 |
|
View previous topic - View next topic |
Author |
Message |
BadMrBox Bringer of Apocalypse
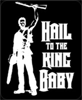
Joined: 26 Jun 2002 Posts: 1022 Location: Dark Forest's of Sweden
|
Posted: Fri Sep 14, 2007 8:15 pm Post subject: Timebased movement |
[quote] |
|
I have some problems with timebased movement. Can someone help me or point my way to an tutorial? _________________
|
|
Back to top |
|
 |
MDS-MU Monkey-Butler

Joined: 24 Oct 2006 Posts: 52 Location: sto dgo, DR.
|
Posted: Fri Sep 14, 2007 8:27 pm Post subject: Re: Timebased movement |
[quote] |
|
BadMrBox wrote: | I have some problems with timebased movement. Can someone help me or point my way to an tutorial? |
do you mean making a delay?
i think you just got to set a max ms for each visual step while moving/scrolling a tile. be sure to also let the os/windows breathe while it...i use timegettime fuction for windows :P
|
|
Back to top |
|
 |
MDS-MU Monkey-Butler

Joined: 24 Oct 2006 Posts: 52 Location: sto dgo, DR.
|
Posted: Fri Sep 14, 2007 8:32 pm Post subject: Re: Timebased movement |
[quote] |
|
mu_ds wrote: | BadMrBox wrote: | I have some problems with timebased movement. Can someone help me or point my way to an tutorial? |
do you mean making a delay?
i think you just got to set a max ms for each visual step while moving/scrolling a tile. be sure to also let the os/windows breathe while it...i use timegettime fuction for windows :P |
TimeGetTime to use timing in ms
remember to set:
timeBeginPeriod 1
timeEndPeriod 1
before and to let the pc breathe use DoEvents
ummm here is a simple function i made
Function pausetemp(ms As Long) 'delay for some time/ms
Dim first As Long
timeBeginPeriod 1
timeEndPeriod 1
first = timeGetTime
Do
DoEvents
Loop Until timeGetTime - first >= ms
End Function
um this one should b a sub actually :P but u get the idea ;)
oh ya the declaration
Option Explicit
'count to 1ms
Public Declare Function timeGetTime Lib "winmm.dll" () As Long
Public Declare Function timeBeginPeriod Lib "winmm.dll" (ByVal uPeriod As Long) As Long
Public Declare Function timeEndPeriod Lib "winmm.dll" (ByVal uPeriod As Long) As Long
|
|
Back to top |
|
 |
Verious Mage

Joined: 06 Jan 2004 Posts: 409 Location: Online
|
Posted: Sat Sep 15, 2007 3:44 pm Post subject: |
[quote] |
|
Time based movement is not a delay. In fact it is the opposite, with time based movement, the game runs as fast as possible and the frame rate is not limited.
Time based movement allows the game to play at the same speed regardless of the hardware speed (within reason); the faster the computer the smoother the game will run.
The basic concept behind time based movement is that you have a starting time (T1) and position (P1) and an ending time (T2) and position (P2). All movements between T1P1 and T2P2 are interpolated.
For example, lets assume the following (I'm exaggerating the time scale for illustration purposes, normally this might occur of the period of few seconds or less):
Origin = T1: 10:01 AM; P1: X=0, Y=0
Destination = T2: 10:05 AM; P2: X=10, Y=10
CT=Current Time
CP=Current Position
_____
On a slow computer, as time elapses, the character might progress through the following points:
[Step 1] CT: 10:01.00 AM; CP: X=0.0,Y=0.0 (0.0% of the way along the path)
[Step 2] CT: 10:02.00 AM; CP: X=2.5,Y=2.5 (25.0% of the way along the path)
[Step 3] CT: 10:03.00 AM; CP: X=5.0,Y=5.0 (50.0% of the way along the path)
[Step 4] CT: 10:04.00 AM; CP: X=7.5,Y=7.5 (75.0% of the way along the path)
[Step 5] CT: 10:05.00 AM; CP: X=10,Y=10 (100.0% of the way along the path)
Note: In the above example steps 2 through 4 are all calculated by the computer.
_____
On a computer that is twice as fast, as time elapses, the character might progress through the following points:
[Step 1] CT: 10:01 AM; CP: X=0.0,Y=0.0 (0.0% of the way along the path)
[Step 2] CT: 10:01.44 AM; CP: X=1.11,Y=1.11 (11.1% of the way along the path)
[Step 3] CT: 10:01.88 AM; CP: X=2.22,Y=2.22 (22.2% of the way along the path)
[Step 4] CT: 10:02.33 AM; CP: X=3.33,Y=3.33 (33.3% of the way along the path)
[Step 5] CT: 10:02.77 AM; CP: X=4.44,Y=4.44 (44.4% of the way along the path)
[Step 6] CT: 10:03.22 AM; CP: X=5.55,Y=5.55 (55.5% of the way along the path)
[Step 7] CT: 10:03.66 AM; CP: X=6.66,Y=6.66 (66.6% of the way along the path)
[Step 8] CT: 10:04.11 AM; CP: X=7.77,Y=7.77 (77.7% of the way along the path)
[Step 9] CT: 10:04.55 AM; CP: X=8.88,Y=8.88 (88.8% of the way along the path)
[Step 10] CT: 10:05.00 AM; CP: X=10,Y=10 (100% of the way along the path)
Note: In the above example steps 2 through 9 are all calculated by the computer. When the time has elapsed the character should always snap to the final position. As illustrated between steps 9 and 10 in the second example.
_____
The number of steps will be different on every computer (and even on the same computer) and will depend largely upon the speed of your game loop. With time based movement, the percent of travel along the path should be recalculated every game loop. This is very important because other tasks may run on the computer (or the amount of data the game is processing may change, for example if more enemies are on the screen), which could change the time interval between each iteration of the game loop.
Time based movement is similar to tweening.
|
|
Back to top |
|
 |
MDS-MU Monkey-Butler

Joined: 24 Oct 2006 Posts: 52 Location: sto dgo, DR.
|
Posted: Sat Sep 15, 2007 4:26 pm Post subject: |
[quote] |
|
Verious wrote: | Time based movement is not a delay. In fact it is the opposite, with time based movement, the game runs as fast as possible and the frame rate is not limited.
Time based movement allows the game to play at the same speed regardless of the hardware speed (within reason); the faster the computer the smoother the game will run.
The basic concept behind time based movement is that you have a starting time (T1) and position (P1) and an ending time (T2) and position (P2). All movements between T1P1 and T2P2 are interpolated.
For example, lets assume the following (I'm exaggerating the time scale for illustration purposes, normally this might occur of the period of few seconds or less):
Origin = T1: 10:01 AM; P1: X=0, Y=0
Destination = T2: 10:05 AM; P2: X=10, Y=10
CT=Current Time
CP=Current Position
_____
On a slow computer, as time elapses, the character might progress through the following points:
[Step 1] CT: 10:01.00 AM; CP: X=0.0,Y=0.0 (0.0% of the way along the path)
[Step 2] CT: 10:02.00 AM; CP: X=2.5,Y=2.5 (25.0% of the way along the path)
[Step 3] CT: 10:03.00 AM; CP: X=5.0,Y=5.0 (50.0% of the way along the path)
[Step 4] CT: 10:04.00 AM; CP: X=7.5,Y=7.5 (75.0% of the way along the path)
[Step 5] CT: 10:05.00 AM; CP: X=10,Y=10 (100.0% of the way along the path)
Note: In the above example steps 2 through 4 are all calculated by the computer.
_____
On a computer that is twice as fast, as time elapses, the character might progress through the following points:
[Step 1] CT: 10:01 AM; CP: X=0.0,Y=0.0 (0.0% of the way along the path)
[Step 2] CT: 10:01.44 AM; CP: X=1.11,Y=1.11 (11.1% of the way along the path)
[Step 3] CT: 10:01.88 AM; CP: X=2.22,Y=2.22 (22.2% of the way along the path)
[Step 4] CT: 10:02.33 AM; CP: X=3.33,Y=3.33 (33.3% of the way along the path)
[Step 5] CT: 10:02.77 AM; CP: X=4.44,Y=4.44 (44.4% of the way along the path)
[Step 6] CT: 10:03.22 AM; CP: X=5.55,Y=5.55 (55.5% of the way along the path)
[Step 7] CT: 10:03.66 AM; CP: X=6.66,Y=6.66 (66.6% of the way along the path)
[Step 8] CT: 10:04.11 AM; CP: X=7.77,Y=7.77 (77.7% of the way along the path)
[Step 9] CT: 10:04.55 AM; CP: X=8.88,Y=8.88 (88.8% of the way along the path)
[Step 10] CT: 10:05.00 AM; CP: X=10,Y=10 (100% of the way along the path)
Note: In the above example steps 2 through 9 are all calculated by the computer. When the time has elapsed the character should always snap to the final position. As illustrated between steps 9 and 10 in the second example.
_____
The number of steps will be different on every computer (and even on the same computer) and will depend largely upon the speed of your game loop. With time based movement, the percent of travel along the path should be recalculated every game loop. This is very important because other tasks may run on the computer (or the amount of data the game is processing may change, for example if more enemies are on the screen), which could change the time interval between each iteration of the game loop.
Time based movement is similar to tweening. |
well yeah movement based ON TIME...
im not delaying the hardware im just using TIME to move around like you said and it works. so yeah delay is a wrong term for this..that would be like using the sleep function :P
|
|
Back to top |
|
 |
Verious Mage

