View previous topic - View next topic |
Author |
Message |
XMark Guitar playin' black mage

Joined: 30 May 2002 Posts: 870 Location: New Westminster, BC, Canada
|
Posted: Thu Nov 06, 2003 8:16 am Post subject: |
[quote] |
|
heh, actually I'm going to stick with the ol' tile engine. I just had a bad day, got a little confused and fell into the old "start everything over again" trap.
Anyway, I figured out how to get it working in Dev-C++. Now I just have one problem. The map file loading thing doesn't seem to be working anymore. Here's my mapfile loading code (half of it, the other half goes through the map entities, loading them one by one and putting them in a vector)
Code: |
ifstream loadmap(filenamecopy, ios::in | ios::binary);
loadmap.read(mapname, 16);
loadmap.read(scriptfile, 16);
loadmap.read(tilefile, 16);
loadmap.read(backimg, 16);
loadmap.read(map1, 16384);
loadmap.read(map2, 16384);
loadmap.read(collision, 16384);
int tempsize;
loadmap.read(&tempsize, 4);
|
map1, map2, and collision are arrays of unsigned chars
and for those three I get this error message:
Code: | invalid conversion from 'unsigned char*' to 'char *' |
and for the loadmap.read(&tempsize, 4) I get this message
Code: | no matching function for call to `std::basic_ifstream<char, |
It's weird, it's like all of a sudden the read function can't handle anything except chars. _________________ Mark Hall
Abstract Productions
I PLAYS THE MUSIC THAT MAKES THE PEOPLES FALL DOWN!
|
|
Back to top |
|
 |
XMark Guitar playin' black mage

Joined: 30 May 2002 Posts: 870 Location: New Westminster, BC, Canada
|
Posted: Thu Nov 06, 2003 8:49 am Post subject: |
[quote] |
|
blarg. Seems 9 times out of 10, I figure out the answers to my questions about 15 minutes after posting them. All I had to do was type cast with some handy-dandy (char *)'s :) _________________ Mark Hall
Abstract Productions
I PLAYS THE MUSIC THAT MAKES THE PEOPLES FALL DOWN!
|
|
Back to top |
|
 |
valderman Mage
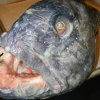
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Thu Nov 06, 2003 9:01 am Post subject: |
[quote] |
|
XMark wrote: | blarg. Seems 9 times out of 10, I figure out the answers to my questions about 15 minutes after posting them. All I had to do was type cast with some handy-dandy (char *)'s :) | It took you 15 minutes to realize that? o_O _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
Adam Mage
Joined: 30 Dec 2002 Posts: 416 Location: Australia
|
Posted: Thu Nov 06, 2003 9:02 am Post subject: |
[quote] |
|
pfft
and that was the first C++ question i had the answer to _________________ https://numbatlogic.com
|
|
Back to top |
|
 |
Mandrake elementry school minded asshole

Joined: 28 May 2002 Posts: 1341 Location: GNARR!
|
Posted: Thu Nov 06, 2003 2:28 pm Post subject: |
[quote] |
|
were you able to get Lua to compile? If not, I think the standard windows precompiled .dll windows pkg should work fine for 4.0, but 5.0 i think it's a source only release (i could be wrong).
If you still can't find them, maybe somebody here could send them to ya. _________________ "Well, last time I flicked on a lighter, I'm pretty sure I didn't create a black hole."-
Xmark
http://pauljessup.com
|
|
Back to top |
|
 |
syn9 Wandering Minstrel

Joined: 31 Aug 2002 Posts: 120 Location: USA
|
|
Back to top |
|
 |
XMark Guitar playin' black mage

Joined: 30 May 2002 Posts: 870 Location: New Westminster, BC, Canada
|
Posted: Fri Nov 07, 2003 11:44 pm Post subject: |
[quote] |
|
heh, actually now that my original source is working, I have my own homegrown scripting language that I'm using, so I don't need Lua anymore :) _________________ Mark Hall
Abstract Productions
I PLAYS THE MUSIC THAT MAKES THE PEOPLES FALL DOWN!
|
|
Back to top |
|
 |
XMark Guitar playin' black mage

