View previous topic - View next topic |
Author |
Message |
Captain Vimes Grumble Teddy
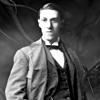
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Mon Oct 27, 2008 2:30 pm Post subject: Yet Another Question from the Captain |
[quote] |
|
As DevX, Hajo and RedSlash already know, I'm working on a game engine... and it's not going so well. I've got the basic skeleton of it coded, but there's a major problem that I'm completely unable to identify.
I'm using SDL - which I love - and that's made things a lot easier, but one of the things I've noticed is that my compiler doesn't return an error when I would be accessing a variable that hasn't been initialized or has a NULL value. It just closes the program when it's started running. That's what I thought this was at first.
See, I've created the basic GameEngine and Sprite class headers and definitions, and started a small project file to test them out. The bulk of the program is basically:
Code: | for(;;)
{
if(SDL_PollEvent(&event))
{
if(event.type == SDL_QUIT) break;
}
pEngine->Draw();
} |
Which should just put a blank window on the screen. It does - once. Then the program closes.
I went into the GameEngine::Draw() function code, which is this:
Code: | void GameEngine::Draw()
{
//First fill the background with black
SDL_FillRect(m_pScreen, NULL, 0);
//Now draw the background
DrawBgrnd();
//Now draw the sprites
DrawSprites();
//Update the screen
SDL_UpdateRect(m_pScreen, 0, 0, 0, 0);
SDL_Flip(m_pScreen);
} |
Each of the two sub-Draw() functions, DrawBgrnd() and DrawSprites(), have safeguards against accessing a NULL variable. But that's beside the point, because I tried commenting the calls to both those functions out, and the error still happened.
In fact, I commented out the entire function code, so it was just an empty function, but the program still crashed. Then I tried not calling Draw() at all, and it worked fine.
Any ideas as to why the action of simply calling GameEngine::Draw() crashes the program?[/code] _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
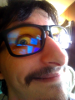
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Mon Oct 27, 2008 4:29 pm Post subject: |
[quote] |
|
Without seeing more code I cannot say exactly why its crashing;
however I can offer some help on debugging it.
In your function GameEngine::Draw, do this:
Code: |
void GameEngine::Draw()
{
fprintf(stderr, "\nGameEngine::Draw() - begin\n");
//First fill the background with black
SDL_FillRect(m_pScreen, NULL, 0);
fprintf(stderr, "\nGameEngine::Draw() - calling DrawBgrnd()\n");
//Now draw the background
DrawBgrnd();
fprintf(stderr, "\nGameEngine::Draw() - calling DrawSprites()\n");
//Now draw the sprites
DrawSprites();
//Update the screen
fprintf(stderr, "\nGameEngine::Draw() - updating m_pScreen\n");
SDL_UpdateRect(m_pScreen, 0, 0, 0, 0);
fprintf(stderr, "\nGameEngine::Draw() - flipping m_pScreen\n");
SDL_Flip(m_pScreen);
fprintf(stderr, "\nGameEngine::Draw() - end\n");
}
|
make sure to include the cstdio header
I use this at the top of almost all my sources
Code: |
#include <cstdlib>
#include <cstdio>
#include <cstring>
|
do the same sort of thing in your DrawBgrnd() and DrawSprites() functions, and run your program from a terminal/dos prompt.
Then post your resulting output "error log text" for me to have a look.
If you find that its not outputting all the lines that it should have, its easy to locate the error. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
valderman Mage
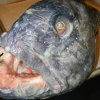
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Mon Oct 27, 2008 5:24 pm Post subject: |
[quote] |
|
This may sound stupid, but are you absolutely positive that pEngine is actually a valid pointer to a GameEngine object? _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Mon Oct 27, 2008 5:38 pm Post subject: |
[quote] |
|
Do you use Visual Studio express? It has an awesome debugger which lets you step through your program line by line and allows you to see all the values of your variables as you step through your program. If your program crashes under the debugger, it should jump to the line that it crashed on, and would provide you with a trace of functions called which led up to the crashing point.
