View previous topic - View next topic |
Author |
Message |
DeveloperX 202192397
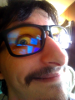
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Thu Feb 28, 2008 3:50 am Post subject: *HELP* Linked List like class in VBScript |
[quote] |
|
Firstly I apologize for the somewhat large code post.
If its a problem, I can upload it and link to it.
For some reason this code keeps failing to run, leaving wscript.exe running, I cannot seem to track down the problem.
Maybe someone else has a clue.
Code: |
''
'' DynamicArray class
''
Class DynamicArray
Private aData
Private Sub Class_Initialize()
ReDim aData(0)
End Sub
Public Property Get Data(iPos)
If iPos < LBound(aData) or iPos > UBound(aData) Then
Exit Property
End If
Data = aData(iPos)
End Property
Public Property Get DataArray()
DataArray = aData
End Property
Public Property Let Data(iPos, varValue)
If iPos < LBound(aData) Then Exit Property
If iPos > UBound(aData) Then
ReDim Preserve aData(iPos)
aData(iPos) = varValue
Else
aData(iPos) = varValue
End If
End Property
Public Function StartIndex()
StartIndex = LBound(aData)
End Function
Public Function StopIndex()
StopIndex = UBound(aData)
End Function
Public Sub Delete(iPos)
If iPos < LBound(aData) Or iPos > UBound(aData) Then
Exit Sub
End If
Dim iLoop
For iLoop = iPos TO UBound(aData) - 1
aData(iLoop) = aData(iLoop + 1)
Next
ReDim Preserve aData(UBound(aData) - 1)
End Sub
End Class
Class WeakListEx
Private dynArray
Private Sub Class_Initialize()
Set dynArray = New DynamicArray
End Sub
Private Sub Class_Terminate()
Set dynArray = Nothing
End Sub
Public Property Get Count()
Count = dynArray.StopIndex() - dynArray.StartIndex()
End Property
Public Sub AddHead(varItem)
Dim iLoop
For iLoop = dynArray.StopIndex() To dynArray.StartIndex() STEP -1
dynArray.Data(iLoop + 1) = dynArray.Data(iLoop)
Next
dynArray.Data(dynArray.StartIndex()) = varItem
End Sub
Public Function RemoveHead()
If dynArray.StopIndex() > dynArray.StartIndex() Then
RemoveHead = dynArray.Data(dynArray.StartIndex())
dynArray.Delete(dynArray.StartIndex())
End If
End Function
Public Function RemoveElement(elementIndex)
If dynArray.StopIndex() > dynArray.StartIndex() Then
If elementIndex > dynArray.StartIndex() And elementIndex < dynArray.StopIndex() Then
RemoveElement = dynArray.Data(elementIndex)
dynArray.Delete(elementIndex)
End If
End If
End Function
Public Sub AddTail(varItem)
dynArray.Data(dynArray.StopIndex()) = varItem
End Sub
Public Function RemoveTail()
If dynArray.StopIndex() > dynArray.StartIndex() Then
RemoveTail = dynArray.Data(dynArray.StopIndex() - 1)
dynArray.Delete(dynArray.StopIndex() - 1)
End If
End Function
Public Function PeekHead()
If dynArray.StopIndex() > dynArray.StartIndex() Then
PeekHead = dynArray.Data(dynArray.StartIndex())
End If
End Function
Public Function PeekTail()
If dynArray.StopIndex() > dynArray.StartIndex() Then
PeekTail = dynArray.Data(dynArray.StopIndex() - 1)
End If
End Function
Public Function ElementAt(elementIndex)
If dynArray.StopIndex() > dynArray.StartIndex() Then
If elementIndex >= dynArray.StartIndex() And elementIndex <= dynArray.StopIndex() Then
ElementAt = dynArray.Data(elementIndex )
End If
End If
End Function
End Class
Class StackEx
Private wlList
Private Sub Class_Initialize()
Set wlList = New WeakListEx
End Sub
Private Sub Class_Terminate()
Set wlList = Nothing
End Sub
Public Property Get Count()
Count = wlList.Count
End Property
Public Function Push(varItem)
wlList.AddHead(varItem)
End Function
Public Function Pop()
Pop = wlList.RemoveHead()
End Function
Public Function Peek()
Peek = wlList.PeekHead()
End Function
Public Function At(elementIndex)
At = wlList.ElementAt(wlList.Count - (1 + elementIndex))
End Function
Public Function Remove(elementIndex)
Remove = wlList.RemoveElement(wlList.Count - (1 + elementIndex))
End Function
End Class
Dim Items(10)
Dim LastItem
Items(0) = "hammer"
Items(1) = "hammer"
Items(2) = "saw"
Items(3) = "nail"
Items(4) = "nail"
Items(5) = "screwdriver"
LastItem = 5
Sub DisplayInventory()
Dim display
Dim target
Dim quantity
Dim tmpItems
Set tmpItems = New StackEx
For I = 0 To LastItem
tmpItems.Push(Items(I))
Next
WScript.Echo("entering while loop")
WScript.Echo("There are " & tmpItems.Count & " items")
display = "You are carrying:" & vbcrlf
While tmpItems.Count > 0
target = tmpItems.Peek()
quantity = 0
For I = 0 TO tmpItems.Count
If tmpItems.At(I) = target Then
tmpItems.Remove(I)
quantity = quantity + 1
I = I - 1
End If
Next
display = display & target & " x" & LTrim(CStr(quantity)) & vbcrlf
WScript.Echo(display)
Wend
Set tmpItems = Nothing
MsgBox display, 0, "Inventory"
End Sub
DisplayInventory
|
Any ideas? Better implementation? Suggestions besides using another language? _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Mattias Gustavsson Mage

Joined: 10 Nov 2007 Posts: 457 Location: Royal Leamington Spa, UK
|
|
Back to top |
|
 |
DeveloperX 202192397
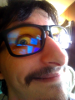
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Thu Feb 28, 2008 11:45 am Post subject: |
[quote] |
|
Mattias Gustavsson wrote: | Any way you could use the Collection object instead? Would make a lot of sense for an inventory... |
There is no Collection object in VBScript or else I would have already done that.
I'm scaling my design farther back, I'd rather be able to make a small game than an incomplete tech demo.
I've redesigned how to handle the items, maybe it will help.
Who knows. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Mattias Gustavsson Mage

Joined: 10 Nov 2007 Posts: 457 Location: Royal Leamington Spa, UK
|
Posted: Thu Feb 28, 2008 3:16 pm Post subject: |
[quote] |
|
You sure? I've used collections with VBScript both when running through windows script host and on ASP... What are you running your VBScripts in? _________________ www.mattiasgustavsson.com - My blog
www.rivtind.com - My Fantasy world and isometric RPG engine
www.pixieuniversity.com - Software 2D Game Engine
|
|
Back to top |
|
 |
DeveloperX 202192397
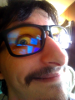
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Thu Feb 28, 2008 6:27 pm Post subject: |
[quote] |
|
Mattias Gustavsson wrote: | You sure? I've used collections with VBScript both when running through windows script host and on ASP... What are you running your VBScripts in? |
...do tell how you got collections working under WSH. because the vbscript chm docs explicitly state that collections are not supported. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |