View previous topic - View next topic |
Author |
Message |
JimKurth Monkey-Butler

Joined: 01 Apr 2007 Posts: 53 Location: Houston, TX
|
Posted: Tue Sep 02, 2008 12:37 am Post subject: XNA 2.0 RPG programming |
[quote] |
|
It's been awhile since I've been on here. I've been going away from QB and more into XNA 2.0. And have some RPG design questions. Here's what I have so far:
Everything is set up in a layer format as follows (in order):
1) Screen engine (title screen, blank screen with text, etc)
2) Map/Town engine (world, towns, buildings)
3) Combat engine (bosses and enemies)
4) Window engine (dialog boxes, menu boxes, etc)
And for each engine I add boolean flags to start/stop certain functions (if WindowEngine's DialogBox = true then show dialog box with it's text, etc).
In this bare model, each engine has a Render and an Input flag. If the Input flag is on (which is checked in Update()) then it'll perform any logic related to it. If the Render flag is on (checked in Draw()) then it'll draw in order based on the flags for that engine in order that is desired.
I also have a Keyboard Parser to return codes based on what keys were pressed (up,down,left,right,[OK],[CANCEL],[MENU]).
Now here is where I run into problems and need help:
1) How does an RPG script engine work to say:
* if player is in this town, at x/y and hits OK, or talks to this NPC, then move forward into the story.
* if player goes to this town and his first time, then run cutscene 14a.
2) I'm having trouble writing a GetKey() function to only get the OK button (like when you're paused). My concept is to set a flag (bool Need_an_OK;) and in the game's Update function, check for the OK button after getting KeyboardState. If it's not pressed then goto the end of the Update function.
Only problem is that no other logic will be performed (no other movements by NPCs or anything).
Any ideas? Thanks! _________________ Being a non-commission officer isn't easy. It it were, they would have given it to the officer corps.
|
|
Back to top |
|
 |
Nodtveidt Demon Hunter
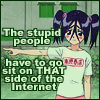
Joined: 11 Nov 2002 Posts: 786 Location: Camuy, PR
|
Posted: Tue Sep 02, 2008 2:15 pm Post subject: |
[quote] |
|
You could use what is sometimes referred to as a "switch list". Basically, this is a bool array that turns on and off event parameters. For example:
Code: | if (!switchlist[124]) run_cutscene(14a, 124); |
Of course, this would be straight-up code and I'm sure everyone and their grandmother's going to be pimping the "benefits" of 100% scripting RPG design, but this is just to illustrate the idea. In the cutscene call above, it passes the ID of the cutscene (could be a #define earlier in the code) and the number of the switch to affect. The function would be designed to run the cutscene and then change the value of switchlist[124] to True, therefore skipping it the next time. _________________ If you play a Microsoft CD backwards you can hear demonic voices. The scary part is that if you play it forwards it installs Windows. - wallace
|
|
Back to top |
|
 |
dbest Milk Maid
Joined: 02 May 2007 Posts: 41
|
Posted: Thu Sep 11, 2008 10:26 am Post subject: |
[quote] |
|
I am just coding a vertical scroller game in XNA and i think its kinda cool and makes life a bit easier.
Will work on some tile engine tutorials later on, and see if i can come up with something nice.
Jim, do keep us informed about the progress of your game.
|
|
Back to top |
|
 |
Terry Spectral Form
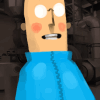
Joined: 16 Jun 2002 Posts: 798 Location: Dublin, Ireland
|
|
Back to top |
|
 |
Hajo Demon Hunter
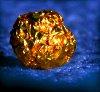
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Thu Sep 11, 2008 2:51 pm Post subject: |
[quote] |
|
A big german computer magazine recently had a fairly big article about game development with XNA. They praised the ease to make games with XNA, unfortunately there wasn't much to read about the troubles that become visible in the linked threads.
I'm just telling, since I assume the article caught much interest, the magazine has a good name here.
|
|
Back to top |
|
 |
JimKurth Monkey-Butler

Joined: 01 Apr 2007 Posts: 53 Location: Houston, TX
|
Posted: Fri Sep 12, 2008 6:20 am Post subject: Hey |
[quote] |
|
I didn't know how bad XNA games didn't work on other PCs or that you need C#/XNA installed to run an XNA game. I thought the compiler or publisher took care of that. Hmm..
well, either case since this is my first RPG, XNA really helps me understand how to code an RPG and to make things a little easier as well. QB was my first choice with ASM routines but that quickly got canned cuz my frame rates were horrible and resolutions were not all that great.
Anyway, I've found that instead of Input and Render flags to each engine, I'm using different gamestates and different substates for each engine (Screen, Map, Menu, Combat engines). Using a current state and previous state variable to log what the current gamestate and substate is and then act on that. Also using shared methods to perform certain actions (i.e. drawdialogbox(), drawtext(), changeStat(), etc) to be shared amongst them all. The idea is to make my game just do what it's told to do and be able to perform any task it's given. After that is complete, then I will write a sample script string for it and develop an engine to parse it and then write a script-file reading engine before I write script codes.
So far, I have my character animated as he moves around the screen and is bound by the display edges. Game will be 800x600 but utilize a mix between 400x300 scaled graphics and 800x600. I'm mostly focused on prewriting code on paper to see if my game/substate-concept will work or not with what I have and what my needs are. _________________ Being a non-commission officer isn't easy. It it were, they would have given it to the officer corps.
|
|
Back to top |
|
 |
JimKurth Monkey-Butler

