 |
|
View previous topic - View next topic |
Author |
Message |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Mon Oct 01, 2007 2:20 am Post subject: Scripting help |
[quote] |
|
I'm going in circles trying to figure out how and what to script. Basically I want the ability to add/remove game items already in existence, be able to make new items specified to my liking and put them into the game without compiling, and add/create/delete items from an admin standpoint (spawning items, etc.)
Here's an example:
3 types of Armor in the game: Bronze, Iron, and Steel
Characters are able to wear 1 type of armor (body armor)
Later in the game I decide I want to add another type of armor (leg armor), and create my own statistics for that leg armor (attributes, colors, etc.) I don't want to recompile the entire game, since I"ve already released it.
Also, say I want to add another type of all major armors: Hardened steel. This would add another type of all armors that characters are able to use in the game.
I need to plan everything out first, because I want to use databases to hold all players, items, monsters, etc. Each item needs to have it's own ability to perform logic, based on character input.
Now, I program in c++. I've heard of things like .dll files to accomplish this, and script objects/files that can be used. I'm uncertain which way to go, and how to construct my programs to handle everything in the game.
I'm looking for books/articles/help from anyone who can help me. Thanks.
-Gardon
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
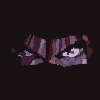
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Mon Oct 01, 2007 3:08 am Post subject: |
[quote] |
|
My current architecture I'm working on will actually offload all those systems to the scripting engine. Any info the players need will be stored in data structures in LUA. Basically my engine manages particles, the viewport and the map. The scripts tap into everything that can be changed there.
Untested idea, but I can tell you once I've had the opportunity to try it out. Right now I just have the map and particle quadtree created. Need a script manager and API calls LUA can make to specify particle behavior.
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Mon Oct 01, 2007 4:41 am Post subject: |
[quote] |
|
I guess I'm struggling with how low-level the engine should be, and how much the scripts should control.
What do you think about a command prompt? Say I hard-coded everything in my game, but was able to have a command prompt on the server that allowed me to add things while in-game?
For instance:
Game Engine allows another thread to process changes to the data within the engine.
If I had a class called: ItemDatabase
and within that class I had a function called: AddNewItem()
I could call from the console: Code: | ItemDatabase->AddNewItem(ArmorItem newArmor) |
with the ArmorItem class defined like so:
Code: | class ArmorItem
{
int durability;
int defenseBoost;
D3DXMESH object;
... etc.
};
|
The game would then be able to access this armor and its attributes. I would then have to update all players on this new item, which I could do the next time they log in (download the model, the item statistics, etc.)
|
|
Back to top |
|
 |
Ninkazu Demon Hunter
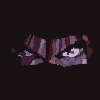
Joined: 08 Aug 2002 Posts: 945 Location: Location:
|
Posted: Mon Oct 01, 2007 4:55 am Post subject: |
[quote] |
|
The server defines item characteristics eh? Ya I'm not too sure how you'd want to have script interactivity there. Sounds like if you want to be able to redefine different item classes you'd have to restructure your server's tables and then tell the game to query for the different info via an updated script.
If structure doesn't need to be changed, you could have LUA use a custom API call you make to query for item info and then you wouldn't have to hardcode it all. The less you have to change the executable the better IMO. Script updates and server-side updates surely are the better choice.
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Mon Oct 01, 2007 6:54 am Post subject: |
[quote] |
|
How about script execution time/speed? Is it really that much slower than c++? I mean, hell, if all the client needs to do is render what the server is giving him, then the client is all set. C++ and DirectX will be used for graphics on the clients' side.
The server on the other hand, would need to implement the combat system, AI for NPCs, handle all players and their interactions, etc.
This is the meat of the game. Will LUA be able to handle it? I could see where scripting items' characteristics and Player Stats would be fine, because it's just like media (load it and you're done), but to have it for conditional logic and processing of all game-related functions might be over-doing it. What do you think?
AI is simple. Either enemies attack you, run away, or wander around. So a few 1000 AI calls might be manageable.