Joined: 06 Jan 2004 Posts: 409 Location: Online
|
Posted: Sat Sep 15, 2007 5:07 pm Post subject: Re: Timebased movement |
[quote] |
|
mu_ds wrote: | Function pausetemp(ms As Long) 'delay for some time/ms
Dim first As Long
timeBeginPeriod 1
timeEndPeriod 1
first = timeGetTime
Do
DoEvents
Loop Until timeGetTime - first >= ms
End Function |
The function you have outlined will cause the game loop to execute an identical number of times regardless of the hardware speed. This is because the function effectively suspends the game loop (including game logic) for a specific period of time in the "pausetemp()" function. This will result in fast hardware sitting underutilized and "idle", trapped in the following lines of code, for longer periods of time than slow hardware:
Code: | Do
DoEvents
Loop Until timeGetTime - first >= ms |
On a side note: The DoEvents statement you have outlined will result in a high level of context switches as your game yields execution to other external applications (and events within your application). However, it will also allow the user interface to remain responsive so this may be a reasonable compromise using the suspend execution approach. It is also important to note that "Sleep" functions (which I realize you did not use, but I mention for the benefit of others), when used in VB, will completely lock a game's interface, since VB is generally single threaded (excluding most ActiveX controls, which often execute in a separate thread space), but I digress.
With time based movement the computer will never sit "idle" and the number of steps/increments will increase with the speed of the hardware. This will result in smoother gameplay, better processor utilization, and a more responsive user interface.
There is a subtle, but important distinction between the two approaches. With the "pausetemp()" approach, on fast hardware, characters would move excessively fast to their destination and then wait until the time has run out, whereas, with time based movement, the characters will move smoothly to their destination during the entire time interval.
|
|
Back to top |
|
 |
MDS-MU Monkey-Butler

Joined: 24 Oct 2006 Posts: 52 Location: sto dgo, DR.
|
Posted: Sat Sep 15, 2007 8:09 pm Post subject: Re: Timebased movement |
[quote] |
|
[quote="Verious"] mu_ds wrote: | Dim first As Long
timeBeginPeriod 1
timeEndPeriod 1
first = timeGetTime
Do
'DO YOUR STUFF HERE <he>= ms
End Function |
that is just an example for him to get started with timeGetTime
and actually this WILL not run the same amount of loops each time wrong there! it's not like im telling him to use that function before each movement...but to make one of his own ;)
|
|
Back to top |
|
 |
MDS-MU Monkey-Butler

Joined: 24 Oct 2006 Posts: 52 Location: sto dgo, DR.
|
Posted: Sat Sep 15, 2007 8:20 pm Post subject: Re: Timebased movement |
[quote] |
|
[quote="Verious"] mu_ds wrote: | Function pausetemp(ms As
There is a subtle, but important distinction between the two approaches. With the "pausetemp()" approach, on fast hardware, characters would move excessively fast to their destination and then wait until the time has run out, whereas, with time based movement, the characters will move smoothly to their destination during the entire time interval. |
man that function i use it to halt the intro of the game before it has even started.... but i still underestand what you mean and i have applied it to my game but still.... it was quite complex to get it done :S
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|