If you use other IDEs, they should have a similar feature.
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
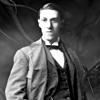
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Mon Oct 27, 2008 5:49 pm Post subject: |
[quote] |
|
Okeydokey... I'll answer everybody's questions.
@RedSlash: I use Bloodshed Dev-C++ 4.9.9.2, which I personally love and adore and would totally have its baby. Thanks for the recommendation, though.
@valderman: That was another thing that occurred to me, so I used DevX's proposed solution with the addition of it outputting an error message if pEngine was equal to NULL. There was no error message, so I guess it wasn't.
@DeveloperX: I did what you said, so here's the output...
stderr wrote: | Begin Hammer::Draw()
Filling screen with black
Drawing the background
Drawing the sprites
Fatal signal: Segmentation Fault (SDL Parachute Deployed) |
Any ideas now? I have no idea what that last bit means. Oh yeah, and the "Drawing the sprites" output bit is located the line before the DrawSprites() function is actually called. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
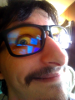
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Mon Oct 27, 2008 6:20 pm Post subject: |
[quote] |
|
Dev-C++ has a debugger too. Enable it by Projects->Project Options->Compiler->Linker->Generate debugging information. Then you can use the Debug menu to start debugging.
In any case, it looks like DrawSprite is the culprit as mentioned above. If you don't mind posting the source, we should be able to help you figure it out.
|
|
Back to top |
|
 |
DeveloperX 202192397
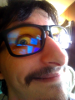
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Mon Oct 27, 2008 7:43 pm Post subject: |
[quote] |
|
RedSlash is right.
First, make sure you are using a project in Dev-C++, not just viewing a source file.
Then go to Project Options - Compiler - Linker and set Generate debugging information to "yes", and make sure you are not using any optimization options (they're not good for debug mode). Also check the Parameters tab, make sure you don't have any optimization options (like -O2 or -O3, but -O0 is ok because it means no optimization) or strip option (-s).
After that, do a full rebuild (Ctrl-F11), then set breakpoint(s) where you want the debugger to stop (otherwise it will just run the program). To set a breakpoint on a line, just click on the gutter (the gray band on the left), or press Ctrl-F5.
Now you are ready to launch the debugger, by pressing F8 or clicking the debug button. If everything goes well, the program will start, and then stop at the first breakpoint. Then you can step through the code, entering function calls, by pressing Shift-F7 or the "step into" button, or stepping over the function calls, by pressing F7 or the "next step" button. You can press Ctrl-F7 or the "continue" button to continue execution till the next breakpoint. At any time, you can add or remove breakpoints.
When the program stopped at a breakpoint and you are stepping through the code, you can display the values of various variables in your program by putting your mouse over them, or you can display variables and expressions by pressing F4 or the "add watch" button and typing the expression.
The help files included with Dev-C++ explain some things in more detail. You should read them. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
valderman Mage
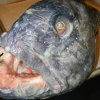
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Mon Oct 27, 2008 8:05 pm Post subject: |
[quote] |
|
Quote: | @valderman: That was another thing that occurred to me, so I used DevX's proposed solution with the addition of it outputting an error message if pEngine was equal to NULL. There was no error message, so I guess it wasn't. | A pointer may very well be invalid even though it's not NULL. Do you anywhere in your code initialise pEngine to anything, and if you do, are you sure that you don't modify it in weird ways after its initialisation?
There is no magic to make sure that a pointer to GameEngine actually points to a GameEngine object, that is up to you. There are several ways to mess this up, including (but not limited to) not initialising the pointer (then your pointer will point to random garbage,) doing arithmetic on it in improper ways or pointing it to something that is not an object of the proper type (GameEngine *p = 0x13371337;).
