 |
|
View previous topic - View next topic |
Author |
Message |
DeveloperX 202192397
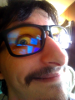
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Fri Oct 06, 2006 5:52 pm Post subject: c++ function pointer question |
[quote] |
|
Is it possible to create function pointers that could have variable arguments?
like I've got 3 functions as such:
void funct1(void*ptr1);
void funct2(void* ptr1, void* ptr2);
void funct3(int v1, int v2, int v3);
and I wanted to use a function pointer to call them dynamically.
I use void (*caller)(void*); for the first one...but I don't think the others would work...would they? (kinda afraid to try it..unknown results if you know what I mean) ...
is it possible?
and if so, can you show me some code that can do it? thanks. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
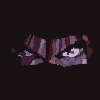
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Fri Oct 06, 2006 7:09 pm Post subject: |
[quote] |
|
function pointers must have the same signature and return type. If you want to send different kinds of data, then send a struct you make yourself that specifies what kind of data it is or whatever.
I had to do this when working with MFC worker threads since you can only send one LPVOID. I'd end up sending the this pointer or a pointer to a new structure I created.
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Sat Oct 07, 2006 1:07 am Post subject: |
[quote] |
|
No, you need individual pointers for each one. i.e.,
Code: |
void (*funct1_ptr)(void *);
void (*funct2_ptr)(void *,void *);
void (*funct3_ptr)(int,int,int);
|
But, yes, with some bad C pointer tricks it is possible to do this:
Code: |
void (*multi_func_ptr)(void *,...) = (void (*)(void *,...))any_func;
|
This would allow you to call any function using multi_func_ptr. Beware of warnings though. Crafty use of #define will take care of the warnings. There is no type checking so if you try this with the wrong amount of parameters or pass improper types as the arguments, who knows what will happen. Obviously, it is not recommended to try this unless you really know what you're doing.
Since, you're using C++, a better solution is to define a class which overloads that one function. i.e.,
Code: |
class FuncPtr {
public:
void (*funct1_ptr)(void *);
void (*funct2_ptr)(void *,void *);
void (*funct3_ptr)(int,int,int);
void multi_func_ptr(void *a) { funct1_ptr(a); }
void multi_func_ptr(void *a, void *b) { funct2_ptr(a,b); }
void multi_func_ptr(int a,int b, int c) { funct3_ptr(a,b,c); };
};
|
and fill in the appropriate pointer variables and then just call multi_func_ptr.
Either that or use the struct approach as decribed above.
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
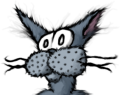
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sat Oct 07, 2006 6:42 am Post subject: |
[quote] |
|
Since you are using C++, why not just go the funtor route? If nothing else, it should be slightly more uniform and safe.
That said, your design is probably flawed if you want to mix method signatures like you suggest; it might be a better idea to evaluate what it is you are attempting to achieve, and redesign your interfaces to better reflect that. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |
DeveloperX 202192397
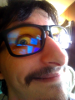
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Oct 07, 2006 6:54 am Post subject: |
[quote] |
|
LeoDraco wrote: | ...your design is probably flawed if you want to mix method signatures like you suggest; it might be a better idea to evaluate what it is you are attempting to achieve, and redesign your interfaces to better reflect that. |
Indeed, now I see that my original plan had some ugly flaws.
of course that IS cause I've done no real planning on this little project,
just started coding the idea as it came to me..I know, I know..BAD...shame on me..yeah yeah. I've cleaned it up alot, and recoded the ugly crap. Its working nicely now.
Perhaps I'll write some docs for it my next day off. heh. damn work.
Oh, and for the curious, the project is simply a custom gui library for allegro. (I hated the builtin one, and the 3rd party ones I've seen weren't what I was after..so this was born.)
done:
- buttons
todo:
-windows
-scrollbars
-static-textfields
-dynamic-textfields
now, the buttons aren't just any ol button...:)
they are easily extended by the end user to provide any style they wish.
quite a feat for me I must say, cause this is my first time using namespaces & multiple inheritance with over 10 classes! :D
well, thanks for your input. goodnight.
and if you want to check out the lib, let me know, and I'll send you a beta once I get the other 4 'controls' implemented. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Fri Oct 13, 2006 3:46 pm Post subject: |
[quote] |
|
DeveloperX wrote: | Oh, and for the curious, the project is simply a custom gui library for allegro. (I hated the builtin one, and the 3rd party ones I've seen weren't what I was after..so this was born.) |
Did you take a look at Guichan? We're using it for the GUI in The Mana World and I think it serves well the niche for lightweight game GUIs. It works with Allegro, but can also deal easily with other libraries for when you might like something else in the future. By default this includes support for SDL, OpenGL and GLUT.
|
|
Back to top |
|
 |
BadMrBox Bringer of Apocalypse
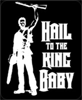
Joined: 26 Jun 2002 Posts: 1022 Location: Dark Forest's of Sweden
|
Posted: Fri Oct 13, 2006 10:24 pm Post subject: |
[quote] |
|
Are the gui for TMW going to be redesigned by the way? _________________
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Sat Oct 21, 2006 1:00 pm Post subject: |
[quote] |
|
BadMrBox wrote: | Are the gui for TMW going to be redesigned by the way? |
I guess in the future the GUI will get a redesign, but this is not a priority at the moment. Of course anybody is free to make it his priority at any time.
|
|
Back to top |
|
 |
DeveloperX 202192397
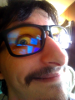
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Wed Nov 01, 2006 7:39 am Post subject: |
[quote] |
|
Bjørn wrote: |
Did you take a look at Guichan? We're using it for the GUI in The Mana World and I think it serves well the niche for lightweight game GUIs. It works with Allegro, but can also deal easily with other libraries for when you might like something else in the future. By default this includes support for SDL, OpenGL and GLUT. |
I've toyed with Guichan.
I was actually mainly writig this lib for experience using more advanced techniques that I've recently learned.
In any event, its taken a backseat, as I'm currently taking courses at www.gameinstitute.com sun-tuesday each week.
gotta go to work tomorrow morning..goodnight. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|