Joined: 01 Apr 2007 Posts: 53 Location: Houston, TX
|
Posted: Sat Sep 13, 2008 12:20 am Post subject: update |
[quote] |
|
Well, I've put it down on paper and here is how it works. I do not have a stack management system but I have a current and old state and substate for each engine. This way if I'm walking in a world and then move onto a location to load a town, I can change the substate of the map engine to a town and then when i leave the town, it will pull the previous substate (world) which will load up and place me right back where i originally was. I feel the name for a complete management system is not necessary for my game since I only use 4 engines (a screen engine, map engine, menu engine, and combat engine) which go in that order.
I've decided to write a sequencer buffer array to hold the script commands and it will read my script files and the process to store it in the sequencer is as follows:
* it will open up necessary script file (1 per city or goal)
* anything to store in the buffer all at once will be surrounded with script commands: REGION and ENDREGION
* each update of the game loop will parse all of these commands inside the REGION tags
* script commands will include an IF and ENDIF to perform conditional statements returning true or false
* Each region has a name and whenever a house is to be entered, it will look for it's name to verify that is the one to load into the sequencer.
now I have to go use the bathroom. It's been fun.
If anyone has tried this and failed getting it to work right, please let me know so I don't go off track. Thanks.
-Jim _________________ Being a non-commission officer isn't easy. It it were, they would have given it to the officer corps.
|
|
Back to top |
|
 |
Terry Spectral Form
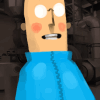
Joined: 16 Jun 2002 Posts: 798 Location: Dublin, Ireland
|
Posted: Sat Sep 13, 2008 2:13 am Post subject: |
[quote] |
|
Grand so! Just don't say I didn't warn you when nobody but you can get your game working... _________________ http://www.distractionware.com
|
|
Back to top |
|
 |
JimKurth Monkey-Butler

Joined: 01 Apr 2007 Posts: 53 Location: Houston, TX
|
Posted: Sat Oct 11, 2008 8:00 pm Post subject: question |
[quote] |
|
Terry wrote: | Grand so! Just don't say I didn't warn you when nobody but you can get your game working... |
My project uses textures and simple sprite placement routines. No real 2D video card special effects (pixel shaders or anything other than animated sprites). I use basic routines to handle input/output and gfx placement and simple logic using X/Y system.
QUESTION: Though people have had trouble getting XNA games to work properly on their computer, does this pose a threat to my project despite it's traditional programming style? I understand there are certain requirements for anyone to play XNA games outlined in the MS Documentation for XNA publishing (.NET 2.0, and some others).
|
|
Back to top |
|
 |
Verious Mage

Joined: 06 Jan 2004 Posts: 409 Location: Online
|
Posted: Sun Oct 12, 2008 2:28 pm Post subject: |
[quote] |
|
I have never had a problem getting XNA games to run on any computer and I've run them on several computers with different configurations.
I think it depends largely on how the installer is configured (and whether or not the dependencies are setup properly).
|
|
Back to top |
|
 |
valderman Mage
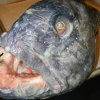
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Sun Oct 12, 2008 7:59 pm Post subject: |
[quote] |
|
Verious wrote: | I have never had a problem getting XNA games to run on any computer and I've run them on several computers with different configurations.
I think it depends largely on how the installer is configured (and whether or not the dependencies are setup properly). | However, you're leaving Mac and Linux users out in the cold. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
dbest Milk Maid
Joined: 02 May 2007 Posts: 41
|
Posted: Mon Oct 13, 2008 7:44 am Post subject: |
[quote] |
|
Again this is a huge topic out there regarding the cross platform compatibility.
For MS, cross platform means Windows, XBOX and now Zune. The other systems (Linux, Mac) are ruled out.
Inspite of that, I think XNA is still cool to program in. It eases the task of development.
With the release of XNA 3.0, the deployment of XNA based games will also be simpler.
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Mon Oct 13, 2008 10:30 am Post subject: |
[quote] |
|
Quote: | However, you're leaving Mac and Linux users out in the cold. |
Not just Mac and Linux, people with older video cards (and older Windows operating systems). XNA refuses to run on systems with most pre-DX9 video cards due to lack of pixel shader 1.1, regardless of whether you use it or not. I guess this isn't an issue since most people other than me will have a decent video card in their PC. My card is capable of running Quake 3, but it looks like I will need to upgrade my video card in order to run your 2D tile based game in XNA.
|
|
Back to top |
|
 |
Hajo Demon Hunter
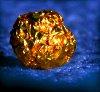
Joined: 30 Sep 2003 Posts: 779 Location: Between chair and keyboard.
|
Posted: Mon Oct 13, 2008 10:33 am Post subject: |
[quote] |
|
Friends just called my equipment "ancient". So you are not alone, there are more with old equipment and little will/little options to upgrade.
|
|
Back to top |
|
 |
valderman Mage
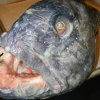
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Tue Oct 14, 2008 12:10 pm Post subject: |
[quote] |
|
RedSlash wrote: | Quote: | However, you're leaving Mac and Linux users out in the cold. |
Not just Mac and Linux, people with older video cards (and older Windows operating systems). XNA refuses to run on systems with most pre-DX9 video cards due to lack of pixel shader 1.1, regardless of whether you use it or not. I guess this isn't an issue since most people other than me will have a decent video card in their PC. My card is capable of running Quake 3, but it looks like I will need to upgrade my video card in order to run your 2D tile based game in XNA. | It runs on the Zune but not on a pre-2006 PC? Some great technology you have there. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |