 |
|
View previous topic - View next topic |
Author |
Message |
DeveloperX 202192397
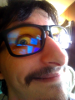
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Jun 06, 2009 1:02 am Post subject: Untitled - New Game Engine Project |
[quote] |
|
I am working on a new project to simplify making simple games.
This is a mock-up of what I'm thinking of.
I'd like to hear your ideas, and comments or suggestions.
The code below is supposed to create a base starting point for a defender-esque type game.
The player is always in the center of the screen on the X axis, and the background will scroll relative to the player's movements.
The player can move up and down freely.
Code: |
// main.cpp
// Project: Untitled - New Game Engine Project
// Author: Richard Marks <ccpsceo@gmail.com>
/*
The purpose of this file is to design a new game engine framework that
is easy to use and easy to extend by someone of even beginner skills in C++
*/
// make sure to set these defines before including the header
#define WINDOW_WIDTH 800
#define WINDOW_HEIGHT 600
#define WINDOW_BITS_PER_PIXEL 32
#define LOCK_FRAME_RATE TRUE
#define FRAMES_PER_SECOND 60
#define WINDOW_TITLE "Example of using the New Game Engine"
// include the header
#include <newgameengine.h>
bool game_initialize()
{
// allocate a new image resource
// the parameter is a prefix to the resource identifier name
// the actual identifier will be bg0 since we have not allocated any more resources
ResourcePtr background = new_image_resource("bg");
// try to load the resource
if (!background->load("data/graphics/background.png"))
{
log.write("unable to load resource: %s", background->resource_name());
return false;
}
// allocate a sprite resource
// the parameters are the resource prefix, the number of frames in the sprite, the width, the height
ResourcePtr ship = new_sprite_resource("ship", 2, 64, 24);
// try to load the resource
if (!ship->load("data/graphics/ship.png"))
{
log.write("unable to load resource: %s", ship->resource_name());
return false;
}
// add the resources to the engine so we can use them
engine.add_resource(background);
engine.add_resource(ship);
return true;
}
void game_main()
{
// create an image object
Image bg;
// init the object from the resource we added to the engine
bg.from_resource(engine.get_resource("bg", 0));
// create a sprite object
Sprite player;
// init the object from the resource we added to the engine
player.from_resource(engine.get_resource("ship", 0));
// our player will always be in the center of the screen
int cx = engine.get_screen_width() / 2 - player.get_width() / 2;
// init the sprite data
player.set_frame(1);
player.set_position(cx, engine.get_screen_height() - (player.get_height() * 4));
// while the engine is running
while(engine.is_running())
{
if (engine.key(KEY_UP))
{
player.move(0, -1, 4); // move on the Y axis 4 pixels per frame
}
if (engine.key(KEY_DOWN))
{
player.move(0, 1, 4); // move on the Y axis 4 pixels per frame
}
if (engine.key(KEY_LEFT))
{
player.set_frame(0);
player.move(-1, 0, 4); // move on the Y axis 4 pixels per frame
}
if (engine.key(KEY_RIGHT))
{
player.set_frame(1);
player.move(1, 0, 4); // move on the Y axis 4 pixels per frame
}
// start the frame (clears the screen to black)
engine.start_frame();
// draw our background image using the parallax renderer
// draw_parallax(x, y, which direction to scroll, pixels per frame to move)
bg.draw_parallax(player.get_x(), 0, ParallaxRight, 4);
// draw our player
player.draw(cx, player.get_y());
// end the frame (shows the actual screen buffer to the monitor)
engine.end_frame();
}
}
// start the main code
int main(int argc, char* argv[])
{
if (engine.initialize());
{
if (game_initialize())
{
engine.start(&game_main);
engine.shutdown();
}
else
{
log.write("game could not be initialized!");
}
}
else
{
log.write("engine could not be initialized!");
}
return 0;
}
|
please, comments and criticism are welcome. :D _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sat Jun 06, 2009 2:38 am Post subject: |
[quote] |
|
Looks promising.
I think scripting languages are ideal for this kind of thing. You need to put an obstacle system in also. And don't forget the projectile engine!
|
|
Back to top |
|
 |
DeveloperX 202192397
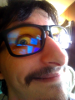
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Jun 06, 2009 3:19 am Post subject: |
[quote] |
|
obstacles and projectiles are implementation specific.
they are not a part of the engine itself.
some basic physics models I might implement, but anything major I'll leave for the user of the engine to create as they want.
scripting isn't really something that is needed for something like this. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Mattias Gustavsson Mage

Joined: 10 Nov 2007 Posts: 457 Location: Royal Leamington Spa, UK
|
Posted: Sat Jun 06, 2009 6:18 am Post subject: |
[quote] |
|
yeah, nothing wrong with that. But I think you're going about it the wrong way - make the game first, than refactor the reuseable bits into an engine or library. The result wil be much more useable, IME. _________________ www.mattiasgustavsson.com - My blog
www.rivtind.com - My Fantasy world and isometric RPG engine
www.pixieuniversity.com - Software 2D Game Engine
|
|
Back to top |
|
 |
DeveloperX 202192397
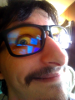
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Jun 06, 2009 10:13 am Post subject: |
[quote] |
|
I seem to find more enjoyment in designing the engine rather than implementing a full game. ..hence the zillion unfinished games on my computer. :P
Thought, as far as writing a game goes. This IS the way that I approach writing a game.
I write code that has no implementation that resembles the way I want my game to be written, then I implement it so that it works.
In fact I've done that for every single game that I've worked on.
design the code, write the code, implement the code, test the code...
its an interesting method that seems to work well for me.
in any event..its 5am now and I'm heading to bed..night all _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Mattias Gustavsson Mage

Joined: 10 Nov 2007 Posts: 457 Location: Royal Leamington Spa, UK
|
Posted: Sat Jun 06, 2009 11:50 am Post subject: |
[quote] |
|
This applies perfectly here: Write games not engines
So, make engines if that's what you like - but make them out of working game code, or it won't work...
DeveloperX wrote: | I seem to find more enjoyment in designing the engine rather than implementing a full game. |
You are aware that writing engine code is the easy bit, right? Making the game is what takes the real skill and effort...
But I guess, if you're just making it for your own enjoyment, with no real intention to use it for something, it's not a problem - doing something you enjoy always feels rewarding. But I think it'll be hard to get anyone to take an interest, or even notice... which is something you may or may not want to take into consideration. _________________ www.mattiasgustavsson.com - My blog
www.rivtind.com - My Fantasy world and isometric RPG engine
www.pixieuniversity.com - Software 2D Game Engine
|
|
Back to top |
|
 |
tcaudilllg Dragonmaster

Joined: 20 Jun 2002 Posts: 1731 Location: Cedar Bluff, VA
|
Posted: Sat Jun 06, 2009 1:27 pm Post subject: |
[quote] |
|
i think what he's saying is that he's going to make the obstacle and projectile algorithms as a seperate project.
|
|
Back to top |
|
 |
 |
Page 1 of 1 |
All times are GMT
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|