Joined: 30 May 2002 Posts: 870 Location: New Westminster, BC, Canada
|
Posted: Sat Nov 08, 2003 8:02 am Post subject: |
[quote] |
|
Okay, I'm having another major problem right now. It's in my scripting engine. Specifically the problem is with adding variables to my scripting engine. What I have is an object called Scriptint which is held in a vector.
Code: |
class Scriptint
{
public:
Scriptint();
~Scriptint();
char name[40];
int value;
};
|
And here's the part of the scripting code which reads a line such as
int myvariable 32
and places the integer value into the variable vector
Code: |
case ST_NEWINT:
foundint = 0;
for (x = 0; x < scriptints.size(); x++) {
if (!strcmp(scriptints[x].name, curword))
foundint = 1;
}
strcpy (tempint.name, curword);
grabword(); // grabs the next word in the script into curword
tempint.value = atoi(curword);
if (!foundint)
scriptints.push_back(tempint);
state = ST_ACTIVE;
nodelay = 0;
break;
|
Now, the problem is with my scriptints vector. The program compiles perfectly with no errors or warnings, but when I run it, it crashes whenever I even touch the vector. By commenting and uncommenting code I narrowed it down and these following things cause the game to lock up/crash:
attempting to push_back into the vector
using either strcmp or strcpy on name
the FOR loop using scriptints.size()
although tempint.value = atoi(curword) seems to work fine for some reason. Any idea what I could possibly be doing wrong here? _________________ Mark Hall
Abstract Productions
I PLAYS THE MUSIC THAT MAKES THE PEOPLES FALL DOWN!
|
|
Back to top |
|
 |
Rainer Deyke Demon Hunter
Joined: 05 Jun 2002 Posts: 672
|
Posted: Sat Nov 08, 2003 5:31 pm Post subject: |
[quote] |
|
This may not have anything to do with your problem, but why do you use character arrays as strings? Using std::string is both easier and safer.
|
|
Back to top |
|
 |
valderman Mage
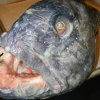
Joined: 29 Aug 2002 Posts: 334 Location: Gothenburg, Sweden
|
Posted: Sat Nov 08, 2003 5:58 pm Post subject: |
[quote] |
|
I would recommend against interpreting the scripts from raw text at runtime. Better to compile them into bytecode, either when the script is done, or at loadtime. _________________ http://www.weeaboo.se
|
|
Back to top |
|
 |
XMark Guitar playin' black mage

Joined: 30 May 2002 Posts: 870 Location: New Westminster, BC, Canada
|
Posted: Sat Nov 08, 2003 7:43 pm Post subject: |
[quote] |
|
well, the problem has nothing to do with interpreting the raw text and I'm not sure how to do the bytecode thing.
Just humor me here, guys, any idea what might be causing these crashes?
EDIT:
I just did a little test, and even when the name thing is completely removed and it only does anything with the value, I still get the crash. (illegal operation) It's like the program really doesn't want me to use vectors in my scripting code. Which is strange because all my other vectors in the code work perfectly. Just this one is screwing up. _________________ Mark Hall
Abstract Productions
I PLAYS THE MUSIC THAT MAKES THE PEOPLES FALL DOWN!
|
|
Back to top |
|
 |
DrunkenCoder Demon Hunter
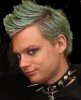
Joined: 29 May 2002 Posts: 559
|
Posted: Sat Nov 08, 2003 9:14 pm Post subject: |
[quote] |
|
hmm.. maybe you should be glad that it crashes so you have the option to use the correct data structure for that...
If your not going to compile it into something at least have a look at std::map instead. _________________ If there's life after death there is no death, if there's no death we never live. | ENTP
|
|
Back to top |
|
 |
Bjorn Demon Hunter

Joined: 29 May 2002 Posts: 1425 Location: Germany
|
Posted: Sun Nov 09, 2003 9:05 pm Post subject: |
[quote] |
|
I have a solution, you could try Lua. It's an extensible scripting language suitable for many kinds of application. If you use Lua, you won't have to write your own interpreter, it'll be much easier and you'll have dynamic typing, tables (that can behave like lists, arrays, hash tables, objects, modules, etc.), garbage collector, nice error messages for syntax and runtime errors, fast execution, and much more. ;-)
|
|
Back to top |
|
 |
XMark Guitar playin' black mage

Joined: 30 May 2002 Posts: 870 Location: New Westminster, BC, Canada
|
Posted: Sat Nov 29, 2003 2:32 am Post subject: |
[quote] |
|
Yes! I finally figured out what the problem was. It had nothing to do with the code, it was entirely a compiler problem. When I deleted all the .o files and compiled the whole program, everything worked really easy-like. I guess the compiler was probably putting the vector in an already-used section of memory or something. _________________ Mark Hall
Abstract Productions
I PLAYS THE MUSIC THAT MAKES THE PEOPLES FALL DOWN!
|
|
Back to top |
|
 |
LeoDraco Demon Hunter
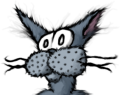
Joined: 24 Jun 2003 Posts: 584 Location: Riverside, South Cali
|
Posted: Sat Nov 29, 2003 3:33 am Post subject: |
[quote] |
|
Valderman wrote: | I would recommend against interpreting the scripts from raw text at runtime. Better to compile them into bytecode, either when the script is done, or at loadtime. |
That's complete and utter FUD bullshit. There is nothing wrong with run-time interpretation of raw text. _________________ "...LeoDraco is a pompus git..." -- Mandrake
|
|
Back to top |
|
 |