If this is indeed the case, you're very lucky that it just crashes your program; such errors often cause very bizarre effects long after the bug actually happened, which makes them nigh-undebuggable. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
valderman Mage
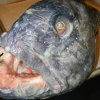
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Mon Oct 27, 2008 8:09 pm Post subject: |
[quote] |
|
RedSlash wrote: | In any case, it looks like DrawSprite is the culprit as mentioned above. If you don't mind posting the source, we should be able to help you figure it out. | Doesn't seem likely, as his program crashes even when he commented out every single call. That looks more like the result of doing ((GameEngine *) RANDOM_GARBAGE)->Draw(); to me. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
DeveloperX 202192397
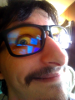
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
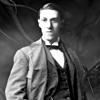
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Tue Oct 28, 2008 6:32 pm Post subject: |
[quote] |
|
Here's the source...
WARNING: MASSIVE WALL OF TEXT APPROACHING
Seriously, unless you really, really want to read through multiple pages of code you might as well leave the thread now.
Code: | //Start Sprite.h
#include <cstdio>
#include <cstdlib>
#include <SDL>
#include <SDL>
using namespace std;
//Sprite class definition
class Sprite
{
private:
//Private variable declarations; these can be used only by the Sprite
int m_xVel, m_yVel; //X and Y velocities
bool m_bVisible; //Whether or not to draw the sprite
SDL_Surface* m_pBmp; //The bitmap that will represent the Sprite
SDL_Surface* m_pScreen;
SDL_Rect m_rcSrc, m_rcDst; //The source and destination rectangles
Sprite* m_pNext; //The next sprite in a linked list
public:
//The constructor and destructor functions. There are several constructors
// provided, depending on how much of the Sprite must be defined during
// its creation.
Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen);
Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen, int x, int y);
Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen, int x, int y, bool visible);
Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen, int x, int y, bool visible, int w, int h);
Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen, int x, int y, bool visible, int w, int h,
int xVel, int yVel);
~Sprite();
//The class accessor functions. These are used to retrieve the values for
// the class's private variables from outside the class so that the program
// knows what's happening inside the class. Essentially, the program "peeks
// inside" the Sprite, but doesn't change anything.
int GetX() { return m_rcDst.x; };
int GetY() { return m_rcDst.y; };
int GetXVel() { return m_xVel; };
int GetYVel() { return m_yVel; };
SDL_Surface* GetBMP() { return m_pBmp; };
SDL_Surface* GetScreen() { return m_pScreen; };
int GetW() { return m_rcDst.w; };
int GetH() { return m_rcDst.h; };
bool GetVisible() { return m_bVisible; };
SDL_Rect GetSrc() { return m_rcSrc; };
SDL_Rect GetDst() { return m_rcDst; };
Sprite* GetNext() { return m_pNext; };
//The class manipulator functions. These change the class variable values.
void SetX(int x) { m_rcDst.x = x; };
void SetY(int y) { m_rcDst.y = y; };
void SetXVel(int x) { m_xVel = x; };
void SetYVel(int y) { m_yVel = y; };
void SetBMP(SDL_Surface* pBmp) { m_pBmp = pBmp; };
void SetScreen(SDL_Surface* pScreen) { m_pScreen = pScreen; };
void SetW(int w) { m_rcDst.w = w; };
void SetH(int h) { m_rcDst.h = h; };
void SetVisible(bool visible) { m_bVisible = visible; };
void SetSrc(SDL_Rect rcSrc) { m_rcSrc = rcSrc; };
void SetDst(SDL_Rect rcDst) { m_rcDst = rcDst; };
void SetNext(Sprite* pNext) { m_pNext = pNext; };
//The rest of the class functions - drawing, moving, stuff like that.
void Draw();
void ChangePos();
};
//This constructor creates a Sprite with x and y values at (0, 0) and no special
// properties - no velocities or anything.
Sprite::Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen)
{
m_pBmp = pBmp;
m_xVel = m_yVel = 0;
m_rcSrc.x = m_rcSrc.y = 0;
m_rcSrc.w = m_pBmp->w;
m_rcSrc.h = m_pBmp->h;
m_rcDst.x = 0;
m_rcDst.y = 0;
m_bVisible = true;
m_pScreen = pScreen;
SDL_SetColorKey(m_pBmp, SDL_SRCCOLORKEY, SDL_MapRGB(m_pBmp->format, 255, 0, 255));
}
//This constructor creates a sprite at the specified x, y coordinates
Sprite::Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen, int x, int y)
{
m_pBmp = pBmp;
m_rcSrc.x = m_rcSrc.y = 0;
m_xVel = m_yVel = 0;
m_rcSrc.w = m_pBmp->w;
m_rcSrc.h = m_pBmp->h;
m_rcDst.x = x;
m_rcDst.y = y;
m_bVisible = true;
m_pScreen = pScreen;
SDL_SetColorKey(m_pBmp, SDL_SRCCOLORKEY, SDL_MapRGB(m_pBmp->format, 255, 0, 255));
}
//This constructor creates a sprite at the specified x, y coordinates, but also
// allows the specification of whether or not the sprite is visible
Sprite::Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen, int x, int y, bool visible)
{
m_pBmp = pBmp;
m_rcSrc.x = m_rcSrc.y = 0;
m_xVel = m_yVel = 0;
m_rcSrc.w = m_pBmp->w;
m_rcSrc.h = m_pBmp->h;
m_rcDst.x = x;
m_rcDst.y = y;
m_bVisible = visible;
m_pScreen = pScreen;
SDL_SetColorKey(m_pBmp, SDL_SRCCOLORKEY, SDL_MapRGB(m_pBmp->format, 255, 0, 255));
}
//This is the same as the previous, but also allows the creation of a sprite
// with a custom size
Sprite::Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen, int x, int y, bool visible, int w, int h)
{
m_pBmp = pBmp;
m_rcSrc.x = m_rcSrc.y = 0;
m_xVel = m_yVel = 0;
m_rcSrc.w = w;
m_rcSrc.h = h;
m_rcDst.x = x;
m_rcDst.y = y;
m_pScreen = pScreen;
m_bVisible = visible;
SDL_SetColorKey(m_pBmp, SDL_SRCCOLORKEY, SDL_MapRGB(m_pBmp->format, 255, 0, 255));
}
//Same as above, but also with starting x and y velocities
Sprite::Sprite(SDL_Surface* pBmp, SDL_Surface* pScreen, int x, int y, bool visible, int w, int h,
int xVel, int yVel)
{
m_pBmp = pBmp;
m_rcSrc.x = m_rcSrc.y = 0;
m_xVel = xVel;
m_yVel = yVel;
m_rcSrc.w = w;
m_rcSrc.h = h;
m_rcDst.x = x;
m_rcDst.y = y;
m_bVisible = visible;
m_pScreen = pScreen;
SDL_SetColorKey(m_pBmp, SDL_SRCCOLORKEY, SDL_MapRGB(m_pBmp->format, 255, 0, 255));
}
//No special deconstruction, so this function is blank
Sprite::~Sprite() {}
//Blit the sprite to the specified surface - m_pScreen
void Sprite::Draw()
{
if(m_bVisible) SDL_BlitSurface(m_pBmp, NULL, m_pScreen, &m_rcDst);
}
//Update the sprite's position
void Sprite::ChangePos()
{
m_rcDst.x += m_xVel;
m_rcDst.y += m_yVel;
} |
Code: | //Game engine.h
#include <cstdio>
#include <cstdlib>
#include <iostream>
#include <time>
#include <SDL>
#include <SDL>
#include <SDL>
#include "Sprite.h"
using namespace std;
//GameEngine class definition ("Hammer" game engine)
class Hammer
{
private:
bool m_bFullscreen; //Whether or not the game is in fullscreen mode
bool m_bCursor; //Whether or not the cursor is visible
int m_iWidth, m_iHeight; //The window's width and height
Sprite* m_pFirst; //First Sprite in a linked list to draw the
// Sprites
SDL_Surface* m_pScreen; //A pointer to the screen
Sprite* m_pBgrnd; //The background of the current area
public:
//Constructor and destructor functions
Hammer(int iWidth, int iHeight);
Hammer(int iWidth, int iHeight, bool bFullscreen);
Hammer(int iWidth, int iHeight, bool bFullscreen, bool bCursor);
~Hammer();
//Accessor functions
bool GetFullscreen() { return m_bFullscreen; };
bool GetCursor() { return m_bCursor; };
int GetWidth() { return m_iWidth; };
int GetHeight() { return m_iHeight; };
Sprite* GetFirst() { return m_pFirst; };
SDL_Surface* GetScreen() { return m_pScreen; };
Sprite* GetBgrnd() { return m_pBgrnd; };
//Manipulator functions
void SetFullscreen(bool bFull) { m_bFullscreen = bFull; };
void SetCursor(bool bCursor) { m_bCursor = bCursor; };
void SetWidth(int iWidth) { m_iWidth = iWidth; };
void SetHeight(int iHeight) { m_iHeight = iHeight; };
void SetFirst(Sprite* pFirst) { m_pFirst = pFirst; };
void SetScreen(SDL_Surface* pScreen) { m_pScreen = pScreen; };
void SetBgrnd(Sprite* pBgrnd) { m_pBgrnd = pBgrnd; };
//Game functions
void Draw();
void DrawSprites();
void DrawBgrnd();
void AddSprite(Sprite* pAdd);
};
Hammer::Hammer(int iWidth, int iHeight)
{
m_bFullscreen = false;
m_bCursor = true;
m_iWidth = iWidth;
m_iHeight = iHeight;
m_pScreen = SDL_SetVideoMode(m_iWidth, m_iHeight, 0, SDL_HWSURFACE | SDL_DOUBLEBUF);
}
Hammer::Hammer(int iWidth, int iHeight, bool bFullscreen)
{
m_bFullscreen = bFullscreen;
m_bCursor = true;
m_iWidth = iWidth;
m_iHeight = iHeight;
if(!m_bFullscreen) m_pScreen = SDL_SetVideoMode(m_iWidth, m_iHeight, 0, SDL_HWSURFACE | SDL_DOUBLEBUF);
else m_pScreen = SDL_SetVideoMode(m_iWidth, m_iHeight, 0, SDL_HWSURFACE | SDL_DOUBLEBUF | SDL_FULLSCREEN);
}
Hammer::Hammer(int iWidth, int iHeight, bool bFullscreen, bool bCursor)
{
m_bFullscreen = bFullscreen;
m_bCursor = bCursor;
m_iWidth = iWidth;
m_iHeight = iHeight;
if(!m_bFullscreen) m_pScreen = SDL_SetVideoMode(m_iWidth, m_iHeight, 0, SDL_HWSURFACE | SDL_DOUBLEBUF);
else m_pScreen = SDL_SetVideoMode(m_iWidth, m_iHeight, 0, SDL_HWSURFACE | SDL_DOUBLEBUF | SDL_FULLSCREEN);
if(!bCursor) SDL_SetCursor(false);
}
Hammer::~Hammer()
{}
//This function adds a Sprite to the sprite list for drawing and manipulation
void Hammer::AddSprite(Sprite* pAdd)
{
//Use a while() loop to find the first Sprite in the list that does not have
// a successor
Sprite* pCur = NULL;
if(m_pFirst != NULL) pCur = m_pFirst;
else
{
m_pFirst = pAdd;
}
while(pCur->GetNext() != NULL)
{
pCur = pCur->GetNext();
}
//We have found the Sprite that has no successor; add the new Sprite onto
// the list as that Sprite's successor
pCur->SetNext(pAdd);
}
void Hammer::DrawBgrnd()
{
if(m_pBgrnd != NULL) m_pBgrnd->Draw();
}
void Hammer::DrawSprites()
{
//Loop through the Sprite list until we find a Sprite with no successor
Sprite* pCur;
if(m_pFirst != NULL) pCur = m_pFirst;
else pCur = NULL;
while(pCur != NULL)
{
pCur->Draw();
if(pCur->GetNext() != NULL)
{
pCur = pCur->GetNext();
}
}
}
void Hammer::Draw()
{
//Draw everything to the screen
//First fill the background with black
fprintf(stderr, "\nBegin Hammer::Draw()\n");
fprintf(stderr, "\nFilling screen with black\n");
SDL_FillRect(m_pScreen, NULL, 0);
//Now draw the background
fprintf(stderr, "\nDrawing the background\n");
DrawBgrnd();
//Now draw the sprites
fprintf(stderr, "\nDrawing the sprites\n");
DrawSprites();
//Update the screen
fprintf(stderr, "\nUpdating the screen\n");
SDL_UpdateRect(m_pScreen, 0, 0, 0, 0);
fprintf(stderr, "\nFlipping buffers\n");
SDL_Flip(m_pScreen);
} |
NOTE: Don't bother about the name here. Kind of a joke between me and my family.
Code: | //Main source
#include <cstdio>
#include <cstdlib>
#include <iostream>
#include <cstring>
#include <SDL>
#include <SDL>
#include "GameEngine.h"
using namespace std;
int main(int argc, char* argv[])
{
SDL_Init(SDL_INIT_VIDEO);
Hammer* pHammer = new Hammer(800, 600);
Sprite* pBgrnd = new Sprite(SDL_LoadBMP("bgrnd.bmp"), pHammer->GetScreen());
pHammer->SetBgrnd(pBgrnd);
Sprite* pSmile = new Sprite(SDL_LoadBMP("smiley.bmp"), pHammer->GetScreen(), 0, 0);
pHammer->AddSprite(pSmile);
SDL_Event event;
for(;;)
{
if(SDL_PollEvent(&event))
{
if(event.type == SDL_QUIT) break;
}
if(pHammer == NULL) fprintf(stderr, "Engine not correctly created\n");
pHammer->Draw();
}
SDL_Quit();
return 0;
} |
I'll examine the DrawSprite() function in more detail later. I'm not exactly sure if that's the problem, but, like valderman said, if it's not then there's something wrong with the game engine itself. I'll check when I have more time.
Thanks to everybody that actually cares enough to read through that mess. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
RedSlash Mage
Joined: 12 May 2005 Posts: 331
|
Posted: Tue Oct 28, 2008 7:03 pm Post subject: |
[quote] |
|
I did not read through the code throughly as I am on a terminal, but in the line that says:
Sprite* pBgrnd = new Sprite(SDL_LoadBMP("bgrnd.bmp"),...
Did you check if the BMP was loaded properly?
Also, did you try the debugger? I wasn't joking when I said it would identify the line your program would've crashed on.
|
|
Back to top |
|
 |
DeveloperX 202192397
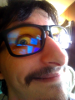
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
|
Back to top |
|
 |
DeveloperX 202192397
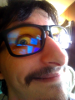
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Tue Oct 28, 2008 7:55 pm Post subject: |
[quote] |
|
ok.
I made your project so I could debug it.
And Presto.
Code: |
Starting program: /home/mpython/Projects/cpp/CaptainVimes_Game/demos/captain
[Thread debugging using libthread_db enabled]
[New Thread 0x7f6cd981d6f0 (LWP 6131)]
Program received signal SIGSEGV, Segmentation fault.
[Switching to Thread 0x7f6cd981d6f0 (LWP 6131)]
0x000000000040204e in Sprite::GetNext ()
|
There is your failure.
Sprite::GetNext () is failing.
The backtrace shows that its the AddSprite() function that is calling Getnext() when it fails.
Code: |
#0 0x000000000040204e in Sprite::GetNext ()
#1 0x0000000000400ebd in Hammer::AddSprite ()
#2 0x0000000000401eee in main ()
|
Another thing that bothers me, is your structure of your code.
If you would like, I can take a moment to restructure it so that things go smoothly.
Will not proceed without your permission. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
 |
Page 1 of 8 |
All times are GMT Goto page 1, 2, 3, 4, 5, 6, 7, 8 Next
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|