View previous topic - View next topic |
Author |
Message |
DeveloperX 202192397
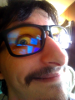
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Aug 22, 2009 7:20 am Post subject: |
[quote] |
|
I use SDL_TTF in my map editor.
One sec...
My init code is
Code: |
if (-1 == TTF_Init())
{
fprintf(stderr, "Unable to initialize SDL_TTF!\nSDL_TTF Error: %s\n", TTF_GetError());
return false;
}
editorfont = TTF_OpenFont("silkscreen.ttf", 8);
if (!editorfont)
{
fprintf(stderr, "Unable to load \"silkscreen.ttf\"!\nSDL_TTF Error: %s\n", TTF_GetError());
return false;
}
|
and here is a function that will make things easier
Code: |
void editor_printf(int x, int y, unsigned int color, const char* format, ...)
{
char textbuffer[0x100];
va_list args;
va_start(args, format);
vsprintf(textbuffer, format, args);
SDL_Color c;
Uint8 r, g, b;
SDL_GetRGB(color, editorscreen->format, &r, &g, &b);
c.r = r;
c.g = g;
c.b = b;
SDL_Surface* textsurface = TTF_RenderText_Solid(editorfont, textbuffer, c);
if (textsurface)
{
SDL_Rect dst;
dst.x = x;
dst.y = y;
SDL_BlitSurface(textsurface, 0, editorscreen, &dst);
SDL_FreeSurface(textsurface);
}
va_end(args);
}
|
here is an example of how to use it
Code: |
editor_printf(
editortilewidth + 4,
editortileheight - 14,
editortextcolor,
"%s | Mouse Tile X %3d/%3d | Mouse Tile Y %3d/%3d | Camera X %3d | Camera Y %3d | "
"Camera Width %2d | Camera Height %2d | Tile 0x%04X",
(EditorBGEdit==editormode)?"Background Edit Mode":
(EditorFGEdit==editormode)?"Foreground Edit Mode":"Collision Edit Mode",
editormousetilex, editorlevel->width,
editormousetiley, editorlevel->height,
editorcamera->intiles->x, editorcamera->intiles->y,
editorcamera->intiles->w, editorcamera->intiles->h, editorcurrentile);
|
if you have any questions, feel free to ask me. :)
oh..
the variables are
Code: |
TTF_Font* editorfont;
unsigned int editortextcolor =SDL_MapRGB(editorscreen->format, 255, 255, 255);
|
if you need anything, let me know _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Nodtveidt Demon Hunter
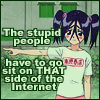
Joined: 11 Nov 2002 Posts: 786 Location: Camuy, PR
|
Posted: Sat Aug 22, 2009 10:58 am Post subject: |
[quote] |
|
I had some trouble with SDL_TTF at first as well...there really is a severe shortage of proper tutorials for it. But once you get it it's pretty easy...it simply renders text starting at the top left of the surface you're rendering to, then you simply blit that surface onto your screen surface or wherever you want it.
Rich, that's a pretty interesting function. A couple of things I wanna know though...
-Any particular reason you use -1 == TTF_Init()? Is this an optimization technique? I've seen such things before but wondered if this is one of them.
-Also, why do you create and destroy the surface in the function? I would figure this is additional overheard that you don't need; I just create the text render surface as a global and destroy it on exit. _________________ If you play a Microsoft CD backwards you can hear demonic voices. The scary part is that if you play it forwards it installs Windows. - wallace
|
|
Back to top |
|
 |
DeveloperX 202192397
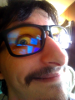
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sat Aug 22, 2009 9:07 pm Post subject: |
[quote] |
|
Nodtveidt wrote: |
Rich, that's a pretty interesting function. A couple of things I wanna know though...
-Any particular reason you use -1 == TTF_Init()? Is this an optimization technique? I've seen such things before but wondered if this is one of them.
