runs at 5 FPS on a REALLY slow PC, you move 20 units per frame (again 100 per second.)
No matter what speed the game runs at, you are 100% guaranteed that the player will run it at the same speed as anyone else. Maybe with a far less enticing framerate, if their PC is sub-par. And if their PC is a space-age monstrosity 100x better than yours? They'll be able to reap the benefits of having uber-smooth 5000 FPS gameplay without having their character fly across the screen 50x faster than you'd expect!
This works best with 3D, where distances are measured in a virtual unit instead of physical pixels, so it may not apply to your game. Try the first method first; in a 2D game, having smooth movement isn't as important as a 3D game. You can get away with 30 FPS in a 2D game and anything more wouldn't make much of a difference; in a 3D game, 30 FPS isn't much by today's standards. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Wed Feb 08, 2006 4:51 am Post subject: |
[quote] |
|
That directly answered my question. Incredible
No seriously, I never would have thought of something like that (well, maybe sooner or later when I finally sat down to really think about what needed to get done).
And that's what I meant, having the guy move the same amount of space, regardless of his framerate.
And it works too because I can have a set speed to move him, unlike previous projects where I move him according to other things.
I went with the 2nd one because it seems more smooth. I've never liked the sleep method, and this is a good alternative.
Thank you so much,
Jason
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Wed Feb 08, 2006 5:13 am Post subject: |
[quote] |
|
I do have another question though.
What would be a good unit for 2D games?
What I was thinking of doing was having the timer function set a variable to however long the last frame was (giving the milliseconds of the frame).
I then divide that millisecond value from 1000 to get the fps.
AFterwards, I take the given speed I want to move at, and divide it by the fps to get the units to move.
So what should the units be? I know this sounds pretty basic, but if you think about it, it gets harder.
Can you approximate pixels and such, so that I can move it based on pixel movement? It would always be constant movement, but the decimal places might throw some things off.
Here's an example:
Time from last frame: 16.667 milliseconds
1000 / 16.667 = 60fps
Desired speed = 100 pixels/second
100 / 60 = 1.667units
Now, if you look at another one:
Time from last frame: 1 millisecond
1000 / 1 = 1000fps
Desired speed = 100 pixels/second
100 / 1000 = .1 units
So for both examples we have 16.667 and .1
Would those round, so 16 would always be 17 pixels and .1 would always be 0?
Jason
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
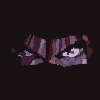
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Wed Feb 08, 2006 5:39 am Post subject: |
[quote] |
|
They don't round unless you're using auto-truncating data types such as an int. I'd use floats for those calculations. Also for choosing your unit size, just think of the idea fps (60) and how fast you want a character to move in that condition (pixels/sec) then convert to your unit size for global use.
|
|
Back to top |
|
 |
RuneLancer Mage

Joined: 17 Jun 2005 Posts: 441
|
Posted: Wed Feb 08, 2006 5:46 am Post subject: |
[quote] |
|
Just backing up LD's earlier post.
Have you experimented before posting? For all you know, sub-pixel precision in your game may average out to something fully acceptable when you display your sprite.
Experiment man, some of the answers you need to find for yourself. ;)
By the way, what you're calculating with...
Quote: | What I was thinking of doing was having the timer function set a variable to however long the last frame was (giving the milliseconds of the frame).
I then divide that millisecond value from 1000 to get the fps. |
...isn't the FPS. It's the amount of time spent on a given frame. Keep a count of how many frames have been rendered and check if a second has elapsed at the end of an iteration through your game loop. If so, your FPS is the amount of frames. Basic way to do it; your FPS will be precise within roughly a second.
You can also take the amount of miliseconds elapsed since the last frame and divide 1000 by it. 35ms to render your frame = 28.5 FPS. Note that this isn't very accurate because GetTickCount is only accurate within roughly 10-15ms.
Read this for information on the various timing functions available to you if you need better precision in future projects. _________________ Endless Saga
An OpenGL RPG in the making. Now with new hosting!
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|