But after thinking about it, why code in c++ at all on the server? If everything will be scripts, why not just write the whole damn thing in LUA?
|
|
Back to top |
|
 |
Hajo Demon Hunter
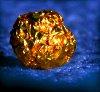
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Mon Oct 01, 2007 7:53 am Post subject: |
[quote] |
|
I've been using Lua and C++ in H-World. I never did measurements, Lua seemed "fast enough". That is, performance bottlenecks were elsewhere, not the Lua scripts, so it didn't seem neccesary. I've been scripting items data, and the "usages" of items, so part of the functionality of the game.
I could imagine you'll want the networking code in C++, since AFAIK Lua has no suitable libraries for that. Also networking is a fairly low level thing often, handling bytes and bits which scripting languages aren't really good for. They excel at the high level stuff.
I ended up moving more and more C++ code to Lua scripts, though. So I can imagine one only wants a small server core in C++ and most game logic in Lua. I think that'd work.
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Mon Oct 01, 2007 3:33 pm Post subject: |
[quote] |
|
I found a tutorial on AngelScript, and went through that to see what it was like (they made it based on some game, so I thought it would do me some good).
In order for C++ to know about the functions/variables that AngelScript has to offer, it has to "register" them within the engine code. Also, in order for AngelScript to know about variables from c++, it has to register them as well.
The downside to this is that you just can't add code on the fly:
Code: | Angelscript->LoadScript()
LoadScript data:
class Player
{
int health;
int equippedItem;
D3DXVECTOR position;
};
|
Basically here, the Player class is within the LoadScript() function, so to use it you must load the script. However, if C++ has to know about the class and all it's variables (or does it?), how are you supposed to modify the game?
I realize you could have a general idea of what you would load (say you have a variable for the number of enemies), and the script could specify that number, but what if you wanted to add something that c++ didn't already know about?
Does this make sense?
My main concern is if I create my Player Class with all it's attributes/stats, and want to add more later. Will I have to tell c++ what that new attribute is, or can I just add it to the script:
Code: | Player.addAttribute(attribute); |
C++ doesn't have a variable for this attribute, since it is new, and I want to add it to the code. Am I able to do this?
|
|
Back to top |
|
 |
Hajo Demon Hunter
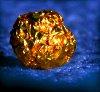
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Mon Oct 01, 2007 7:27 pm Post subject: |
[quote] |
|
In H-World I kept all attributes of items and mobs in hashtables and looked them up by name. This was fairly easy to interlink between C++ and Lua, and although way slower than direct variable lookups, it still fast enough.
This approach needs big care though, since a mistyped attribute name will not be reported by the compiler nor interpreter upon a hashtable lookup, unless you define constants for them all and only use the constants in your code.
I had the vision to have the core engine expandable with new stats and attributes for all kinds of player defined modules. It worked, but then on hindsight, I think I overdid it.
In Lua this looked like:
Code: |
local blind = thing_get_value(user, "effect.blind") or 0
if blind > 0 then
thing_set_value(user, "effect.blind", blind-1);
if(blind < 2 and thing_get_value(user, "is_player")) then
translate("You can see again.")
end
end
|
In C++ it'd look like this:
Code: |
thinghandle_t &ti = things[pos.x + pos.y*size.x];
// must be stackable, and less than max stack
const int max = ti->get_int("max_stack", 1);
const int cur = ti->get_int("cur_stack", 1);
|
So yes, you can do this. But you need more dynamic data structures for this.
|
|
Back to top |
|
 |
Gardon Scholar
Joined: 05 Feb 2006 Posts: 157
|
Posted: Mon Oct 01, 2007 8:04 pm Post subject: |
[quote] |
|
Awesome, thanks. What I think what I'm going to do is implement everything through hard-code methods to begin with. I won't be testing the functionality (ie. how well the player's hitpoints measure up to attack damage, etc.) just quite yet, so if I can get the basics down then I might be better off.
I find myself worrying about scripting too much, to the extent where I'm overplanning everything and not getting anything done.
I'll come back to this post in a few months when I'm ready to get the flexibility built.
Thank you Hajo and Ninkazu for both your help.
|
|
Back to top |
|
 |
Hajo Demon Hunter
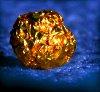
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Mon Oct 01, 2007 8:15 pm Post subject: |
[quote] |
|
Good luck :)
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|