-Also, why do you create and destroy the surface in the function? I would figure this is additional overheard that you don't need; I just create the text render surface as a global and destroy it on exit. |
It is because that is what determines if there was an error with initializing SDL_TTF.
See documentation:
3.1.2 TTF_Init
int TTF_Init()
Initialize the truetype font API.
This must be called before using other functions in this library, excepting TTF_WasInit.
SDL does not have to be initialized before this call.
Returns: 0 on success, -1 on any error
The overhead from the surface creation isn't that bad actually.
You could create a single "scratch pad" surface outside the main loop and clear it, and then write on it each time, however I find that SDL copes with creation/deletion of a surface faster than it takes to clear an existing surface.
One of the little things I came across while writing my map editor.
I got it from 2FPS to 40FPS by changing several things that I did.
That was one change that gave me about a 10FPS difference. _________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Nodtveidt Demon Hunter
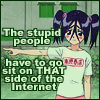
Joined: 11 Nov 2002 Posts: 786 Location: Camuy, PR
|
Posted: Sun Aug 23, 2009 2:44 am Post subject: |
[quote] |
|
DeveloperX wrote: | It is because that is what determines if there was an error with initializing SDL_TTF. |
That is not what I meant. I was referring to the order in which your comparison was done. You have the value to the left, and the function to the right. It is typically done the other way around. I wanted to know if you had a particular purpose for doing it the way you did it. _________________ If you play a Microsoft CD backwards you can hear demonic voices. The scary part is that if you play it forwards it installs Windows. - wallace
|
|
Back to top |
|
 |
DeveloperX 202192397
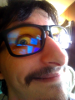
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
Posted: Sun Aug 23, 2009 3:20 am Post subject: |
[quote] |
|
Oh that!
Yes. In fact. A very good reason.
Its a very common and frustrating typo to use an assignment operator when you meant an equality comparison operator.
By using the value on the left hand side, you avoid every having to worry about frustrating bugs from that typo.
Because an assignment operator will compile if the left hand side contains a variable.
Code: |
int a = 5;
if (a = 5) { fprintf(stdout, "a is equal to five!\n"); } // logic bug
|
versus
Code: |
int a = 5;
if (5 = a) { fprintf(stdout, "a is equal to five!\n"); } // error
|
The latter is much better because then the compiler will point out your typo.
Code: |
int a = 5;
if (5 == a) { fprintf(stdout, "a is equal to five!\n"); } // correct
|
_________________ Principal Software Architect
Rambling Indie Games, LLC
See my professional portfolio
|
|
Back to top |
|
 |
Nodtveidt Demon Hunter
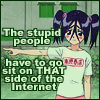
Joined: 11 Nov 2002 Posts: 786 Location: Camuy, PR
|
Posted: Mon Aug 24, 2009 12:12 am Post subject: |
[quote] |
|
Interesting...I can see the advantage, especially if switching back and forth between BASIC and C. _________________ If you play a Microsoft CD backwards you can hear demonic voices. The scary part is that if you play it forwards it installs Windows. - wallace
|
|
Back to top |
|
 |
DeveloperX 202192397
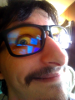
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
|
Back to top |
|
 |
Captain Vimes Grumble Teddy
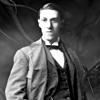
Joined: 12 May 2006 Posts: 225 Location: The City Streets
|
Posted: Wed Aug 26, 2009 3:39 pm Post subject: |
[quote] |
|
Thanks for the TTF help, guys. I haven't quite reached that point in the programming process yet, but I will soon. This will really help a lot. _________________ "Sometimes it is better to light a flamethrower than to curse the darkness."
- Terry Pratchett
|
|
Back to top |
|
 |
DeveloperX 202192397
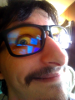
Joined: 04 May 2003 Posts: 1626 Location: Decatur, IL, USA
|
|
Back to top |
|
 |
 |
Page 2 of 2 |
All times are GMT Goto page Previous 1, 2
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|